Question
Java Implementation - Needed Asap in an hour. import java.util.*; /** * A class representing a auto shop that has an owner . * A
Java Implementation - Needed Asap in an hour.
import java.util.*;
/**
* A class representing a auto shop that has an owner .
* A auto shop owns a collection (or possibly collections) of vehicles,
* but does not own the vehicles themselves . In other words,
* the auto shop and its collection of vehicles form a composition .
*
*
* Only the owner of the auto shop is able to sell
* vehicles
* from the auto shop. The auto shop does NOT own its owner .
* In other words, the auto shop and its owner form an
* aggregation .
*
*/
public class AutoShop {
/*
* YOU NEED A FIELD HERE TO HOLD THE Vehicle OF THIS AutoShop
*/
/**
* Initializes this auto shop so that it has the specified owner
* and no vehicles.
*
* @param owner
* the owner of this auto shop
*/
public AutoShop (ShopOwner owner) {
// COMPLETE THIS
}
/**
* Initializes this auto shop by copying another auto shop. This auto shop
* will have the same owner and the same number and type of vehicles as
* the other auto shop.
*
* @param other
* the auto shop to copy
*/
public AutoShop(AutoShop other) {
// COMPLETE THIS
}
/**
* Returns the owner of this auto shop.
*
*
* This method is present only for testing purposes. Returning
* the owner of this auto shop allows any user to sell vehicles
* from the auto shop (because any user can get the owner
* of this auto shop)!
*
* @return the owner of this auto shop
*/
public ShopOwner getOwner() {
// ALREADY IMPLEMENTED; DO NOT MODIFY
return this.owner;
}
/**
* Allows the current owner of this auto shop to give this
* auto shop to a new owner.
*
* @param currentOwner the current owner of this auto shop
* @param newOwner the new owner of this auto shop
* @throws IllegalArgumentException if currentOwner is not the
* current owner of this auto shop
*/
public void changeOwner(ShopOwner currentOwner, ShopOwner newOwner) {
// COMPLETE THIS
}
/**
* Adds the specified vehicles to this auto shop.
*
* @param vehicles
* a list of vehicles to add to this auto shop
*/
public void add(List
// COMPLETE THIS
}
/**
* Returns true if this auto shop contains
* the specified vehicle, and false
* otherwise.
*
* @param vehicle
* a vehicle
* @return true if this auto shop contains the specified vehicle,
* and false otherwise
*/
public boolean contains(Vehicle vehicle) {
// COMPLETE THIS
}
/**
* Allows the owner of this auto shop to sell a single vehicle
* equal to the specified vehicle from this auto shop.
*
*
* If the specified user is not equal to the owner of this auto shop,
* then the vehicle is not sold from this auto shop,
* and null is returned.
*
* @param user
* the person trying to sell the vehicle
* @param vehicle
* a vehicle
* @return a vehicle equal to the specified vehicle from this auto shop,
* or null if user is not the owner of this auto shop
* @pre. the auto shop contains a vehicle equal to the specified vehicle
*/
public Vehicle sellingsingleVehicle(ShopOwner user, Vehicle vehicle) {
// COMPLETE THIS
}
/**
* Allows the owner of this auto shop to sell
* the smallest number of vehicles whose total price value in dollars
* is equal or less than to the specified price value in dollars.
*
*
* Returns the empty list if the specified user is not equal to
* the owner of this auto shop.
*
* @param user
* the person trying to sell vehicles from this auto shop
* @param pricevalue
* a value in dollars
* @return the smallest number of vehicles whose total price value
* in dollars is equal to the specified value in dollars
* from this auto shop
* @pre. the auto shop contains a group of vehicles whose total price value is equal
* to specified value
*/
public List
// COMPLETE THIS
}
/**
* Returns a deep copy of the vehicles in this auto shop.
* The returned list
* has its vehicles in sorted order
* (from smallest price value to largest price value).
*
Remember that
* the auto shop and its collection of vehicles form a composition.
*
* @return a deep copy of the vehicles in this auto shop sorted by price value
*/
public List
// COMPLETE THIS
}
/**
* Returns a Shallow copy of the vehicles in this auto shop.
* The returned list
* has its vehicles in sorted order
* (from smallest year of make value to largest year of make value).
*
Remember that
* the auto shop and its collection of vehicles form a composition.
*
* @return a show copy of the vehicles in this auto shop sorted by year of make
*/
public List
// COMPLETE THIS
}
/**
* Returns a deep copy of the vehicles in this auto shop.
* The returned list
* has its vehicles in sorted based on make and then ordered based on price value.
*
* (i.e., from smallest price value to largest price value).
*
Remember that
* the auto shop and its collection of vehicles form a composition.
*
* @return a deep copy of the vehicles in this auto shop sorted
* based on make and then ordered based on price value.
*/
public List
// COMPLETE THIS
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
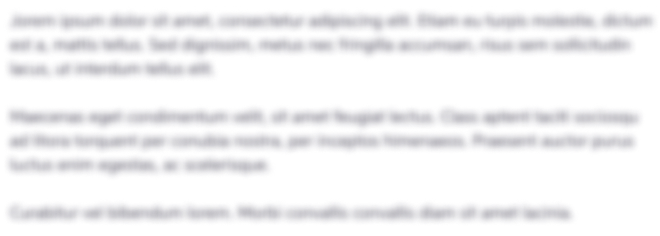
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started