Question
JAVA In a guessing game, the computer picks a number between 1 and 100 at random, and it asks the user to guess a number.
JAVA
In a guessing game, the computer picks a number between 1 and 100 at random, and it asks the user to guess a number. After each guess, it tells the user if they are too high or too low, and then lets them guess again. If the user ever guesses correctly, the computer should congratulate the user and end the program. Here's an example of me interacting with my Guessing Game:
Welcome to the guessing game! The computer's job is to select a number between 1 and 100. Your job is to guess that number! Guess the number: 50 Too low! Guess the number: 75 Too low! Guess the number: 82 Too low! Guess the number: 90 Too low! Guess the number: 95 Too high! Guess the number: 93 Too high! Guess the number: 92 Too high! Guess the number: 91 Good job! 91 was my number. You win!
- First, read through the code, and understand the structure. What state does a GuessingGame have?
- Next, try compiling the code: At the command line, type javac GuessingGame.java.
- Compiling the code should result in two errors. Read the compiler error message and try to understand why it's giving you these errors.
- Fix these errors by implementing getNumber() and getDefaultNumber() (each of these methods only needs one line of code!). Each time you finish making a change in your code, compile the program. When you've finished this part, you should no longer get any compile errors.
To implement the guessing game, you'll need to change two methods: playGame and main. Both of these methods already exist in the code, but they don't do anything yet. As you're implementing these methods, jot down compiler errors and exceptions that you get, as well as how you fixed them (Just like in Python, you'll find that it's helpful to have a mental library of what particular messages mean. Reflecting on how you fix an error can help you to build that mental library faster.)
Start off by implementing main(). The main method executes when the user runs GuessingGame from the command line: java GuessingGame (Try it, and you will see the message "Sorry, the game hasn't been programmed."). You'll need to construct a new GuessingGame object, start the game (i.e., call the startNewGame method on the GuessingGame instance you created), and play the game. Remember that unlike Python, you need to use the word new when constructing an object. Test your code and make sure it runs. (Compile your code first, and then run it.) You'll still see a message about not being able to play ("Sorry, playGame() hasn't been programmed."), since you haven't implemented playGame(), but now you're ready to play once you get that method working.
Now you're ready to write playGame(). This should get input from the user and have her guess until she's correct. Your game should provide feedback to the user about whether her guess is too high or too low. It may be helpful to talk through the high level outline of the logic for the method with your partner before turning your ideas into code.
Once you think you have playGame() working, compile your code again, and run it. You should be able to interact with the game! Try a few games to make sure that the program performs properly, and debug your code with your partner to fix any issues. Once it works, you've completed the lab!
/** * GuessingGame picks a number between 1 and 100 at random, and asks the user to guess. * After each guess, it tells the user if they are too high/too low and then lets them guess again. * Ends the program when the user guesses correctly. * Your tasks: * - Read through the code, and understand the structure. What state does a GuessingGame have? * - Implement getNumber() and getDefaultNumber() (each of these methods only needs one line of code!). Why does * the compiler give an error before you've implemented these methods? Each time you finish making a change in * your code, compile and run the program. Don't try to go through all these instructions and only at the very * end see if things are working! * - Change main to make it so that a game actually gets played, with a random number chosen. You'll need to * construct a new game, start the game, and play the game. Test your code and make sure it runs. You'll still * see a message about not being able to play, since you haven't implemented playGame(), but now you're ready * to play once you get that method working. * - Implement playGame(). This should get input from the user and have her guess until she's correct. * Your game should provide feedback to the user about whether her guess is too high or too low. * */ public class GuessingGame { //Instance variable storing the number the user is guessing private int number; //Static variable storing the default number to have the user guess. //Why have a default number? // (a) For debugging - you know exactly what number you should be guessing // and (b) For practice understanding the difference between static and non-static. private static int defaultNumber = 27; /** * Constructs a new GuessingGame. To start game, * you must call startNewGame(). Otherwise, the number to guess will always * use the same default value to guess. */ public GuessingGame() { number = defaultNumber; } /** * Returns the number the user is supposed to guess. */ public int getNumber() { /* IMPLEMENT - YOUR CODE GOES HERE */ } /** * Returns the default number for guessing (if player tries to play without starting a new game). */ public static int getDefaultNumber() { /* IMPLEMENT - YOUR CODE GOES HERE */ } /** * Changes the default number for guessing. This number is used if the player tries to play * without calling startNewGame(). * @param defaultNumber */ public static void changeDefaultNumber(int defaultNumber) { GuessingGame.defaultNumber = defaultNumber;//What happens if you don't include "GuessingGame."? Make sure you understand why. } /** * Starts a new guessing game by randomly selecting an integer between 1 and 100 for the * player to guess. */ public void startNewGame() { number = (int) Math.ceil(Math.random()*100); } /** * Plays a guessing game by having the player * guess until she correctly identifies the computer's number. */ public void playGame() { System.err.println("Sorry, playGame() hasn't been programmed."); } /** * GuessingGame main constructs a new game and has the player play a single game in which * she must guess the computer's chosen number (from 1-100). * @param args the command line arguments aren't used for this class */ public static void main(String[] args) { System.err.println("Sorry, the game hasn't been programmed."); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
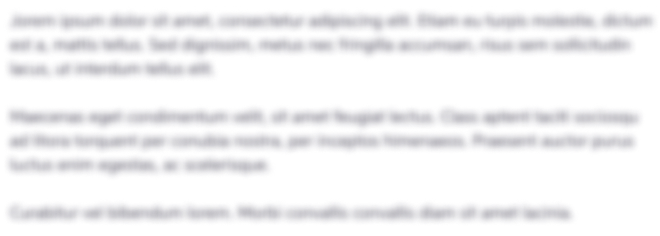
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started