Question
JAVA In Eclipse, create a Java project called Billfold. We will be creating cards and demonstrating inheritance and polymorphism. Create a class called Card (card
JAVA
In Eclipse, create a Java project called Billfold. We will be creating cards and demonstrating inheritance and polymorphism. Create a class called Card (card as in identification, credit card, etc not playing card) in Eclipse with the following code.
public class Card
{
private String name;
public Card()
{
name = "";
}
public Card(String n)
{
name = n;
}
public String getName()
{
return name;
}
public boolean isExpired()
{
return false;
}
public String format()
{
return "Card holder: " + name;
}
}
The card class is the super class. The other classes below will inherit from the card class.
Class | Data |
YMCACard | ID number |
DebitCard | Card number, PIN |
DriverLicense | DLNumber, Expiration date * |
Create these three additional classes and have them extend the Card class (use the keyword extends). For each subclass, create the private instance variables provided above. Create empty constructors and a method called format() that returns a String. For now, just have it return Not set. Also create your getters and setters in the subclasses.
* For the expiration date for the DriverLicense, we are going to use the Java 8 date and time library. In the DriverLicense class, create the expirationDate variable as a private LocalDate (private LocalDate expirationDate;). You will need to import the LocalDate when you add it (import java.time.LocalDate;).
Implement constructors for each of the three subclasses. Each constructor should call the superclass constructor to set the name. We have two constructors created in the Card class.
public Card() { name = ""; } | This constructor creates a blank card and assigns the name instance variable to an empty string. |
public Card(String n) { name = n; }
| This constructor takes a String called n and assigns n into the name instance variable. |
We can use the Card constructor in our subclasses. Here is one example:
public YMCACard(String n, String id)
{
super(n);
setIdNumber(id); //use your setIdNumber method check the capitalization
}
In this example, the YMCACard accepts two strings. The first string is passed into the super constructor from the Card superclass. It will use the super constructor from the Card class with n as the parameter being set for name. Then we use id to assign the idNumber an instance variable in the YMCACard class. Create the constructor for the DebitCard in the same manner. The DriverLicense will be a little different because of the use of LocalDate.
In your DriverLicense card, you used a data type called LocalDate. Your constructor will take the name, driver license number and a LocalDate variable. It will look like this: public DriverLicense(String n, String dln, LocalDate e)
Replace the implementation of the format method for the three subclasses. The methods should produce a formatted description of the card details. The subclass methods should call the superclass format method to get the formatted name of the cardholder. For example, the YMCACard format would look like this:
public String format()
{
return super.format() + ", ID: " + idNumber;
Devise another class, Billfold, which contains slots for two cards, card1 and card2, a method void addCard(Card n) and a method String formatCards(). The Billfold class does not extend other classes. The instance variables will look a little different this time. We will have a private Card card1 instance variable. Its a private variable of the type Card called card1. Create the instance variable for card2 in the Billfold class.
The addCard method checks whether card1 is null. If so, it sets card1 to the new card. If not, it checks card2. If both cards are set already, the method has no effect. Your if statement is quite simple: if (card1 == null)
The formatCards method invokes the format method on each card and produces a string with the format: [card1|card2]
Write a tester program called BillfoldTesterYourLastName. Create two cards. Note: when you create a DriverLicense, you have to provide an expiration date. You can do that in your constructor. It will look like this: DriverLicense dl = new DriverLicense("Amy", "0098778", LocalDate.of(2016, 5, 23));
Create a new Billfold. Note that we did not create a constructor for the billfold class so we will create a blank billfold and then add the cards to it. Add the two cards you just created to the billfold using the addCards method. Call the formatCards method from the billfold class to see what the billfold holds.
The Card superclass defines a method isExpired, which always returns false. This method is not appropriate for the driver license. Supply a method header and body for DriverLicense.isExpired() that checks whether the driver license is already expired (i.e., the expiration date is before the current date). Its return type will be a Boolean the same method signature as the method in the super class.
To work with dates, you can use the methods and constants supplied in the class DateTime. We need to create two date variables one with the expiration date and one with the current date. If the expiration date is before the current date, then this means the Driver License is expired.
To obtain the current date, use LocalDate current = LocalDate.now(); This will get the current system date and store it into the current variable. To get the date of the driver license expiration, use your method and store it into expires. LocalDate expires = this.getExpiration(); (or whatever the name of your method is that returns the expiration date).
Now we can use a built in method to find out if the expiration date is before the current date.
if(expire.isBefore(current)){
return true;
} else {
return false;
}
The YMCA and the debit card dont expire. Since the Card superclass already defines a method isExpired, which always returns false, we dont have to do anything in our subclasses.
In the Billfold class, add a method getExpiredCardCount, which counts the number of expired cards in the billfold. Your method will return an int. Inside your method, create a local variable called count and increase it by 1 if the result of card1.isExpired() is true and increase it by one if the result of card2.isExpired() is true. Return the value in count from the method.
In your BillfoldTester class, create two more cards one that is an expired drivers license. Create another billfold and populate the billfold with the expired drivers license and another card. Then call the getExpiredCardCount method. Write out to the console the number of expired cards in that wallet. Run your tester to verify that your method works correctly.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
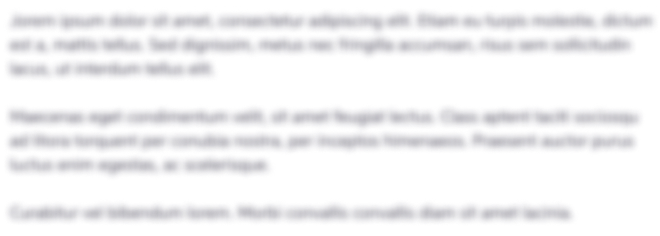
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started