Question
Java Inheritance: Build a new Employee class(Employee.java). This class will be a sub-class of Person. So it will inherit all the properties of Person(FirstName, LastName,
Java Inheritance:
Build a new Employee class(Employee.java). This class will be a sub-class of Person. So it will inherit all the properties of Person(FirstName, LastName, Address and Email). Also add 2 new properties, an employee id number and a salary. Create this class, make sure to add 2 constructors(one that takes no args and 1 that takes all props from both the Person class and the Employee class) and a display() method that prints out all the props from both the Person class and the Employee class. Then use main to test out this class.
Main code:
Employee e1;
e1 = new Employee(2323, Bill, Clinton, Marietta, bc@msn.com, 43000.00);
e1.display(); //prints all 6 properties
Here is what I got so far:
public class Person {
// ========================== Properties ===========================
private String FirstName;
private String LastName;
private String Address;
private String Email;
//constructor with parameters
Person(String FirstName,String LastName,String Address,String Email) {
this.FirstName = FirstName;
this.LastName = LastName;
this.Address = Address;
this.Email = Email;
}
//constructor with no parameters
Person() {
this.FirstName = "";
this.LastName = "";
this.Address = "";
this.Email = "";
}
// ========================== Behaviors ==========================
public void setFirstName(String fn) {
this.FirstName = fn;
}
public void setLastName(String ln) {
this.LastName = ln;
}
public void setAddress(String a) {
this.Address = a;
}
public void setEmail(String e) {
this.Email = e;
}
public String getFirstName() {
return this.FirstName;
}
public String getLastName() {
return this.LastName;
}
public String getAddress() {
return this.Address;
}
public String getEmail() {
return this.Email;
}
//method that displays person data
public void display() {
System.out.println("First Name : "+this.FirstName);
System.out.println("Last Name : "+this.LastName);
System.out.println("Address : "+this.Address);
System.out.println("Email : "+this.Email);
} //end display()
//overriding toString method
public String toString() {
return "FirstName: "+getFirstName() + " LastName: "+getLastName() + " " +this.Address.toString()
+" Email: "+getEmail();
}
//main method
public static void main(String args []) {
Person p1;
p1 = new Person("Rodney","Duncan","70 Bowman St. South Windsor, CT 06074","rduncan@msn.com");
p1.display();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
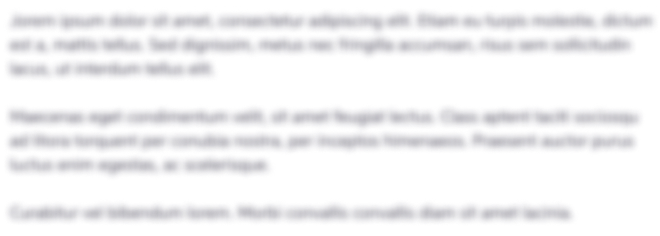
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started