JAVA JAVA JAVA JAVA JAVA JAVA JAVA I need the code below edit to meet the requirements below: NOTE: Most of the steps are finished
JAVA JAVA JAVA JAVA JAVA JAVA JAVA
I need the code below edit to meet the requirements below:
NOTE: Most of the steps are finished just need to be edited to meet requirements.
NOTE: I marked in the code where the major error is occurring. The error is incompatible types Char cannot be converted into String.
Test 1 (10 pts):
Within the EventManager class:
? Define variables to hold an amount type and an amount value.
? Using the already defined File and Scanner variables from the template,
add code to instantiate a File object and a Scanner object that can be used to read the file whose
name was input by the user.
? Read data from the first line of the file only.
? After reading the line, display a message containing the data from the line read, with the amount
formatted to 2 decimal places, as follows:
Line read: T 111.11
? Close the file.
Test 2 (15 pts):
Within the EventManager class:
? Add loop around the code that reads the data from the file and displays what was read, so that it
reads and displays all lines in the data file (should work no matter how many lines are in the file).
? After all data has been read and displayed, use the constant to display a message including the
filename, as follows:
Done reading file event1.txt
Test 3 (10 pts):
Within the Event class:
? Add a addAmount() method that:
o Has 2 parameters: an amount type letter and an amount value
o Increments the total for the amount type specified by the first parameter by the amount
specified in the second parameter
Test 4 (15 pts):
Within the Event class:
? Add a displayTotals() method that will:
o Display one blank line
o Display the data fields, as follows (with decimals aligned and final digit in column 30):
Within the EventManager class:
? Above the file reading loop,
create an Event object.
? Within the loop, as each line of data is read,
use the Event object to call the addAmount() method,
with the data read from the file line as the arguments.
? After reading all data in the file,
add code to use the Event object to call the displayTotals() method.
Total ticket sales: 2000.00
Total donations: 500.00
Total expenses: 133.33
Test 5 (10 pts):
Within the Event class:
? Add a calcEventProfit() method that:
o Will alculate and return the event profit (tickets + donations expenses)
(NOTE: The profit will be negative, if the event lost money)
Tests 6 & 7 (10 pts each):
Within the EventManager class:
? Display a blank line.
? Use the Event object to call the calcEventProfit() method
? If the returned event profit is positive (including 0), display
Event generated a profit of $ 111.11
Otherwise display
Event generated a loss of $ -111.11
Test 8 (10 pts):
Within the EventManager class:
? Remove the following clause from the main method header.
throws IOException
? Implement file exception handling:
o Add a try block to catch any errors when opening the data file.
o Add a catch block to display the following message that includes the filename:
Could not open input file data.txt. Program exiting.
EventManager.java
import java.io.File;
import java.io.FileNotFoundException;
import java.text.DecimalFormat;
/**
* Program description
* @author
*/
import java.util.Scanner;
public class EventManager {
public static void main(String[] args) {
Scanner keyboard = new Scanner(System.in);
System.out.println("Enter filename:");
String filename=keyboard.nextLine();
Scanner eventFile;
try {
eventFile = new Scanner(new File(filename));
Event event=new Event();
while(eventFile.hasNextLine()){
String line=eventFile.nextLine();
String[] values=line.split("\\s");
String amountType=values[0];
double amount=Double.parseDouble(values[1]);
event.addAmount(amountType,amount);
System.out.println("Line read: "+line);
}
event.displayTotals();
double eventProfit=event.calcEventProfit();
DecimalFormat format=new DecimalFormat("0.00");
if(eventProfit>=0){
System.out.println(" Event generated a profit of $ "+format.format(eventProfit));
}else{
System.out.println(" Event generated a loss of $ "+format.format(eventProfit));
}
keyboard.close();
eventFile.close();
} catch (FileNotFoundException e) {
System.err.println("Could not open input file "+filename+" Program exiting.");
}
}
}
Event.java
import java.text.DecimalFormat;
/**
*
* Class description
*
* @author
*
*/
public class Event {
private double ticketSales; // total profit from event ticket sales
private double donations; // total profit from event donations
private double expenses; // total expenses to put on the event
DecimalFormat format=new DecimalFormat("0.00");
public Event() {
ticketSales = 0;
donations = 0;
expenses = 0;
}
public double getTicketSales() {
return ticketSales;
}
public double getDonations() {
return donations;
}
public double getExpenses() {
return expenses;
}
// Add your code here (and do not delete any of the above code)
public void addAmount(String amountType, double amount){ // error is occuring becaus of the Chars?
if(amountType.equalsIgnoreCase("T")){
ticketSales+=amount;
}else if(amountType.equalsIgnoreCase("D")){
donations+=amount;
}else if(amountType.equalsIgnoreCase("E")){
expenses+=amount;
}else{
System.out.println("Invalid Amount type");
}
}
public void displayTotals(){
System.out.println();
System.out.println("Total ticket sales: "+format.format(ticketSales)); //edit to printf to 6 spaces
System.out.println("Total donations: "+format.format(donations)); //edit to printf to 6 spaces
System.out.println("Total expenses: "+format.format(expenses)); //edit to printf to 6 spaces
}
public double calcEventProfit(){ // TESTING EVENT METHOD calcEventProfit java:6: error: incompatible types: char cannot be converted to String event.addAmount('T', 100); java:7: error: incompatible types: char cannot be converted to String event.addAmount('D', 200); java:8: error: incompatible types: char cannot be converted to String event.addAmount('E', 160); ^
return (ticketSales+donations-expenses);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
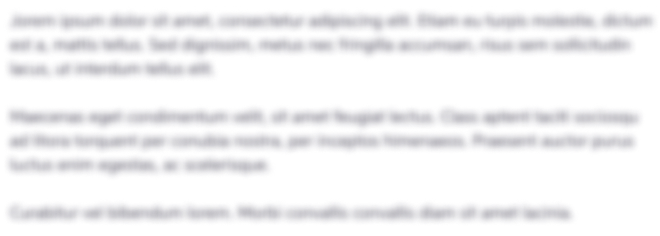
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started