Question
**JAVA language** eclipse In this assignment, you will apply your knowledge of object oriented programming and lists. You will consider the following concepts: (1) a
**JAVA language** eclipse
In this assignment, you will apply your knowledge of object oriented programming and lists.
You will consider the following concepts:
(1) a two dimensional shape which we call a Shape2D
(2) a three dimensional shape which we call a Shape3D
--------------------------------------------------------------------------------------
Description
In this assignment, you will create:
- an abstract class called Shape2D that implements Comparable
- a class Rectangle that extends Shape2D
- a class Square that extends Rectangle
- an abstract class called Shape3D
- an abstract class PlatonicSolid that extends Shape3D
- a class Main for testing your code
(10 pts) Define an abstract class named Shape2D
- Shape2D must implement Comparable
- Shape2D must include a method with signature: int compareTo(Shape2D otherShape)
- The compareTo method must compare the area of itself with the area of otherShape
- Shape2D must have an abstract method with signature: double computeArea()
- Shape2D must have an abstract method with signature: String toString()
- Shape2D must have a static method with signature: List
getLargestShapes(List shapes)
(10 pts) Define a class named Rectangle
- Rectangle must extend Shape2D
- Rectangle must have:
- a constructor with signature: Rectangle(double width, double height)
- a computeArea method that returns the product of width and height
- an equals method that checks if two Rectangles have the same width and height
- a toString method that returns the width and height of the Rectangle as a String. For example, if width = 10 and height = 3, then it would return "(10, 3)".
(5 pts) Define a class named Square
- Square must extend Rectangle
- Square must have a constructor with signature: Square(double length)
- Square's constructor must call Rectangle's constructor
(10 pts) Implement getLargestShapes method
- In the Shape2D abstract class, you must implement the static method called getLargestShapes
- The getLargestShapes method takes in a list of Shape2D's and it must return a List that contains all Shape2D's with the largest area
(5 pts) Define abstract classes named Shape3D and PlatonicSolid
- Shape3D must have an abstract method with signature: double computeSurfaceArea()
- PlatonicSolid must extend Shape3D
- PlatonicSolid must have member variables double edgeLength and int numOfFaces
- PlatonicSolid must have an abstract method with signature: double computeAreaOfOneFace()
- PlatonicSolid must implement the computeSurfaceArea method by returning numOfFaces * this.computeAreaOfOneFace();
(5 pts) Define a class named Main
- Main must contain a public static void main method
- The main method must:
- create a List of Shape2D's and populate this list with at least 8 distinct Rectangle objects
- call the getLargestShapes method and print the result
Step by Step Solution
There are 3 Steps involved in it
Step: 1
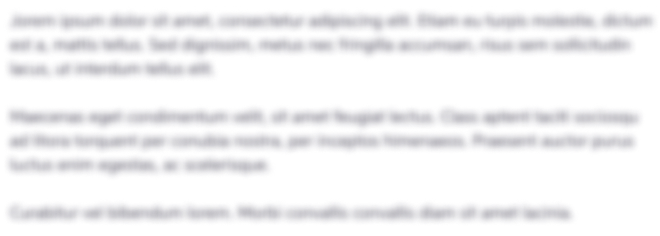
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started