Question
*JAVA LANGUAGE QUESTION* Part 1: Create a class EventItem that holds the location of an event called location (a String), the name of the event
*JAVA LANGUAGE QUESTION*
Part 1:
Create a class EventItem that holds the location of an event called location(a String), the name of the event called eventName (a String), a start hour (in 24hour format) called startTime (an integer) and an end hour (in 24 hour format) called endTime (an integer). All state values are public and a constructor is needed that takes all four parameters.
[0.5 marks]
Paste your code for Part 1 here:
Part 2:
Create a class DailyScheduler that holds EventItem objects in an array called dailySchedule (The maximum size of the array is 100). It must also have a variable that holds the maximum number of EventItems(100) and another for the actual number of EventItems.
All state variables must be initialized in a single constructor that takes no parameters.
[0.5 marks for all necessary class state declarations]
The class DailyScheduler has the following operations:
void sortAscendingByEventName() - This method sorts the EventItems in ascending order using insertion sort by their eventName. [1.25 marks]
void sortAScendingByStartTime() - This method sorts the EventItems in ascending order using insertion sort by their startTime. [0.5 marks]
void sortDescendingByLength() - This method sorts the EventItems in descending order using selection sort by their length of time (endTime startTime). [1.5 marks]
String printDayScheduleByStartTime() - This method returns a string of all EventItems with their location, eventName, startTime and endTime in ascending order by their starting time. [0.5 marks]
String printDayScheduleByLongestTime() - This method returns a string of all EventItems with their location, eventName, startTime and endTime in ascending order by their starting time. [0.25 marks]
int binarySearch(String eventName) This method uses binary search to return the location of an EventItem with the eventName matching the parameters, or -1 if not. YOU MUST ASSSUME THE ARRAY IS SORTED IN ORDER BY EVENTNAME. [2 marks]
boolean addEventItem(String location,String eventName,int startTime,int endTime) - This method must in order:
- Check if there is space to add a new EventItem (returns false if not)
- Sort the list in ascending order by eventName. [0.25 marks for 1 and 2]
- Uses binarySearch to check if there is an item with the same eventName in the array. [1 mark]
- Creates an EventItem and adds it to the end of the array if there is no item with the same eventName and location. (returns false if there is a clash and true if not)[0.75 marks]
YOU MAY ASSUME EVENT NAMES ARE UNIQUE (two EventItems will not have the same eventNames).
Paste your code for Part2 here:
Part 3
Write a main program that:
- Adds the following event items to a DailyScheduler. [0.5 marks]
Order of adding to Queue | Location | Event name | Start time | End time |
1 | Library | breakFast1 | 7 | 9 |
2 | Main Hall | Conference3 | 12 | 13 |
3 | Boardroom1 | breakFast1 | 8 | 11 |
4 | Boardroom1 | Meeting2 | 14 | 17 |
(Note that item 3 will not be added to the list by the program as it has the same eventName as item 1)
- Prints the dailySchedule (in ascending order by startTime). [0.25 marks]
- Prints all the items in descending order by their length of time. [0.25 marks]
Paste your code for Part3 here:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
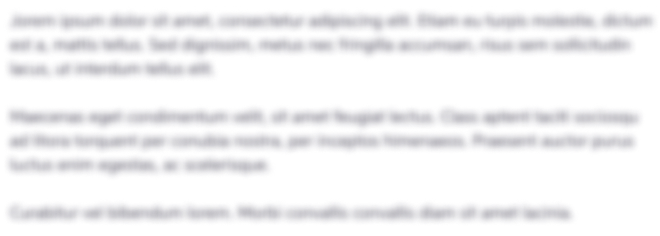
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started