Question
JAVA Line Segment Create the following classes: (1) Segment a. a constructor that takes two points p1, p2, stores them and creates a corresponding line
JAVA Line Segment Create the following classes:
(1) Segment
a. a constructor that takes two points p1, p2, stores them and creates a corresponding line object
b. a method "makeLine" that returns a line object based on p1 and p2
c. a method that computes the shortest distance to a point p.
This method uses the nearestPointTo method of the Line objects to find the nearest point p' to p.
Then it checks whether p' is between p1 and p2. If it is, then the distance between p' and p is what we want.
Otherwise, the shortest distance to p is the shortest distance between p1 and p or p2 and p.
(2) Line interface with a method nearestPointTo
(3) HorizontalLine class that implements Line
(4) VerticalLine class that implements Line
(5) SlopedLine class that implements Line The shortest distance from a line segment to a point is either the nearest point p on the line to p if the point p is within the segment or it is the shorter distance from one of the end points to p. I have everything complete except for the Line Segment class:
import org.junit.Test;
import static org.junit.Assert.*;
public class ex6 {
public static void main (String[] args) {
Point p1 = new Point(0,0), p2 = new Point(1,1), p = new Point(2,1);
Segment s = new Segment(p1, p2);
System.out.println(s.distanceTo(p)); // should print 1
}
}
//point class
class Point {
final double x, y;
public Point(double x, double y) {
this.x = x;
this.y = y;
}
public double distanceTo(Point p) {
return Math.sqrt(Math.pow(x - p.x, 2) + Math.pow(y - p.y, 2));
}
public String toString() {
return "(" + x + ", " + y + ")";
}
public boolean equals(Object that) {
boolean ret = false;
if(that instanceof Point) {
Point thatPoint = (Point) that;
ret = x == thatPoint.x && y == thatPoint.y;
}
return ret;
}
}
// line interface
interface Line {
double distanceTo(Point p);
Point nearestPointTo(Point p);
}
// horizontal line class
class HorizontalLine implements Line {
final double y;
HorizontalLine(double y) {
this.y = y;
}
public double distanceTo(Point p) {
return Math.abs(y - p.y);
}
public Point nearestPointTo(Point p) {
Point p1 = new Point(p.x, y);
return p1;
}
// vertical line class
class VerticalLine implements Line {
final double x;
VerticalLine(double x) {
this.x = x;
}
public double distanceTo(Point p) {
return Math.abs(x - p.x);
}
public Point nearestPointTo(Point p) {
Point p1 = new Point(x, p.y);
return p1;
}
}
// sloped line class
class SlopedLine implements Line {
final double m, k;
public SlopedLine(Point p1, Point p2) {
m = (p1.y - p2.y) / (p1.x - p2.x);
k = p1.y - p1.x * m;
}
public SlopedLine(double m, double k) {
this.m = m; this.k = k;
}
private SlopedLine normal(Point p) {
double m1 = -1/m; // slope of a line perpendicular to this line
return new SlopedLine(m1, p.y - p.x * m1);
}
private Point intersect(SlopedLine l) {
double x = (l.k - k) / (m - l.m);
double y = m * x + k;
return new Point(x, y);
}
public Point nearestPointTo(Point p) {
return intersect(normal(p));
}
public double distanceTo(Point p) {
return nearestPointTo(p).distanceTo(p);
}
}
//segment class
class Segment {
public Segment(Point p1, Point p2) {
}
public double distanceTo(Point p) {
return 1;
}
// tests
@Test
public void testHorizontal1() {
Point p1 = new Point(1,1), p2 = new Point(2,1), p3 = new Point(5,5);
Segment s = new Segment(p1, p2);
assertEquals(s.distanceTo(p3), 5, 0.01);
}
@Test
public void testHorizontal2() {
Point p1 = new Point(1,1), p2 = new Point(2,1), p3 = new Point(1.5,3);
Segment s = new Segment(p1, p2);
assertEquals(s.distanceTo(p3), 2, 0.01);
}
@Test
public void testVertical1() {
Point p1 = new Point(1,1), p2 = new Point(1,2), p3 = new Point(5,5);
Segment s = new Segment(p1, p2);
assertEquals(s.distanceTo(p3), 5, 0.01);
}
@Test
public void testVertical2() {
Point p1 = new Point(1,1), p2 = new Point(1,2), p3 = new Point(3,1.5);
Segment s = new Segment(p1, p2);
assertEquals(s.distanceTo(p3), 2, 0.01);
}
@Test
public void testSloped1() {
Point p1 = new Point(0,0), p2 = new Point(1,1), p3 = new Point(2,1);
Segment s = new Segment(p1, p2);
assertEquals(s.distanceTo(p3), 1, 0.01);
}
@Test
public void testSloped2() {
Point p1 = new Point(0,0), p2 = new Point(1,1), p3 = new Point(1,0);
Segment s = new Segment(p1, p2);
assertEquals(s.distanceTo(p3), Math.sqrt(2)/2, 0.01);
}
Line makeLine(Point p1, Point p2) {
Line ret;
if (p1.x == p2.x) {
ret = new VerticalLine(p1.x);
}
else if (p1.y == p2.y) {
ret = new HorizontalLine(p1.y);
}
else {
ret = new SlopedLine(p1, p2);
}
return ret;
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
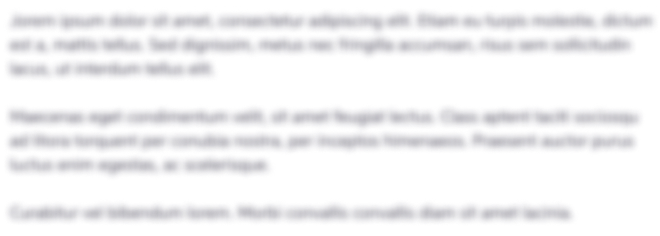
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started