Question
JAVA- Modify the code to change the Person class to only search by last name instead of first, last, and age. Make sure code compiles.
JAVA- Modify the code to change the Person class to only search by last name instead of first, last, and age. Make sure code compiles.
/////////////////////////////////////////////////
import java.util.Scanner;
public class Lab8 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int type, choice, age;
String search, fname, lname;
System.out.println("Test for String (1), Integer(2), or Person(3)? ");
choice = sc.nextInt();
System.out.println("Traverse in terms of preorder(1), inorder(2), or postorder(3)? ");
type = sc.nextInt();
switch (choice) {
case 1:
System.out.println("Enter a value to find: ");
search = sc.next();
testString(type, search);
break;
case 2:
System.out.println("Enter a value to find: ");
search = sc.next();
testInteger(type, search);
break;
case 3:
System.out.println("Enter a Person details to find: ");
System.out.println("Enter First Name : ");
fname = sc.next();
System.out.println("Enter Last Name : ");
lname = sc.next();
System.out.println("Enter Age : ");
age = sc.nextInt();
testPerson(type, new Person(fname, lname, age));
break;
default:
System.out.println("Invalid Input...");
break;
}
sc.close();
}
static void testString(int traType, String target) {
BinarySearchTree
stree.insert("George");
stree.insert("Michael");
stree.insert("Tom");
stree.insert("Adam");
stree.insert("Jones");
stree.insert("Peter");
stree.insert("Daniel");
// Traverse tree
stree.traverse(traType);
// Search for an element
Node
if(res != null)
System.out.println(target + " is found");
else
System.out.println(target + " not found");
}
static void testInteger(int traType, String target) {
BinarySearchTree
itree.insert(15);
itree.insert(10);
itree.insert(20);
itree.insert(5);
itree.insert(13);
itree.insert(11);
itree.insert(19);
// Traverse tree
itree.traverse(traType);
// Search for an element
Node
if(res != null)
System.out.println(target + " is found");
else
System.out.println(target + " not found");
}
static void testPerson(int traType, Person target) {
BinarySearchTree
ptree.insert(new Person("Max", "Hunter", 35));
ptree.insert(new Person("Jon", "Doe", 45));
ptree.insert(new Person("Hugh", "Grant", 40));
ptree.insert(new Person("Scott", "Hamilton", 29));
ptree.insert(new Person("Lee", "Xavier", 27));
ptree.insert(new Person("Alex", "Smith", 55));
ptree.insert(new Person("Bill", "Johnson", 54));
// Traverse tree
ptree.traverse(traType);
// Search for an element
Node
if(res != null)
System.out.println(target.toString() + " is found");
else
System.out.println(target.toString() + " not found");
}
}
class BinarySearchTree
private Node
public BinarySearchTree() {
root = null;
}
public Node
return find(root, e);
}
public Node
{
if(localRoot == null)
return null;
//Condition 1. we found the element
if(e.compareTo(localRoot.element) == 0) {
return localRoot;
}
//Condition 2.
//Value is less than node value. so go left sub tree
else if(e.compareTo(localRoot.element) < 0)
return find(localRoot.left, e);
//Condition 3.
//Value is greater than node value. so go right sub tree
else if(e.compareTo(localRoot.element) > 0)
return find(localRoot.right, e);
return null;
}
public void insert(E e) {
Node
if (root == null) {
root = newNode;
} else {
Node
Node
while (true) {
parent = current;
if (e.compareTo(current.element) < 0) {
current = current.left;
if (current == null) {
parent.left = newNode;
return;
}
}else if (e.compareTo(current.element) > 0){
//!!! complete the code
current = current.right;
if (current == null) {
parent.right = newNode;
return;
}
}
}
}
}
public void traverse(int traverseType) {
switch (traverseType) {
case 1:
System.out.print(" Preorder traversal: ");
preOrder(root);
break;
case 2:
System.out.print(" Inorder traversal: ");
inOrder(root);
break;
case 3:
System.out.print(" Postorder traversal: ");
postOrder(root);
break;
}
System.out.println();
}
//Preorder traversal
private void preOrder(Node
if (localRoot != null) {
System.out.print(localRoot.element + " ");
preOrder(localRoot.left);
preOrder(localRoot.right);
}
}
//Postorder traversal
private void postOrder(Node
if (localRoot != null) {
postOrder(localRoot.left);
postOrder(localRoot.right);
System.out.print(localRoot.element + " ");
}
}
//Inorder traversal
private void inOrder(Node
if (localRoot != null) {
inOrder(localRoot.left);
System.out.print(localRoot.element + " ");
inOrder(localRoot.right);
}
}
}
class Person implements Comparable
private String lastName;
private String firstName;
private int age;
public Person(String first, String last, int age) {
lastName = last;
firstName = first;
this.age = age;
}
@Override
public String toString() {
//!!! return "person info: firstName lastName, age"
return "Person : " + firstName + " " + lastName + ", " + age;
}
public int getAge() {
return age;
}
public String getName() {
return lastName;
}
@Override
public int compareTo(Person p) {
//First Name test
if((this.firstName).compareTo(p.firstName) > 0)
return 1;
else if(((this.firstName).compareTo(p.firstName) < 0))
return -1;
else //First Name equal then check last name
{
if((this.lastName).compareTo(p.lastName) > 0)
return 1;
else if(((this.lastName).compareTo(p.lastName) < 0))
return -1;
else
{ //If Last name equal test age
if(new Integer(this.age).compareTo(new Integer(p.age)) > 0)
return 1;
else if(new Integer(this.age).compareTo(new Integer(p.age)) < 0)
return -1;
}
}
//If all values are equal
return 0;
}
}
class Node
protected E element;
protected Node
protected Node
public Node(E e) {
element = e;
left = right = null;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
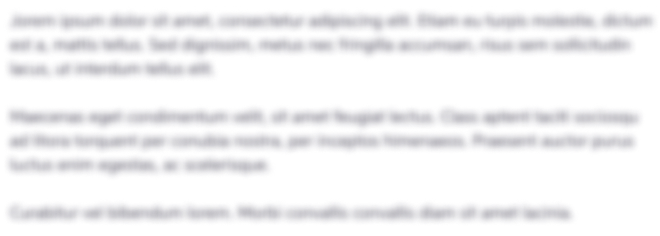
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started