Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Java Modify the IntegerSet class by adding methods to handle the set functions difference() and union(). Each should accept an IntegerSet object as its single
Java
Modify the IntegerSet class by adding methods to handle the set functions difference() and union(). Each should accept an IntegerSet object as its single parameter and return the resulting IntegerSet:
IntegerSet difference( IntegerSet incoming )
IntegerSet union( IntegerSet incoming )
In addition, add tests to the IntegerSetTest class to handle the new methods. Be sure to test all possible cases (especially with null and empty sets).
public class IntegerSet { // --------------------------------------------------------------------- // Declarations // --------------------------------------------------------------------- private ArrayList< Integer > model; /** * constructor - init model object. */ public IntegerSet() { model = new ArrayList<>(); } // constructor model /**************************** public Methods *************************/ /** * add - add an int to the collection (convert to Integer). * * **MLN 9/19/2016 - added uniqueness condition (using contains() ) * * @param newInt the int to add */ public void add( int newInt ) { // 1. Test to see if list is empty or if the new item should be at // the end. Ensure it is not there first if ( !model.contains( new Integer( newInt ) ) ) { if ( size() == 0 || isLast( newInt ) ) { append( newInt ); } else { insert( newInt ); } } } // method add /** * get - return a single ArrayList element. * * @param index - the index of the item to get * @return Integer */ public int get( int index ) { int valToReturn = -1; if ( index >= 0 && index < size() ) { valToReturn = model.get( index ).intValue(); } return valToReturn; } // method get /** * intersection - return the intersection of 2 sets. * * **MLN 9/19/2016 - new for HW08 * * @param other - the incoming set * @return the intersection of 2 sets */ public IntegerSet intersection( IntegerSet other ) { IntegerSet result = new IntegerSet(); if ( other != null ) { for ( int i = 0; i < other.size(); i++ ) { if ( model.contains( other.get( i ) ) ) { result.add( other.get( i ) ); } } } return result; } // method intersection /** * size - return the size of the ArrayList. * * @return int */ public int size() { return model.size(); } // method size /** * subset - returns true if incoming is a subset of the current set. * * **MLN 9/19/2016 - new for HW08 * * @param other - the set to test * @return true if the incoming set is a subset of the current set */ public boolean subset( IntegerSet other ) { boolean isSubset = true; if ( other != null && other.size() > 0 ) { for ( int i = 0; i < other.size() && isSubset; i++ ) { if ( !model.contains( other.get( i ) ) ) { isSubset = false; } } } return isSubset; } /******************************* private methods **********************/ /** * append - add to the end of the list. * * @param newInt the int to append */ private void append( int newInt ) { model.add( new Integer( newInt ) ); } // method append /** * insert - insert an Integer into the list at its proper position. * * @param newInt the int to insert */ private void insert( int newInt ) { if ( newInt < model.get( model.size() - 1 ).intValue() ) { int index = 0; // locate the insert point while ( newInt > ( model.get( index ) ).intValue() ) { index++; } model.add( index, new Integer( newInt ) ); // insert Integer at // index } else { append( newInt ); } } // method insert /** * isLast - return true if the object is bigger than the last object in the * list. * * @param comparableInt - an int to compare * @return true if this is greater than the last value */ private boolean isLast( int comparableInt ) { boolean isLast = true; if ( model.size() > 0 ) { isLast = comparableInt >= model.get( model.size() - 1 ).intValue(); } return isLast; } // method isLast } // class IntegerSet
**********************************************************************************************
public class IntegerSetTest { private IntegerSet source = new IntegerSet(); private IntegerSet other = new IntegerSet(); private IntegerSet result = new IntegerSet(); /** * Setup method. */ @Before public void setup() { source = new IntegerSet(); other = new IntegerSet(); result = new IntegerSet(); } /** * Test add with no duplicates. */ @Test public void testAddNoDuplicates() { source.add( 3 ); source.add( 5 ); source.add( 9 ); source.add( 1 ); source.add( 12 ); source.add( 6 ); assertTrue( source.size() == 6 ); } /** * Test add with duplicates. */ @Test public void testAddWithDuplicates() { source.add( 3 ); source.add( 5 ); source.add( 3 ); source.add( 5 ); source.add( 5 ); source.add( 1 ); assertTrue( source.size() == 3 ); } /** * Test ordering. */ @Test public void testAddingInOrder() { boolean inOrder = true; source.add( 3 ); source.add( 5 ); source.add( 9 ); source.add( 1 ); source.add( 12 ); source.add( 6 ); for ( int i = 0; i < source.size() - 1 && inOrder; i++ ) { if ( source.get( i ) > source.get( i + 1 ) ) { inOrder = false; } } assertTrue( inOrder ); } /** * Test intersection with empty source. */ @Test public void testIntersectionEmptySource() { other.add( 3 ); other.add( 5 ); result = source.intersection( other ); assertTrue( result.size() == 0 ); } /** * Test intersection with empty other. */ @Test public void testIntersectionEmptyOther() { source.add( 3 ); source.add( 5 ); result = source.intersection( other ); assertTrue( result.size() == 0 ); } /** * Test intersection with empty source and other. */ @Test public void testIntersectionEmptyBoth() { result = source.intersection( other ); assertTrue( result.size() == 0 ); } /** * Test valid intersection. */ @Test public void testIntersectionValid() { source.add( 3 ); source.add( 5 ); other.add( 7 ); other.add( 5 ); result = source.intersection( other ); assertTrue( result.size() == 1 ); } /** * Test intersection with no match. */ @Test public void testIntersectionNoMatch() { source.add( 3 ); source.add( 5 ); other.add( 7 ); other.add( 9 ); result = source.intersection( other ); assertTrue( result.size() == 0 ); } /** * Test valid subset. */ @Test public void testSubsetGood() { source.add( 3 ); source.add( 5 ); source.add( 7 ); other.add( 7 ); other.add( 3 ); assertTrue( source.subset( other ) ); } /** * Test invalid subset. */ @Test public void testSubsetNotGood() { other.add( 3 ); other.add( 5 ); other.add( 7 ); source.add( 7 ); source.add( 3 ); assertFalse( source.subset( other ) ); } /** * Test subset with empty source. */ @Test public void testSubsetSourceEmpty() { other.add( 3 ); other.add( 5 ); other.add( 7 ); assertFalse( source.subset( other ) ); } /** * Test subset with empty other. */ @Test public void testSubsetOtherEmpty() { source.add( 3 ); source.add( 5 ); source.add( 7 ); assertTrue( source.subset( other ) ); } /** * Test subset with empty source and other. */ @Test public void testSubsetBothEmpty() { assertTrue( source.subset( other ) ); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
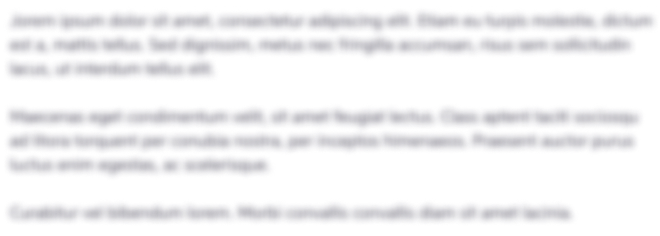
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started