Question
Java: Need to fill out the model class from the stub written. Also need to write a JUnit test class import java.io.*; import java.util.Calendar; public
Java:
Need to fill out the model class from the stub written. Also need to write a JUnit test class
import java.io.*;
import java.util.Calendar;
public class Project {
class Publication
{
String author;
String title;
String city;
String publisher;
int year;
Publication( String author,String title,String city,String publisher,int year )
{
setAuthor( author );
setCity( city );
setPublisher( publisher );
setTitle( title );
setYear ( year );
}
public boolean canAdd()
{
int authorLength,titleLength;
authorLength=author.length();
titleLength=title.length();
if ((authorLength>0)&&(titleLength>0)&&((year>1450)||(year==0)))
{
return true;
}
else
{
return false;
}
}
public String getCity()
{
return city;
}
public String getPublisher()
{
return publisher;
}
public String getTitle()
{
return title;
}
public int getYear()
{
return year;
}
public boolean setAuthor(String author)
{
int len;
len=author.length();
if (len>0)
{
this.author=author;
return true;
}
else if(len==0)
{
this.author="";
return false;
}
return false;
}
public void setCity(String city)
{
int len;
len=city.length();
if (len>0)
{
this.city=city;
}
else if(len==0)
{
this.city="";
}
}
public void setPublisher(String publisher)
{
int len;
len=publisher.length();
if (len>0)
{
this.publisher=publisher;
}
else if(len==0)
{
this.publisher="";
}
}
public boolean setTitle(String title)
{
int len;
len=title.length();
if (len>0)
{
this.title=title;
return true;
}
else if(len==0)
{
this.title="";
return false;
}
return false;
}
public boolean setYear(int year)
{
int currentYear=Calendar.getInstance().get(Calendar.YEAR);
if (((year>=1450)&&(year<=currentYear+1))||(year==0))
{
this.year=year;
return true;
}
else
{
return false;
}
}
public String toString()
{
String str;
str="Author: "+author+" City: "+city+" Publisher: "+publisher+" Title: "+title+" Year: "+year+" ";
return str;
}
}
class Bibliography
{
Publication arr[];
int last;
int Capacity;
Bibliography()
{
last=-1;
Capacity=10;
arr=new Publication[Capacity];
}
public boolean add(Publication pub)
{
boolean check;
check=pub.canAdd();
if(check)
{
if(last==(capacity()-1))
{
int i;
Publication arr2[]=new Publication[capacity()*2];
for(i=0;i<=last;i++)
{
arr2[i]=arr[i];
}
arr=null;
arr=arr2;
arr2=null;
Capacity=capacity()*2;
}
last++;
arr[last]=pub;
return true;
}
else
{
return false;
}
}
public int capacity()
{
return Capacity;
}
public boolean deleteLast()
{
if (last==-1)
{
System.out.println("No publication object found.");
return false;
}
else
{
last--;
return true;
}
}
public Publication get(int whichOne)
{
if(whichOne>last)
{
return null;
}
else
{
return arr[whichOne];
}
}
public int size()
{
return last;
}
}
public class JUnit
{
public void main(String args[])throws IOException
{
Bibliography obj=new Bibliography();
{
}
}
}
}
Publication: This class represents a single publication. It will need instance variables to store the author (String), title (String), city (String), publisher (String), and year of publication (int). It will need methods to set and get these values. It will also need a toString() method that returns the String required for the display list. This class will require the following methods:
public Publication( String author, String title, String city, String publisher, int year ) This constructor will set the values of the incoming parameters to the respective instance variables. You should use the various set... methods below to accomplish the assignments.
public boolean canAdd() This method will return true if the Publication object is correctly configured and false otherwise. A Publication object will be correctly configured if both the author and title attributes contain values (!null && length > 0) and the year is valid (between 1450 and the year after this one or 0 if not entered).
public String getAuthor() This method will return the author String. public String getCity() This method will return the city String.
public String getPublisher() This method will return the publisher String.
public String getTitle() This method will return the title String.
public int getYear() This method will return the year (as an int).
public boolean setAuthor( String author ) This method will set the value of the author attribute. The method will return true if the value of author is valid (!null && length > 0). If the incoming parameter is either null or an empty String, then the method should assign an empty String to author and return false.
public void setCity( String city ) This method will set the value of the city attribute. If the incoming parameter is null, then the value should be set to an empty String ().
public void setPublisher( String publisher ) This method will set the value of the publisher attribute. If the incoming parameter is null, then the value should be set to an empty String ().
public boolean setTitle( String title ) This method will set the value of the title attribute. The method will return true if the value of title is valid (!null && length > 0). If the incoming parameter is either null or an empty String, then the method should assign an empty String () to title and return false.
public boolean setYear( int year ) This method sets the value of the year attribute. The method will return true if the value of year is valid (>= 1450 && <= current year + 1) and false otherwise. Note: zero (0) is also allowed if no year is being stored. Note: this method should accept and maintain whatever value is sent. If the date is invalid, then the method should return false and the canAdd() method should return false.
public String toString() this method returns the String required for the display list (see above).
Bibliography: This class represents the collection of publications used by your program. It will need an instance variable to represent the collection this will be built around an array of Publication objects as well as an int variable called last that indicates the position in the array for the last element added. As a collection, this class will require methods to add a Publication to the collection, delete a Publication from the collection, and get (return) a Publication from the collection. You should also provide a method that returns the size of the collection (the number of Publications currently stored, not the size of the array). This class will require the following public methods:
public boolean add( Publication pub ) This method adds a Publication to the end of the list. This method will return true if the Publication can be added successfully. A Publication can be added successfully if its canAdd() method returns true. If the Publication cannot be added (if its canAdd() method returns false), then the method should return false. Note: this method will need to check the size of the array to ensure that there is room to add the Publication. If the array is full, the method will need to double the size of the array as indicated below. You will need to increment the value of last whenever a Publication is added.
public int capacity() This method returns the current size (capacity) of the array. public boolean deleteLast() This method removes from the array the last Publication object that was added. You will need to decrement the value of last whenever a Publication is removed.
public Publication get( int whichOne ) This method will return the Publication object found at the position indicated by whichOne. If the value of whichOne is invalid, then the method should return null.
public int size() This method returns the size of the collection (the number of Publication objects currently stored). This is not the same as the capacity of the array (unless, of course, the array is full).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
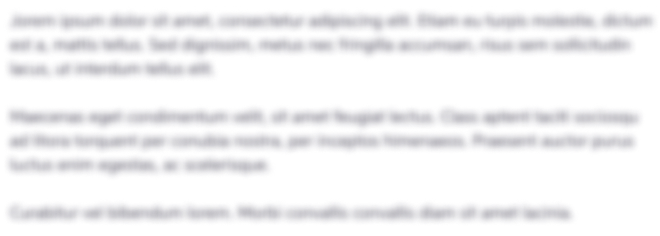
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started