Question
Java: Need to modify the code ****************************************************************************************** public class BiblioControl { public static final int LIMIT = 16; public static final int CURRENT_YEAR = Calendar.getInstance()
Java: Need to modify the code
******************************************************************************************
public class BiblioControl {
public static final int LIMIT = 16;
public static final int CURRENT_YEAR = Calendar.getInstance()
.get( Calendar.YEAR );
// finals for functions
public static final int ADD = 0;
public static final int DELETE = 1;
public static final int EDIT = 2;
public static final int LIST = 3;
public static final int SEARCH = 4;
public static final String NL = " ";
private int page; // which page are we on (0-based)
private int spaces; // required for correct placement of error messages
private BiblioUserIO io;
private Bibliography bibliography;
/**
* Default constructor.
*/
public BiblioControl() {
page = 0;
spaces = 0;
io = new BiblioUserIO();
bibliography = Bibliography.getInstance();
}
/**
* The entry point into the application.
*
* @throws IOException the IOException
*/
public void run() throws IOException {
char choice = '~'; // default to something ridiculous
BiblioFileHandler handler = new BiblioFileHandler();
// before doing anything else, read the bibliography.txt file if there
handler.readFile();
do {
displayMenu();
choice = getChoice();
actOnChoice( choice );
}
while ( choice != 'q' );
handler.writeFile();
}
/**************************** private methods ****************************/
/**
* Act on the choice of the user.
*
* @param choice the char representing the user choice
* @throws IOException the IOException
*/
private void actOnChoice( char choice ) throws IOException {
switch ( choice ) {
case 'a':
add();
break;
case 'b':
backPage();
break;
case 'd':
delete();
break;
case 'e':
edit();
break;
case 'l':
list();
break;
case 'n':
nextPage();
break;
case 's':
search();
break;
case 'q':
quit();
break;
default:
io.display( "Oops!!" + NL );
}
}
/**
* Add a book.
*
* @throws IOException the IOException
*/
private void add() throws IOException {
int year = 0;
Publication newPub;
String author = "";
String city = "";
String publisher = "";
String title = "";
io.clearScreen();
io.center( "Add Book" + NL );
io.display( NL + NL );
io.display( String.format( "%-11s", "Author:" ) );
author = validate( "Author:", io.getInput() );
io.display( String.format( "%-11s", "Title:" ) );
title = validate( "Title:", io.getInput() );
io.display( String.format( "%-11s", "City:" ) );
city = io.getInput().trim();
io.display( String.format( "%-11s", "Publisher:" ) );
publisher = io.getInput().trim();
io.display( String.format( "%-11s", "Year:" ) );
year = validate( ADD, io.getInput() );
if ( confirmYesNo( "Add (Y/N): " ) ) {
newPub = new Publication( author, title, city, publisher, year );
bibliography.add( newPub );
}
// if this takes us to a new page, then go there
// (but only if we're on the former last page).
if ( bibliography.size() > ( page * LIMIT + 16 )
&& ( bibliography.size()
- ( page * LIMIT + 16 )
nextPage();
}
io.clearScreen();
}
private boolean confirmYesNo(String string) {
// TODO Auto-generated method stub
return false;
}
/**
* Move back a page if possible.
*/
private void backPage() {
if ( isPriorPage() ) {
page--;
}
io.clearScreen();
}
/**
* Choose which book to edit/delete.
*
* **MLN (PA2) Added for PA2
*
* @param function Edit or Delete
* @return the book number
* @throws IOException the IOException
*/
private int chooseBook( String function ) throws IOException {
boolean valid = true;
int bookNum = -1;
int lowerLimit = page * LIMIT + 1;
int upperLimit = bibliography.size() > page * LIMIT + LIMIT
? page * LIMIT + LIMIT
: bibliography.size();
String choice;
io.indent( spaces, function + " Publication Number -> " );
choice = io.getInput();
do {
valid = Utilities.isValidInt( choice, lowerLimit, upperLimit );
if ( valid ) {
bookNum = Integer.parseInt( choice );
} else {
io.display( NL );
io.indent( spaces,
"Must be a number between " + lowerLimit
+ " and " + upperLimit
+ ". Please re-enter." + NL );
io.indent( spaces, function + " Publication Number -> " );
choice = io.getInput();
// io.display( NL );
}
}
while ( !valid );
return bookNum;
}
/**
* Delete a book.
*
* **MLN (PA2) -- Modified for PA2 to delete the item specified
*
* @throws IOException the IOException
*/
private void delete() throws IOException {
int bookNum = chooseBook( "Delete" );
io.clearScreen();
io.center( "Delete Book" + NL );
io.display( NL );
io.display( NL );
if ( bibliography.size() > 0 ) {
Publication pub = bibliography.get( bookNum - 1 );
if ( Utilities.confirmYesNo( "Delete \"" + pub.getShortTitle()
+ "\"? (Y/N ) -> " ) ) {
bibliography.delete( bookNum - 1 );
}
} else {
io.pause( "Nothing to delete." + NL
+ "Press
}
// if this was the only item on the current page, then go back a page
if ( bibliography.size()
backPage();
}
io.clearScreen();
}
/**
* Display the list of choices to the users.
*/
private void displayChoices() {
io.display( NL );
spaces = io.center( "(A)dd, (E)dit, (D)elete, (S)earch, read(L)ist, "
+ "(N)ext, (B)ack, (Q)uit" + NL );
io.display( NL );
io.indent( spaces, "Choose Operation -> " );
}
/**
* Display the list of publications.
*/
private void displayList() {
String itemWidth = "";
String highestWidth = "" + ( page * LIMIT + 16 );
for ( int i = 1; i
itemWidth = "" + ( page * LIMIT + i );
io.indent( highestWidth.length() - itemWidth.length(),
( page * LIMIT + i ) + ". "
+ getListEntry( page * LIMIT + i )
+ NL );
}
}
/**
* Display the menu.
*/
private void displayMenu() {
// Let's start up cleanly
io.clearScreen();
displayTitle();
displayList();
displayChoices();
}
/**
* Display the title of the screen.
*/
private void displayTitle() {
io.center( "JMUBiblio Bibliography Manager" + NL );
io.center( "CS159 (Fall 2017)" + NL );
io.display( NL + NL );
}
/**
* Edit books.
*
* **MLN (PA2) - Edit functionality added for PA2
*
* @throws IOException the IOException
*/
private void edit() throws IOException {
int year = 0;
Publication newPub;
String author = "";
String city = "";
String publisher = "";
String title = "";
String yearAsString = "";
int bookNum = chooseBook( "Edit" );
newPub = bibliography.get( bookNum - 1 );
io.clearScreen();
io.center( "Edit Book" + NL );
io.display( NL + NL );
io.display( "Author (" + newPub.getAuthor() + ")" + NL );
io.display( " NewValue - > " );
author = io.getInput();
author = author.length() > 0
? validate( " New Value -> ", author.trim() )
: newPub.getAuthor();
io.display( "Title (" + newPub.getTitle() + ")" + NL );
io.display( " NewValue - > " );
title = io.getInput();
title = title.length() > 0
? validate( " New Value -> ", title.trim() )
: newPub.getTitle();
io.display( "City (" + newPub.getCity() + ")" + NL );
io.display( " NewValue - > " );
city = io.getInput();
city = city.length() > 0
? city.trim()
: newPub.getCity();
io.display( "Publisher (" + newPub.getPublisher() + ")" + NL );
io.display( " NewValue - > " );
publisher = io.getInput();
publisher = publisher.length() > 0
? publisher.trim()
: newPub.getPublisher();
io.display( "Year (" + ( newPub.getYear() == 0
? "N/A"
: newPub.getYear() )
+ ")" + NL );
io.display( " NewValue - > " );
yearAsString = io.getInput();
year = yearAsString.length() > 0
? validate( EDIT, io.getInput().trim() )
: newPub.getYear();
if ( Utilities.confirmYesNo( "Confirm Changes (Y/N): " ) ) {
newPub.setAuthor( author );
newPub.setTitle( title );
newPub.setCity( city );
newPub.setPublisher( publisher );
newPub.setYear( year );
// remove old & add new
bibliography.delete( bookNum - 1 );
bibliography.add( newPub );
}
io.clearScreen();
}
/**
* Get a valid choice from the user. Must be in 'abdelnqs".
*
* @return the user's choice
* @throws IOException the IOException
*/
private char getChoice() throws IOException {
boolean valid = false;
String choices = "abdelnqs";
String entry;
entry = io.getInput();
do {
entry = entry.toLowerCase().trim();
if ( entry.length() == 1 && choices.indexOf( entry ) > -1 ) {
valid = true;
} else {
io.display( NL );
io.indent( spaces, "Incorrect entry (" + entry
+ "). Please re-enter." + NL );
io.indent( spaces, "Choose operation -> " );
entry = io.getInput();
}
}
while ( !valid );
return entry.charAt( 0 );
}
/**
* Get the line item for the listing.
*
* @param whichOne which entry to get
* @return to String to print in the listing
*/
private String getListEntry( int whichOne ) {
String line = "";
if ( whichOne
Publication pub = bibliography.get( whichOne - 1 );
line = pub.toString();
}
return line;
}
/**
* Is there a page before this one?
*
* @return true if there is a prior page
*/
private boolean isPriorPage() {
return page > 0;
}
/**
* Is there a page after this one?
*
* @return true if there is another page
*/
private boolean isNextPage() {
return bibliography.size() > page * LIMIT + LIMIT;
}
/**
* Search for books.
*
* @throws IOException the IOException
*/
private void list() throws IOException {
io.clearScreen();
io.center( "Reading List" + NL );
io.display( NL + NL + "This Function is not currently available."
+ NL );
io.pause( "Press
io.clearScreen();
}
/**
* Go to the next page if possible.
*/
private void nextPage() {
if ( isNextPage() ) {
page++;
}
io.clearScreen();
}
/**
* Search for books.
*
* @throws IOException the IOException
*/
private void search() throws IOException {
io.clearScreen();
io.center( "Search Books" + NL );
io.display( NL + NL + "This Function is not currently available."
+ NL );
io.pause( "Press
io.clearScreen();
}
/**
* Quit the application.
*/
private void quit() {
io.clearScreen();
}
/**
* Validate the year given the string incoming.
*
* **MLN (PA2) -- Modified for PA2 - add function add or edit
*
* @param function Add or Edit
* @param str the string to convert and test
* @return the valid year
* @throws IOException the IOException
*/
private int validate( int function, String str ) throws IOException {
String input = str;
String prompt = function == ADD
? String.format( "%-11s", "Year:" )
: " NewValue - > ";
if ( input.trim().length() == 0 ) {
input = "0";
}
while ( !Utilities.isValidYear( input ) ) {
io.display( NL );
io.display( "Must be a year between 1450 and "
+ ( CURRENT_YEAR + 1 )
+ ". Please re-enter." + NL );
io.display( prompt );
input = io.getInput();
io.display( NL );
}
return Integer.parseInt( input );
}
/**
* Ensure string has length > 0.
*
* @param prompt the prompt to print
* @param str the string to test
*
* @return the validated string
* @throws IOException the IOException
*/
private String validate( String prompt, String str ) throws IOException {
String input = str;
while ( input.trim().length() == 0 ) {
io.display( NL );
io.display( "Required entry. Please re-enter." + NL );
io.display( String.format( "%-11s", prompt ) );
input = io.getInput();
io.display( NL );
}
return input.trim();
}
}
9. Search. 1 If the user chooses "S" or "s (for "Search"), the program will ask the user to specify the values for the fields on which they want to search (author and/or title). The following sym bols have special meaning for the search function: Example ^Ern $gway also R 1( ^Ern) bol Meanin starts with: ends with: contains: not: Translation Starts with "Ern'" Ends with "gway" Contains "also R" Does not start with "Ern The program will prompt multiple times for artist and title matches. Pressing KENTER> without entering a search term will move from artist to title and from title to executing the search. Note: searches will be case insignificant (in other words, case does not matter) The program will print 25 blank lines to the screen (to clear the original screen) and then print the following: Search PublicationsStep by Step Solution
There are 3 Steps involved in it
Step: 1
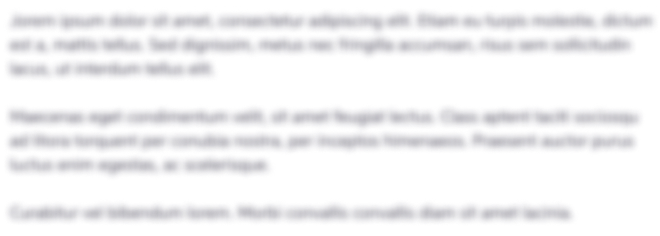
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started