Question
Java Objective Write a program that reads in lines from the input. For every pair of line, it creates Lamp objects and operates on them
Java
Objective
Write a program that reads in lines from the input.
For every pair of line, it creates Lamp objects and operates on them as per instructions in the lines. Then, it prints out information on the status of each Lamp.
The important part of this assignment is to make use of the Lamp class. Details are given after an explanation of what the program is to do.
Input
The input will be one or more PAIRS of lines.
Each pair of lines will have on the first line names of lights and on the second line will have the states of the lines. The first line will have an integer followed by that many strings consisting of names of colors.
Example: 2 green gray The second line will have for each lamp a 0 or 1 . A 0 will signify that the lamp is to be turned OFF and a 1 will indicate that the particular lamp is to turned ON.
Example: 2 green gray followed by 1 1 will indicate that both the green and the gray lamps are to be turned on.
Output
Each pair of input lines will be followed by ONE line of output indicating the status of the lamps described in the input. In the above example, the output will be "green is ON gray is ON".
Sample Input
2 green gray
1 1
1 orange
0
Sample Output
green is ON gray is ON
orange is OFF
Now that you know what this program is to do, let us get into more details of what you need to do.
You will need to write code for TWO classes ( a Lamp class and a Main class).
Lamp class
1. The Lamp class should have TWO instance variables a boolean variable to indicate the status of the Lamp a String variable that will indicate the name of the Lamp
2. The Lamp class should have FIVE methods
a. a constructor public Lamp(String s) that will set the name of the Lamp to the String s.
b. public void turnOn() that will set the status of the Lamp to true.
c. public void turnOff() that will set the status of the Lamp to false.
d. public boolean isOn() that will return the value of the status
e. public String toString() that will return a String consisting of name + " is " + "ON" (if the Lamp's status is true) OR name + " is " + "OFF" (if the Lamp's status is false)
Main class
The Main class should have the main method (public static void main(String [] args) {})
The main method should keep reading lines
1. // process the FIRST line of the pair a. it should read in the number of lamps b. then it should read in the names of the lamps, create a new Lamp, and store the lamps in an array.
2. // process the second line of the pair a. Next, it should read in an int from the line and take a turnOn or turnOff action on the particular lamp (which is in the array). It should do this for all the lamps.
3. // print the output a. traverse the lamp array and print each lamp (use System.out.print(lamp + " "); )
4. remember to print a final println after printing the lamps.
Thank you!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
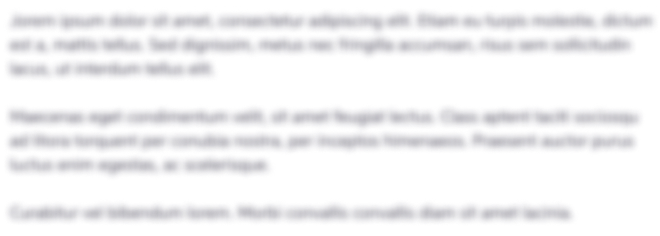
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started