Question
JAVA - Part A and B - *USE HINTS GIVEN TO MAKE THE CODE* (Please help I will give thumbs up if it works. Thanks
JAVA - Part A and B - *USE HINTS GIVEN TO MAKE THE CODE* (Please help I will give thumbs up if it works. Thanks you!!)
HINTS:
BST.java [starter code] - use for PART A
import java.util.ArrayList;
/** BST implementation for Dictionary ADT */ class BST
/** Constructor */ BST() { root = null; nodecount = 0; }
/** Reinitialize tree */ public void clear() { root = null; nodecount = 0; }
/** * Insert a record into the tree. * * @param k * Key value of the record. * @param e * The record to insert. */ public void insert(K k, E e) { root = inserthelp(root, k, e); nodecount++; }
/** * Remove a record from the tree. * * @param k * Key value of record to remove. * @return Record removed, or null if there is none. */ public E remove(K k) { E temp = findhelp(root, k); // find it if (temp != null) { root = removehelp(root, k); // remove it // System.out.println("called removehelp"); nodecount--; } return temp; }
/** * Remove/return root node from dictionary. * * @return The record removed, null if empty. */ public E removeAny() { if (root == null) return null; E temp = root.element(); root = removehelp(root, root.key()); --nodecount; return temp; }
/** * @return Record with key k, null if none. * @param k * The key value to find. */ public E find(K k) { return findhelp(root, k); }
/** @return Number of records in dictionary. */ public int size() { return nodecount; }
private E findhelp(BSTNode
private BSTNode
private BSTNode
private BSTNode
else { rt.setLeft(deletemin(rt.left())); return rt; } }
/** * Remove a node with key value k * * @return The tree with the node removed */ private BSTNode
/** * Creates a list storing the the nodes in the subtree of a node, ordered * according to the inorder traversal of the subtree. */
protected void inorderElements(BSTNode
/** Returns an iterable collection of the tree nodes. */ public Iterable
public Iterable
protected void findAllhelp(BSTNode
/* the following are for solving the exercises in Shaffer, ch. 5 */
public Iterable
protected void rangehelp(BSTNode
PrintRange.java [starter code] -- use for PART B
import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner; import java.util.ArrayList;
/* * Print out all dictionary records in a given range of keys. */ public class PrintRange {
public static void main(String[] args) { BST
// Read in the (shuffled) cmu pronunciation dictionary. try { Scanner scanner = new Scanner(file); while (scanner.hasNextLine()) { String line = scanner.nextLine(); if (line.substring(0, 3).equals(";;;")) continue; // skip comment lines Pronunciation p = new Pronunciation(line); PDict.insert(p.getWord(), p); // key dictionary on word } scanner.close(); } catch (FileNotFoundException e) { e.printStackTrace(); }
Scanner input = new Scanner(System.in); String a = input.next(); // first word in range String b = input.next(); // last word in range for(Pronunciation p : PDict.range(a,b)) System.out.println(p.getWord()+" "+p.getPhonemes()); } }
(a) Add a method levelorder Elements() to the BST.java binary search tree dictionary implementation on Vocareum. In level order the root comes first, then all nodes of level 1, then all nodes of level 2, and so on. Within a level, the order is left to right; i.c., in ascending order. Model your method on inorder Elements(). Test your method by changing the calls to inorderElements() in values() to levelorder Elements(). This way, values() will return the values in the dictionary in level order. Hint: Preorder traversals make use of a stack through recursive calls. Consider making use of another data structure to help implement the levelorder traversal. (b) The BST class has a method range() with the following declaration: public IterableStep by Step Solution
There are 3 Steps involved in it
Step: 1
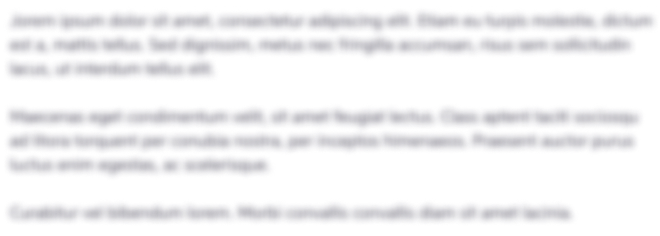
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started