Question
Java Peer-to-Peer Develop a peer-to-peer system for storing files. It is based on Chord. The processors and the files are mapped to an unique identifier
Java Peer-to-Peer
Develop a peer-to-peer system for storing files. It is based on Chord. The processors and the files are mapped to an unique identifier using a hash function that distributes them uniformly. Thus, when a node joins or leaves the systems, only O(1/n) keys are needed to be move where n is the number of processors. Each processor stores the IP and port of the peers that have joined.. The Chord overlay uses an ordered logical ring of size 2m. A key k gets assigned to the first node such that its node identifier equals or follows the key identifier of k in the common identifier space. Each processor i maintains a routing table, called the finger table, with at most O(log n) distinct entries, such that the x-th entry (1 x m) is the processor of the node succ(i + 2x1 ). The following code uses an RPC notation:
Join(ip, port): Join the system. First, peers obtain the GUID base on the IP and Port and then it stores in a file and distributed. put(GUID, data): Stores data in replicas at all nodes responsible for the object identified by GUID. remove(GUID): Deletes all references to GUID and the associated data. value = get(GUID): Retrieves the data associated with GUID from one of the nodes responsible for it. print: Print the state of the systems.
integer: successor initial value; integer: predecessor initial value; integer f inger[1...m]; integer: next f inger 1; (1) i.Locate Successor(key); where key 6= i: (1a) if key (i, successor] then (1b) return(successor) (1c) else (1d) j Closest P receding Node(key); (1e) return (j.Locate Successor(key). (2) i.Closest P receding Node(key); where key 6= i: (2a) for count = m down to 1 do (2b) if f inger[count] (i, key] then (2c) break(); (2d) return(f inger[count]). (3) i.Create New Ring: (3a) predecessor ; (3b) successor i. (4) i.Join Ring(j), where j is any node on the ring to be joined: (4a) predecessor ; (4b) successor j.Locate Successor(i). (5) i.Stabilize: executed periodically to verify and inform successor (5a) x successor.predecessor; (5b) if x (i, successor) then (5c) successor x; (5d) successor.Notify(i). (6) i.Notify(j): j believes it is predecessor of i (6a) if predecessor = or j (predecessor, i) then (6b) transfer keys in the range [j, i) to j; (6c) predecessor j. (7) i.F ix F ingers: executed periodically to update the finger table (7a) next f inger next f inger + 1; (7b) if next f inger > m then (7c) next f inger 1; (7d) f inger[next f inger] Locate Successor(i + 2next f inger1 ). (8) i.Check P redecessor: executed periodically to verify whether predecessor still exists (8a) if predecessor has failed then (8b) predecessor .
Here is the given code, implement the missing methods based on the description with complete output and file and ports you used.
Chord.java
import java.rmi.*; import java.rmi.registry.*; import java.rmi.server.*; import java.net.*; import java.util.*; import java.io.*; public class Chord extends java.rmi.server.UnicastRemoteObject implements ChordMessageInterface { public static final int M = 2; Registry registry; // rmi registry for lookup the remote objects. ChordMessageInterface successor; ChordMessageInterface predecessor; ChordMessageInterface[] finger; int nextFinger; int i; // GUID public ChordMessageInterface rmiChord(String ip, int port) { ChordMessageInterface chord = null; try{ Registry registry = LocateRegistry.getRegistry(ip, port); chord = (ChordMessageInterface)(registry.lookup("Chord")); return chord; } catch (RemoteException e) { e.printStackTrace(); } catch(NotBoundException e){ e.printStackTrace(); } return null; } public Boolean isKeyInSemiCloseInterval(int key, int key1, int key2) { if (key1 < key2) return (key > key1 && key <= key2); else return (key > key2 || key <= key1); }
public Boolean isKeyInOpenInterval(int key, int key1, int key2) { if (key1 < key2) return (key > key1 && key < key2); else return (key > key2 || key < key1); } public void put(int guid, byte[] data) throws RemoteException { } public byte[] get(int guid) throws RemoteException { return null; } public void delete(int guid) throws RemoteException { } public int getId() throws RemoteException { return i; } public boolean isAlive() throws RemoteException { return true; } public ChordMessageInterface getPredecessor() throws RemoteException { return predecessor; } public ChordMessageInterface locateSuccessor(int key) throws RemoteException { if (key == i) throw new IllegalArgumentException("Key must be distinct that " + i); if (successor.getId() != i) { if (isKeyInSemiCloseInterval(key, i, successor.getId())) return successor; ChordMessageInterface j = closestPrecedingNode(key); if (j == null) return null; return j.locateSuccessor(key); } return successor; } public ChordMessageInterface closestPrecedingNode(int key) throws RemoteException {
} public void joinRing(String ip, int port) throws RemoteException { } public void stabilize() { } public void notify(ChordMessageInterface j) throws RemoteException { } public void fixFingers() {
} public void checkPredecessor() { } public Chord(final int port) throws RemoteException { int j; finger = new ChordMessageInterface[M]; for (j=0;j ChordMessageInterface.java import java.rmi.*; import java.io.*; public interface ChordMessageInterface extends Remote { public ChordMessageInterface getPredecessor() throws RemoteException; ChordMessageInterface locateSuccessor(int key) throws RemoteException; ChordMessageInterface closestPrecedingNode(int key) throws RemoteException; public void joinRing(String Ip, int port) throws RemoteException; public void notify(ChordMessageInterface j) throws RemoteException; public boolean isAlive() throws RemoteException; public int getId() throws RemoteException; public void put(int guid, byte[] data) throws IOException, RemoteException; public byte[] get(int id) throws IOException, RemoteException; public void delete(int id) throws IOException, RemoteException; } ChordUser.java import java.rmi.*; import java.net.*; import java.util.*; import java.io.*; public class ChordUser { int port; public ChordUser(int p) { port = p; Timer timer1 = new Timer(); timer1.scheduleAtFixedRate(new TimerTask() { @Override public void run() { try { Chord chord = new Chord(port); System.out.println("Usage: \tjoin
Step by Step Solution
There are 3 Steps involved in it
Step: 1
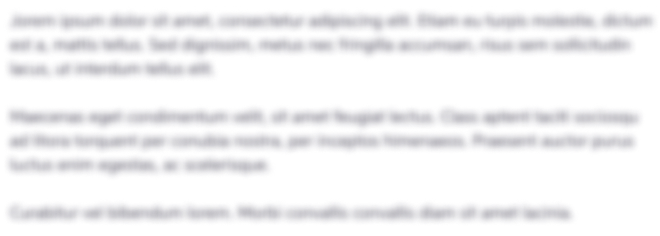
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started