Question
Java Please! Create a new project called StacksCafe and do all of your work in that project. In this exercise, you are going to practice
Java Please!
Create a new project called StacksCafe and do all of your work in that project.
In this exercise, you are going to practice working with stacks and queues to implement some functionality in Stacks Caf, where you get what we give you! In Stacks Caf, there are 4 spring-loaded food servers (see Figure 1). Each time an item is removed from a food server, the spring pushes up to reveal the next item. In this exercise, you are going to create some classes to hold various types of food or drink and then you are going to create data structures to represent the food servers. You are then going to create a series of orders and fill them using items from the food servers. Finally, you are going to check out the orders, which will involve printing off the items on the order and listing the total cost.
1. (2 points) Create an Abstract LunchItem class.
a. Create the class, indicating in the class declaration that it is abstract.
b. Create private class variables for the attributes shown in the UML diagram in Figure 1.
c. Create public getters (i.e., get methods) for the 3 private class variables you created in step 1b.
d. Create a constructor that takes 3 parameters (i.e., one for each of the private class variables). In the body of the constructor, just set the value of the private class variables to the parameters passed in.
2. (2 points) Create a FoodItem class that extends the abstract LunchItem class.
a. Add one private class variable as shown in the UML diagram in Figure 2.
b. Create a public getter for the private class variable you added in the previous step.
c. Create a constructor that takes in the same 3 parameters you setup for the LunchItem class in step 1b, as well as the parameter for the private class variable you created in step 2a (i.e., create a constructor that takes in 4 parameters).
d. The very first call in your constructor should be a call to the LunchItem constructor, passing in the 3 appropriate parameters. You can do this using the keyword super. If you are unsure how to do this, Google it! J
e. Override the toString method create a String describing a food item. Format it to look like this: slice of pizza (350 calories) - $3.75. To help with the currency formatting, look at the NumberFormat class. It will have methods that will help you format a double as currency.
3. (2 points) Create a DrinkItem class that extends the abstract LunchItem class.
a. Add one private class variable as shown in the UML diagram in Figure 2.
b. Create a public getter for the private class variable you added in the previous step.
c. Create a constructor that takes in the same 3 parameters you setup for the LunchItem class in step 1b, as well as the parameter for the private class variable you created in step 3a (i.e., create a constructor that takes in 4 parameters).
d. The very first call in your constructor should be a call to the LunchItem constructor, passing in the 3 appropriate parameters. You can do this using the keyword super. If you are unsure how to do this, Google it! J
e. Override the toString method create a String describing a food item. Format it to look like this: 20 ounces of Pepsi (150 calories) - $1.25. To help with the currency formatting, look at the NumberFormat class. It will have methods that will help you format a double as currency.
4. (4 points) Create an Order class that extends ArrayList
a. Make sure your Order class extends ArrayList
b. Add one private class variable as shown in the UML diagram in Figure 2.
c. Add a public getter for the private class variable you added in the previous step.
d. Create a constructor that takes in one parameter (corresponding to the class variable you defined in step 4b.). All you need to do is set this private variable to the parameter passed in.
e. Create a calculateTotalCost method that loops through the LunchItems being stored and totals up the cost.
f. Override the toString method so it producs output that is formatted like this:
Order #1
quarter-pound of cheeseburger(450) - $3.25
side of salad(120) - $2.15
piece of chocolate cake(500) - $2.95
16.0 ounces of Diet Pepsi(10) - $1.50
Total: $9.85
5. (8 points) Create the StacksCafe class based on the UML diagram shown in Figure 2.
a. Create the private class variables shown in the UML diagram in Figure 2 (i.e., 4 Stack variables and one Queue variable). These private variables should be initialized to null.
b. Create a getter for the meats Stack variable. Use lazy initialization to create the Stack only when it is first created. In the body of the getter method, make up 10 food items that are meats you might serve at a caf, such as hamburger, pizza, spaghetti, etc. For each of these food items, provide a name, cost, number of calories, and a unit (i.e., slice, piece, plate, etc.). Add these 10 food items to the meats Stack.
c. Create a getter for the vegetables Stack variable. Use lazy initialization to create the Stack only when it is first created. In the body of the getter method, make up 6 food items that are vegetables you might serve at a caf, such as french fries, salad, broccoli, etc. For each of these food items, provide a name, cost, number of calories, and a unit (i.e., slice, piece, plate, etc.). Add these 6 food items to the vegetables Stack.
d. Create a getter for the desserts Stack variable. Use lazy initialization to create the Stack only when it is first created. In the body of the getter method, make up 5 food items that are desserts you might serve at a caf, such as cake, ice cream, pie, etc. For each of these food items, provide a name, cost, number of calories, and a unit (i.e., slice, piece, plate, etc.). Add these 5 food items to the desserts Stack.
e. Create a getter for the drinks Stack variable. Use lazy initialization to create the Stack only when it is first created. In the body of the getter method, make up 10 drink items that you might serve at a caf, such as Pepsi, Diet Pepsi, coffee, tea, water, etc. For each of these drink items, provide a name, cost, number of calories, and a size (in ounces). Add these 10 drink items to the drinks Stack.
f. Create the getter for the Orders Queue. Since Queue is an interface, you will need to use a concrete class. Use a LinkedList for this purpose (i.e., define Orders to be a LinkedList). In the body of this method, create 10 empty orders (i.e., just create orders, each with an order number (1, 2, 3, etc.) and add them to the queue.
g. Create the fillOrders method. In this method, each order in the Queue is filled with LunchItems from the 4 stacks (meats, vegetables, desserts, and drinks). Note that some of the LunchItems will run out before others.
h. Create a checkOutOrders method that basically just prints out all of the orders in the queue.
6. (2 points) Test things out by adding a main method to the StacksCafe class.
a. Create a main method in the StacksCafe class. All you need to do in this method is create an instance of StacksCafe and then call the fillOrders method and then the checkoutOrders method.
Make sure the code runs and produce s the expected output
Step by Step Solution
There are 3 Steps involved in it
Step: 1
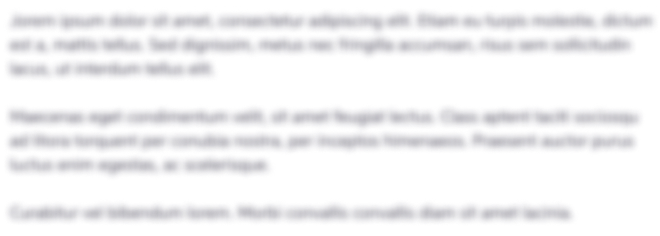
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started