Question
JAVA PLEASE HELP!! I need to implement two things: Write an abstract class AbstractGuessingGame that implements the ADT using a bag to hold the correct
JAVA PLEASE HELP!!
I need to implement two things: Write an abstract class AbstractGuessingGame that implements the ADT using a bag to hold the correct values.
Write two concrete classes to extend the abstract class: one using the array implementation of the BagWithCQS ADT and one using the linked-list implementation.
my code:
import java.util.Random; import java.util.Scanner; public class Guessing_Game { public static final int CONSTANT = 10; public static final int MAXGUESSES = 3; //randomly generate number to guess public static void main(String[] args) { Scanner console = new Scanner(System.in); Random rand = new Random(); int numGuesses = 0; int numTooHigh = 0; int numTooLow = 0; int number; int guess = -1; number = 1+rand.nextInt(CONSTANT);
System.out.println("I'm thinking of a number between 1 and " + CONSTANT);
//print message to enter a guess System.out.print("Your guess? "); guess = console.nextInt(); numGuesses++;
//read in guess of user, print whether guess it too high or two low. while (guess != number && numGuesses <= MAXGUESSES) { if (guess < number) { System.out.println("Your guess is too low."); numTooLow++; } else if (guess > number) { System.out.println("Your guess is too high."); numTooHigh++; } if(numGuesses < MAXGUESSES){ System.out.println("Number of guesses remaining: " + (MAXGUESSES - numGuesses)); //print for user to try again if enters wrong answer. System.out.println("Try again!"); guess = console.nextInt(); } numGuesses++; }
if (numGuesses == 1) System.out.println("Congratulations! You got it only 1 guess!"); else if(numGuesses <= MAXGUESSES){
//print message saying your guess is right System.out.println("Congratulations! You got it in " + numGuesses + " guesses"); System.out.println(numTooHigh + " of your guesses were too high."); System.out.println(numTooLow+ " of your guesses were too low."); } else{ System.out.println("You lost, My number is " + number); } } }
REFERENCE BELOW BAGWithCQS:
/**
BagCQS.java defines a parameterized interface Bag for the Bag Abstract Data Type that conforms (with one exception) to the principle of Command-Query-Separation
The Bag ADT is an unordered collection that allows for multiple occurrences of any item.
This interface is closely modeled on Frank Carrano's Bag interface in "Data Structures and Abstractions in Java" (3rd Edition)
Invariants:
if (isEmpty() == true) then getCount() == 0 else getCount() > 0
if (contains(item) == true) then getFrequencyOf(item) > 0 else getFrequencyOf(item) == 0
Creation constraints:
The constructor for any class the implements the Bag ADT must create an initially empty bag. So, immediately afterer contraction, isEmpty() == true AND getCount() == 0
*/
interface BagWithCQS { /** * Adds the specified item to the bag *
* pre-condition: NOT isFull() *
* post-condition: * getFrequencyOf(item) == * getFrequencyOf(item)@pre + 1 * AND * getCount() == getCount()@pre + 1 *
* @param item -- the item to be added to the bag * */ public void add(T item); /** * Returns the total number of items in the bag. * * @return number of items in the bag (counting duplicates) */ public int getCount(); /** Removes all items from the bag
pre-condition: none (true)
post-condition: getCount() == 0 */ public void clear(); /** * Removes one occurrence of the specified item from the * bag, if there is one *
* pre-condition: none(true) * * post-condition: iff (item is in bag)@pre * getCount() == get Count)@pre-1 * getFrequencyOf(item) == getFrequencyof(item)@pre-1 * AND result=true, * else result == false * getCount() == getCount()@pre * AND getFrequency() == getFrequency@pre
* @param item - the item to be removed */ public void remove(T item); /** * Removes an occurrence of an unspecified item from the * bag and returns it. Returns null if the bag is empty *
* pre-condition: None * * post-condition: * IF(isEmpty()@pre == false) * THEN * bag@pre*exists(removedItem:T|frequencyOf(removedItem) * ==frequencyOf(removedItem)@pre-1) * AND * getCount() == getCount()@pre-1) * AND * result == removedItem * ELSE * getCount() == getCount()@pre * AND * result == null * * @return removed item - note that this is a (minor) * violation of command query separation */ public T remove(); /** Checks whether the bag is empty
@return if getCount() == 0 true else false */ public boolean isEmpty(); /** Checks whether the bag is full (i.e., no item can be added), false otherwise
@return if bag is full then true else false */ public boolean isFull(); /** Checks whether the bag contains an occurrence of the specified item
@return if getFrequencyOf(item) > 0 then true else false */ public boolean contains (T item); /** Returns the number of occurrences of the specified item in the bag
@return the number of occurrences of item in the bag */ public int getFrequencyOf(T item); /** Returns an array of length getCount() containing the items in the bag. */ public T[] toArray(); } // interface Bag
Step by Step Solution
There are 3 Steps involved in it
Step: 1
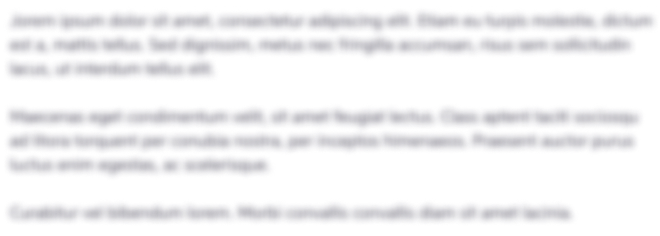
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started