Question
Java: Please help me modify the following code according to the instructions. Thank you. Instructions: User Class Subclass of Account class. Instance variables username String
Java: Please help me modify the following code according to the instructions. Thank you.
Instructions:
- User Class
- Subclass of Account class.
- Instance variables
- username String
- fullName String
- deptCode int representing the department code
- No other instance variables are needed.
- Methods
- Default constructor sets all instance variables to a default value
- Call the super class constructor passing appropriate parameters to it.
- Set the rest of the variables
- Parameterized constructors
- Call the super class constructor passing appropriate parameters to it.
- Set the rest of the variables to the remaining parameters
- Accessor and mutator methods for all variables in this class
- toString
- Calls super class methods as needed.
- Returns a nicely formatted String representing the user to include fullName, username, deptCode, accountID number, clearPassword, encryptPassword and key
- Default constructor sets all instance variables to a default value
Code:
public class User extends Account { private String username; private String fullName; private int deptCode;
/** * Constructor to initialize the variables. * */ public User() { super("", ""); setUsername(""); setFullName(""); setDeptCode(0); }
/** * Constructor to set the variables to the input information. */ public User(String newUsername, String newFullName, int newDeptCode, String newClearPassword, String newKey) { super(newClearPassword, newKey); setUsername(newUsername); setFullName(newFullName); setDeptCode(newDeptCode); }
/** * Method to return the user's username. */ public String getUsername() { return username; }
/** * Method to set the username */ public void setUsername(String newUsername) { username = newUsername; }
/** * Method to return the user's full name. */ public String getFullName() { return fullName; }
/** * Method to set the user's full name */ public void setFullName(String newFullName) { fullName = newFullName; }
/** * Method to return the department code */ public int getDeptCode() { return deptCode; }
/** * Method to set the department code. * */ public void setDeptCode(int newDeptCode) { deptCode = newDeptCode; }
/** * Returns the information for the user. */ public String toString() { String info; info = String.format("%-5s %s %d ", getUsername(), getFullName(), getDeptCode()); info += super.toString(); return info; //(getUsername() + " " + getFullName() + " " + getDeptCode() + "" + super.toString()); }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
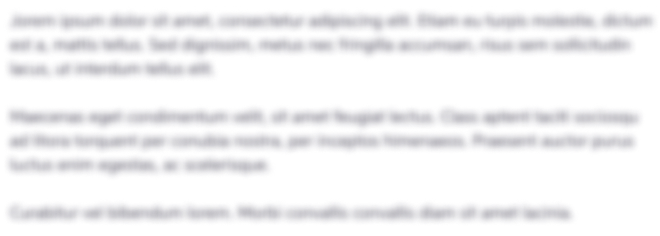
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started