Question
[JAVA] Please help me with writing methods for this assignment. The code I have that needs to be modified is provided below. Thanks. The questions:
[JAVA] Please help me with writing methods for this assignment. The code I have that needs to be modified is provided below. Thanks.
The questions:
a.) Write a recursive method for converting a string of digits into the integer it represents. For example, "12341" represents the integer 12341. Hint: Process the string left to right.
b.) Write a recursive method that counts the number of nodes in a singly linked list.
c.) Write a recursive method that finds the smallest integer value in an array of int.
d.) Write a resursive method that determines if a string is a palindrome, that is, it is equal to its reverse. For example, "racecar" is a palindrome. Hint: Be careful to return the correct value for both odd - and even-length strings.
e.) Write a recursive method that takes a character string s and returns its reverse. So for example, the reverse of "pots&pans" would be "snap&stop". Hint: Swap the first and last characters.
f,) Write a recursive method that displays the directories on your computer - example J:\
g.) Modify the "public static int sum" method that calculates the sum of integers between 1 and N. Have the new version match the following recursive definition: The sum of 1 to N is the sum of 1 to (N/2) plus the sum of (N/2 + 1) to N. Trace your solution using an N of 7.
h.) Write a recursive defintion of x^y (x to the power of y), where x and y are integers and y >= 0. In addition, write the recursive method.
i.) Write a recursive method to display the contents (data) of a linked-list in reverse order.
j.) Write a recursive method to convert a number, n, to a base b, and return result as a String.
Here are the questions again with the snippets of code provided for each that need to be written with a recursive method...
package lab8; import java.io.File; public class Lab8App { public static void main(String[] args) { Lab8App recursion = new Lab8App(); System.out.println(recursion.strToNum("12341")); System.out.println(recursion.findMin(new int[] {3,2,1,4,5}, 5, 0)); System.out.println(recursion.isPalindrome("racecar", 0, 6)); System.out.println(recursion.reverseString("pots&pans")); recursion.traverse(new File("J:/")); recursion.hanoi(4); LinkedList ulist = new LinkedList(); String[] str = {"hello","this","is","a","test"}; for(String s : str) ulist.insert(s); System.out.println(ulist); Node node = ulist.getfront(); System.out.println(recursion.countNodes(node)); } public int strToNum(String str) { if(str.length() = 0. * In addition, write the recursive method. */ /* * 4. Write a recursive method to display the contents of a linked-list in reverse order. */ /* * 5. Write a recursive method to convert a number, n, to a base, b, and return result as a String. */ } class Node{ private E data; private Node next; public Node(){ data = null; next = null; } public Node(E d){ data = d; next = null; } public Node(E d, Node n){ data = d; next = n; } public Node getNext(){ return next; } public void setNext(Node n){ next = n; } public E getData() { return data; } public void setData(E data) { this.data = data; } } class LinkedList>{ protected Node head = new Node(); // dummy Node protected int numItems; public Node getfront(){ return head.getNext(); } public int getSize(){ return numItems; } public boolean isEmpty(){ return numItems == 0; } public void insert(T insertItem) { if(insertItem == null) throw new NullPointerException(); Node trav = head; while(trav.getNext() != null) trav = trav.getNext(); trav.setNext(new Node(insertItem)); ++numItems; } public String toString(){ String str = " ================================== " + "The list contains " + numItems + " items. " + "================================== ["; Node trav = head.getNext(); while(trav != null){ // str += trav.data + " "; str += trav.getData() + ((trav.getNext() == null) ? "" : "->"); trav = trav.getNext(); } return str + "]"; } }
package lab8; public class Factorial { public static void main(String[] args) { int n = 20; int factorial = 1; int i = 1; while (i Write a recursive method for converting a string of digits into the integer it represents. For example, "12341 represents the integer 12341. Hint: Process the string left to right. public int strToNum (String str) ( if(str.length)1) return 0 else return ((str.charAt (str.length() - 1)-0') + (10 * strToNum(str.substring (0, str.length) - 1)))); Write a recursive method that counts the number of nodes in a singly linked list. private int countNodes (Nodetrav) if (trav null) return 0 return 1 countNodes (trav.next); Write a recursive method for converting a string of digits into the integer it represents. For example, "12341 represents the integer 12341. Hint: Process the string left to right. public int strToNum (String str) ( if(str.length)1) return 0 else return ((str.charAt (str.length() - 1)-0') + (10 * strToNum(str.substring (0, str.length) - 1)))); Write a recursive method that counts the number of nodes in a singly linked list. private int countNodes (Node trav) if (trav null) return 0 return 1 countNodes (trav.next)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
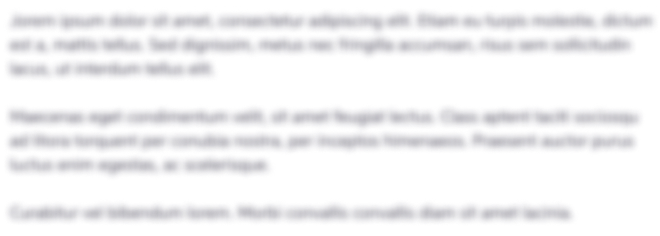
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started