Question
Java: Please help with my following code and tester. Thank you. Instructions for code: Activity - Implement a program prompts a user for their name,
Java: Please help with my following code and tester. Thank you.
Instructions for code:
Activity - Implement a program prompts a user for their name, age, salary, hackername and email address.
- Catches the following exceptions
- NumberFormatException for the salary
- User defined Malformed email address (See below)
- Allows the user to try again in either case.
- User Class
- Stores user information in instance variables name, age, salary, hacker name and email address in the appropriate data type.
- Default constructors only
- Accessor and mutator methods including:
- boolean setSalary(String newSalary)
- receives a string and converts it to a number
- Handles the NumberFormatException.
- Returns true if conversion is successful and false if not.
- boolean setEmail(String newEmail)
- receives a String
- Tests for Malformed email address and throws MalformedEmailAddress Exception if it is malformed.
- Returns true if address is correctly formed, false if not.
- boolean setSalary(String newSalary)
- setHackerName method
- Use your creativity to create an algorithm that uses the data the create a hacker nickname,
- The nickname does not necessary have to be a pronounceable word, but that would be nice.
- You may want to store a bank of words to use as part of your hacker name see http://forums.windrivers.com/showthread.php?38547-Good-hacker-names for some ideas.
- toString method - Displays all the instance variable data in a neatly formated output
-
- Exception handling
- Email Address
- Check to see if an email address is valid
- Valid address is simply contains an @ symbol between the first and last characters of the address.
- If email address is malformed it throws a the User Defined MalformedEmailAddress Exception.(see below)
- The MalformedEmailAddress should be caught and the user given a chance to correct the address. (Think about this as its a little trickier than you think)
- Email Address
- Exception handling
- MalformedEmailAddress Exception class - Creates an error message explaining the exception.
- UserTest
- Test all methods in the User class including the exceptions.
- To simplify the process read all data in from a Scanner as a String.
Code:
import java.net.MalformedURLException; import java.util.Random; /** * @author Admin * */ public class User { /** * instance variable age */ private int age; /** * Instance variable salary */ private int salary; /** * instance variable hackerName */ private String hackerName; /** * Instance variable emailAddress */ private String emailAddress; /** * Default constructors only */ public User() { this.age = 0; this.salary = 0; this.hackerName = " "; this.emailAddress = " "; } /** Methods */ /** * Mutator * @param age */ public void setAge(int age) { this.age = age; } /** * Accessor * @return age */ public int getAge() { return age; } /** * Mutator * boolean setSalary(String newSalary) * Receives a string and converts it to a number. * Handles the NumberFormatException. * Returns true if conversion is successful and false if not. * @param newSalary * @return */ public boolean setSalary(String newSalary) { try { this.salary = Integer.parseInt(newSalary); } catch (NumberFormatException ex) { System.out.println("please enter in numericals"); return false; } return true; } /** * Accessor * @return salary */ public int getSalary() { return salary; }
/** * Mutator * boolean setEmail(String newEmail) * Receives a String. * Tests for Malformed email address and throws * MalformedEmailAddress Exception if it is malformed. * Returns true if address is correctly formed, false if not. */ public boolean setEmail(String newEmail) { int count = 0; for (int i = 0; i < newEmail.length(); i++) { if (newEmail.charAt(i) == '@') { count++; } } if (count > 1) { try { throw new MalformedURLException(); } catch (MalformedURLException e) { return false; } } return true; } /** * Accessor * @return email */ public String getEmail() { return emailAddress; } /** * Mutator * setHackerName method: Use your creativity to create an algorithm * that uses the data the create a hacker nickname. * The nickname does not necessary have to be a pronounceable word, * but that would be nice. * You may want to store a bank of words to use as part of your * hacker name * @param hackerName * @return */ public String setHackerName(String hackerName) { String[] Beginning = { "Kr", "Ca", "Ra", "Mrok", "Cru", "Ray", "Bre", "Zed", "Drak", "Mor", "Jag", "Mer", "Jar", "Mjol", "Zork", "Mad", "Cry", "Zur", "Creo", "Azak", "Azur", "Rei", "Cro", "Mar", "Luk" }; String[] Middle = { "air", "ir", "mi", "sor", "mee", "clo", "red", "cra", "ark", "arc", "miri", "lori", "cres", "mur", "zer", "marac", "zoir", "slamar", "salmar", "urak" }; String[] End = { "d", "ed", "ark", "arc", "es", "er", "der", "tron", "med", "ure", "zur", "cred", "mur" }; Random rand = new Random(); return Beginning[rand.nextInt(Beginning.length)] + Middle[rand.nextInt(Middle.length)] + End[rand.nextInt(End.length)]; } /** * Accessor * @return hackerName */ public String getHackerName() { return hackerName; } /** * toString method - Displays all the instance variable * data in a neatly formated output. */ @Override public String toString() { return "User [salary=" + salary + ", age=" + age + ", hackerName=" + hackerName + ", newEmail=" + emailAddress + "]"; } @Override public int hashCode() { final int prime = 31; int result = 1; result = prime * result + age; result = prime * result + ((emailAddress == null) ? 0 : emailAddress.hashCode()); result = prime * result + ((hackerName == null) ? 0 : hackerName.hashCode()); result = prime * result + salary; return result; } @Override public boolean equals(Object obj) { if (this == obj) return true; if (obj == null) return false; if (getClass() != obj.getClass()) return false; User other = (User) obj; if (age != other.age) return false; if (emailAddress == null) { if (other.emailAddress != null) return false; } else if (!emailAddress.equals(other.emailAddress)) return false; if (hackerName == null) { if (other.hackerName != null) return false; } else if (!hackerName.equals(other.hackerName)) return false; if (salary != other.salary) return false; return true; }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
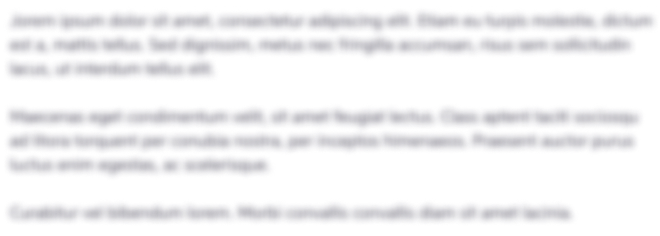
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started