Question
[JAVA] Please help with this program about how to use Iterators. I provided the assignment and code I have below. Thank you! package lab7; import
[JAVA] Please help with this program about how to use Iterators. I provided the assignment and code I have below. Thank you!
package lab7;
import list.OrderedList; import list.UnorderedList; import sort.Sort;
import java.util.Iterator; public class ListApp { public static void main(String[] args){ String[] str = {"hello","this","is","the","beginning"}; UnorderedList
System.out.println(ulist.search(str[4])); System.out.println(olist.search(str[4])); System.out.println(ulist.search("hello")); System.out.println(olist.search("hello")); System.out.println(ulist.search("Hello")); olist.remove("beginning"); ulist.remove("beginning"); System.out.println(olist); System.out.println(ulist); Integer[] x = {5, 4, 2, 6, 1, 7}; Sort.insertionSort(x); for(int n : x) System.out.println(n); Sort.insertionSort(str); for(String s : str) System.out.println(s); } }
------------------------------------
package list;
import jdk.jshell.spi.ExecutionControl;
import java.util.Iterator; import java.util.NoSuchElementException;
public abstract class List
protected class Node
class ListIterator protected Node @Override abstract public void add(T item); @Override public T first() throws ListEmptyException { // TODO Auto-generated method stub return null; } @Override public T last() throws ListEmptyException { // TODO Auto-generated method stub return null; } @Override public T removeFirst() throws ListEmptyException { // TODO Auto-generated method stub return null; } @Override public T removeLast() throws ListEmptyException { // TODO Auto-generated method stub return null; } @Override public T removeAtPosition(int location) throws IndexOutOfBoundsException { // TODO Auto-generated method stub return null; } @Override abstract public void remove(T item); @Override public void set(T item1, T item2) throws ListEmptyException { // TODO Auto-generated method stub } @Override public T set(T item, int location) throws IndexOutOfBoundsException { // TODO Auto-generated method stub return null; } @Override abstract public int search(T item); @Override public boolean contains(T item) throws ListEmptyException { if(isEmpty()) throw new ListEmptyException(); return search(item) != -1; } @Override public boolean isEmpty() { return size == 0; } @Override public int getSize(){ return size; } @Override public void clear() { size = 0; front.setNext(null); System.gc(); } @Override public Iterator public String toString(){ String str = "["; Iterator ----------------------------------- package list; import java.util.Iterator; public interface ListADT /** * Adds an item to the list * * @param item * the item to be added */ void add(T item); /** * Returns the first item in the list * * @return the first item * @throws ListEmptyException * if the list is empty */ T first() throws ListEmptyException; /** * Returns the last item in the list * * @return the last item * @throws ListEmptyException * if the list is empty */ T last() throws ListEmptyException; /** * Removes and returns the first item in the list * * @return the first item * @throws ListEmptyException * if the list is empty */ T removeFirst() throws ListEmptyException; /** * Removes and returns the last item in the list * * @return the last item * @throws ListEmptyException * if the list is empty */ T removeLast() throws ListEmptyException; /** * Removes and returns an item stored at a specified location in the list * * @param location * the location of the item to removed from the list * @return the item stored at that location * @throws IndexOutOfBoundsException * if location = size() */ T removeAtPosition(int location) throws IndexOutOfBoundsException; /** * Removes first occurrence of an item from the list * * @param item * the item to removed from the list * @throws ListEmptyException * if the list is empty */ void remove(T item) throws ListEmptyException; /** * Replaces all occurrences of the item stored in the list * * @param item1 * the old item * @param item2 * the new item * @throws ListEmptyException * if the list is empty */ void set(T item1, T item2) throws ListEmptyException; /** * Replaces the item stored at the given location and returns the old item * * @param item * the new item * @param location * the location * @return the old item * @throws IndexOutOfBoundsException * if location = size() */ T set(T item, int location) throws IndexOutOfBoundsException; /** * Searches for an item in the list and returns its location or -1 if the * item is not in the list * * @param item * the item to search for * @return the location of the item * @throws ListEmptyException * if the list is empty */ int search(T item) throws ListEmptyException; /** * Searches for an item in the list and returns true if it can be found * * @param item * the item to search for * @return true if an item is in the list or false otherwise * @throws ListEmptyException * if the list is empty */ boolean contains(T item) throws ListEmptyException; /** * Returns the number of items in the list * * @return the number of items in the list */ /** * Returns true if the list is empty, or false otherwise * * @return true if the list is empty, or false otherwise */ boolean isEmpty(); /** * Returns the number of items in the list * * @return the number of items in the list */ int getSize(); /** * Removes all of the items in this list. */ void clear(); /** * Returns an iterator over the items in this list in proper sequence. */ Iterator ----------------------------------------------------- package list; public class ListEmptyException extends Exception { public ListEmptyException() { super("List Empty Exception..."); } public ListEmptyException(String message) { super(message); } } ---------------------------------------------------- package list; public class OrderedList @Override public void add(T item) { Node @Override public void remove(T item) { boolean found = false, okay = true; Node ------------------------------------------------------ package list; public class UnorderedList @Override public void add(T item) { Node @Override public void remove(T item) { boolean found = false; Node @Override public int search(T item) { int index = 0; boolean found = false; Node } ------------------------------------------------------------- package sort; public class Sort{ public static
Step by Step Solution
There are 3 Steps involved in it
Step: 1
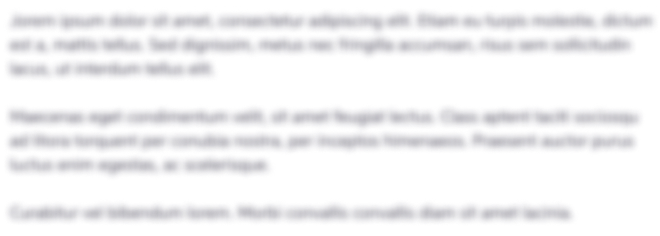
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started