Question
Java, please implement into SocialMediaProfile.java Remember to keep the **names of variables and methods the same as I have them below** for testing purposes!Remember to
Java, please implement into SocialMediaProfile.java
Remember to keep the **names of variables and methods the same as I have them below** for testing purposes!Remember to **create** your **program piece by piece** and **test it in main** before moving onto the next piece!Remember the **Homework Help Forum** if you need help!
**Task 1: Add the Required Variables**
**Task 2: update the constructor to initialize these required variables. You should not need to pass in any additional parameters to the constructor**
We will now add the ability for a social media profile to have a friendsList of other social media profiles (it will be more like following because Profile A can have Profile B as a friend but Profile B might not have Profile A as a friend)
We will accomplish this through a **Partially Filled Array** which is an important concept. Basically A profile might start out with no friends and then it might add a friend which means the friendsList, which will be a SocialMediaProfile Array, will have 1 useful value and the rest not useful. So if the array is of length 10, it will have 1 useful value, and 9 trash values. To keep track of this we will have to have a count variable and to keep track of the total friends we can add to a profile we will have to have a MAX_FRIENDS variable.
**Required Variables**You will add 3 variables to SocialMediaProfile: **friendsList**, **count**, and **MAX_FRIENDS**. friendsList and count will be private and MAX_FRIENDS will be public.
You will use your Social Media Profile you created in Assignment 1 and add a **friendsList** private variable which will be an array of all the SocialMediaProfiles that this person is "following"
You will also add a **count** variable that keeps track of the number of friends and is also the next place we insert into the friendsList. Note that **count** will start at 0 when the SocialMediaProfile is constructed
You will add a **MAX_FRIENDS** public static final variable that will be set to **3**. For now we will assume each social media profile has at maximum 3 friends.
Your **friendsList** should be an array of SocialMediaProfiles that is constructed to be the length of **MAX_FRIENDS** in the constructor
**Task 3: Add the Required methods Making Sure to test each aspect of each method in main before moving onto the next one**
**Required methods**You should update your SocialMediaProfile code with the **2** following methods
**String stringOfFriends**-> returns a string of the SocialMediaProfile person's name followed by the word friends and then each social Media Profile name who is a friend on the same line separted by a "-". The format of this must be exact. For example let's say a profile with the name Ashok has friends withe the names Sandy and Julie and Trevor. Calling stringOfFriends gives you the following string
> _Ashok Friends: Sandy-Julie-Trevor_
You should implement this first because it'll be used to test all your functions (i.e. you won't pass any tests unless this is working. To test it you can manually add students to the array inside the constructor, just remember to comment this code out later when you actually do the addFriend function.
**boolean addFriend(?)**->
Test 1: Adds a friend to the next array slot and updates the count variable and returns true.
Test 2: Duplicate Adds Only adds a friend if that friend hasn't already been added. If it is a duplicate being added this returns false
Test 3: Additionally to Test 2, we only add a friend if we're not at capacity. If we are at capacity this returns false. Note we want to add a reference to the socialMediaProfile (Shallow Copy) so that if the two SocialMediaProfiles, Profile C and Profile B, reference the same friend, Profile A, in their friend's list and that Profile A changes a value, that value change is reflected in the Profile C's and Profile B's SocialMediaProfiles
Test 3: Returns the correct add friend returns true if the friend was successfully added
Note if we were doing this for real want to update toString to invoke stringOfFriends, update equals to consider the equality of two socialMediaProfiles taking into consideration the friendsList, and allow a profile to have more than 3 friends. We will deal with this later on.
public class SocialMediaProfile {
private String name;
private int age;
private String country;
private int numberOfPosts;
private int numberOfLikes;
public SocialMediaProfile(String name, int age, String country, int numberOfPosts, int numberOfLikes)
{
this.name = name;
if(age >= 0 )
this.age = age;
else
this.age = 0;
this.country = country;
if(numberOfPosts >= 0)
this.numberOfPosts = numberOfPosts;
else
this.numberOfPosts = 0;
if(numberOfLikes >= 0)
this.numberOfLikes = numberOfLikes;
else
this.numberOfLikes = 0;
}
public void setname(String name)
{
this.name = name;
}
public void setage(int age)
{
if(age >= 0 )
this.age = age;
}
public void setcountry(String country)
{
this.country = country;
}
public void setnumberOfPosts(int numberOfPosts)
{
if(numberOfPosts >= 0 )
this.numberOfPosts = numberOfPosts;
}
public void setnumberOfLikes(int numberOfLikes)
{
if(numberOfLikes >= 0)
this.numberOfLikes = numberOfLikes;
}
public String getname()
{
return name;
}
public int getage()
{
return age;
}
public String getcountry()
{
return country;
}
public int getnumberOfPosts()
{
return numberOfPosts;
}
public int getnumberOfLikes()
{
return numberOfLikes;
}
public double averageLikesPerPost()
{
if(numberOfPosts == 0)
return 0;
else
return ((double)numberOfLikes)/numberOfPosts;
}
@Override
public boolean equals(Object obj)
{
if(obj instanceof SocialMediaProfile)
{
SocialMediaProfile s = (SocialMediaProfile)obj;
return s.name.equals(name) && s.age == age && s.country.equals(country) && s.numberOfLikes == numberOfLikes && s.numberOfPosts == numberOfPosts;
}
return false;
}
public String toString()
{
return "name:"+name+", age:"+age+", country:"+country+", numberOfPosts:"+numberOfPosts
+", numberOfLikes:"+numberOfLikes+", averageLikesPerPost:"+averageLikesPerPost();
return toReturn;
}
}
here is the main(please do not change)
public class Main {
public static void main(String[] args) {
System.out.println("Hello world!");
//*******UNCOMMENT THE FOLLOWING LINE AND THE TEST CASES BELOW WHEN YOU ARE READY TO TEST******//
// org.junit.runner.JUnitCore.main("Main");
}
//***UNCOMMENT THESE TESTS WHEN YOU ARE READY TO TEST!****//
/*
@Test
public void ConstructorAndPrintEmptyStringOfFriendsTest() {
// Failure message:
// This test creates a social media profile with the following information
// "Ashok" 35 "USA" 15, 3
// then prints out the FriendsList which should be empty!
SocialMediaProfile myProfile= new SocialMediaProfile("Ashok",35, "USA", 15,3);
assertTrue(myProfile.stringOfFriends().equals("Ashok Friends:") || myProfile.stringOfFriends().equals("Ashok Friends: "));
}
@Test
public void addOneFriend() {
// Failure message:
// This test creates a social media profile with the following information
// "Ashok" 35 "USA" 15, 3
// then adds a single friend "Tony" 56 "China" 33, 9
// The add should return true and when the stringOfFriends is printed should print out Ashok Friends: Tony
SocialMediaProfile myProfile= new SocialMediaProfile("Ashok",35, "USA", 15,3);
SocialMediaProfile tonyProfile= new SocialMediaProfile("Tony",56, "China", 33,9);
assertEquals(myProfile.addFriend(tonyProfile),true);
assertEquals(myProfile.stringOfFriends(),"Ashok Friends: Tony");
}
@Test
public void addThreeFriends() {
// Failure message:
// This test creates a social media profile with the following information
// "Ashok" 35 "USA" 15, 3
// then adds three friends in the following order
// "Tony" 56 "China" 33, 9
// "Sandry" 66 "Brazil", 66,20
// //"Minho" 77 "Austrailia", 120,66
// The add should return true and when the stringOfFriends is printed should print out Ashok Friends: Tony-Sandry-Minho
SocialMediaProfile myProfile= new SocialMediaProfile("Ashok",35, "USA", 15,3);
SocialMediaProfile tonyProfile= new SocialMediaProfile("Tony",56, "China", 33,9);
SocialMediaProfile sandryProfile= new SocialMediaProfile("Sandry", 66, "Brazil", 66,20);
SocialMediaProfile minhoProfile= new SocialMediaProfile("Minho", 77, "Austrailia", 120,66);
assertEquals(myProfile.addFriend(tonyProfile),true);
assertEquals(myProfile.addFriend(sandryProfile),true);
assertEquals(myProfile.addFriend(minhoProfile),true);
assertEquals(myProfile.stringOfFriends(),"Ashok Friends: Tony-Sandry-Minho");
}
@Test
public void addDuplicateFriend() {
// Failure message:
// This test creates a social media profile with the following information
// "Ashok" 35 "USA" 15, 3
// then adds a single friend "Tony" 56 "China" 33, 9
// The attempts to add the friend again, the function should return false and when the stringOfFriends is printed should print out
// Ashok Friends: Tony
SocialMediaProfile myProfile= new SocialMediaProfile("Ashok",35, "USA", 15,3);
SocialMediaProfile tonyProfile= new SocialMediaProfile("Tony",56, "China", 33,9);
assertEquals(myProfile.addFriend(tonyProfile),true);
assertEquals(myProfile.addFriend(tonyProfile),false);
assertEquals(myProfile.stringOfFriends(),"Ashok Friends: Tony");
}
@Test
public void addFourFriendsTest() {
// Failure message:
// adds 4 friends, only 3 should be added because we have set MAX_FRIENDS to 3
// This test creates a social media profile with the following information
// "Ashok" 35 "USA" 15, 3
// then adds three friends in the following order
// "Tony" 56 "China" 33, 9
// "Sandry" 66 "Brazil", 66,20
// //"Minho" 77 "Austrailia", 120,66
// then adds a fourth friend, this should not add and should return false
// "Jamie", 44, "South Africa", 233, 44
// The add should return true and when the stringOfFriends is printed should print out Ashok Friends: Tony-Sandry-Minho
SocialMediaProfile myProfile= new SocialMediaProfile("Ashok",35, "USA", 15,3);
SocialMediaProfile tonyProfile= new SocialMediaProfile("Tony",56, "China", 33,9);
SocialMediaProfile sandryProfile= new SocialMediaProfile("Sandry", 66, "Brazil", 66,20);
SocialMediaProfile minhoProfile= new SocialMediaProfile("Minho", 77, "Austrailia", 120,66);
SocialMediaProfile jamieProfile= new SocialMediaProfile("Jamie", 44, "South Africa", 233,44);
assertEquals(myProfile.addFriend(tonyProfile),true);
assertEquals(myProfile.addFriend(sandryProfile),true);
assertEquals(myProfile.addFriend(minhoProfile),true);
assertEquals(myProfile.addFriend(jamieProfile),false);
assertEquals(myProfile.stringOfFriends(),"Ashok Friends: Tony-Sandry-Minho");
}
*/
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
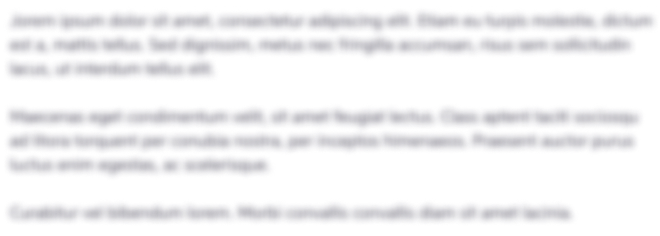
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started