Question
JAVA problem In this exercise, you will create a simple spell checker program. Your program will have the following characteristics: Your program will be capable
JAVA problem
In this exercise, you will create a simple spell checker program. Your program will have the following characteristics:
Your program will be capable of reading multiple files to be spell checked from command line arguments.
When your program starts up, you will read in the dictionary.txt file which contains a list of known words. Only read in this file once. Store these words in a HashSet data structure.
Create a TreeSet to store any miss-spelled words that you find.
For each file in the command line parameter list, print out the name of the file before reading in any words.
For each file parse out each of the words in the file and check to see if the word is in the HashSet you created with the dictionary.txt words or its already in your list of miss-spelled words.
If the word is in the dictionary HashSet or the miss-spelled word TreeSet then proceed to the next word.
If the word is not in the dictionary or the miss-spelled word list, give your user 2 choices: Add the word to the HashSet dictionary, or add the word to the list of miss-spelled words.
At the end of each file processed, dump out the list of miss-spelled words.
When you then start to read in the next file, make sure you clear the list of miss-spelled words. Keep any words added to the dictionary HashSet.
More details:
In parsing the words in these text files, you will need to filter out punctuation characters. I used the StringTokenizer, and I called the following constructor: StringTokenizer st = new StringTokenizer(line, " \t,.;:-%'\"");
before checking the word against the dictionary, make sure you lower case the word
if the "word" doesn't start out with a letter (i.e. >= 'a' And <= 'z'), then skip it because it is likely a number
If the word couldn't be found in either list and ends with an 's', try removing the 's' to create a singular version of the word and try again to find the word in your dictionary or miss-spelled word list using the singular version of the word.
Only print out lines that contain a word needing a user response. See the Sample output below from the published answer.
Use the following dictionary file
dictionary.txt
You can find the input files to test from the following links:
Russia.txt
Ukraine.txt
Your command line arguments will be:
Russia.txt Ukraine.txt
When you execute your program to publish your output, answer "n" to the question of whether to add the word to the dictionary for all words except the for the word "christianity" .
See the sample output below:
Some of the output from running this program should look something like:
First part of the output from running the published answer
For those of you who like to see a template of the published answer, here it is:
public class SpellCheck { // We could hava also used TreeSet for the dictionary private HashSetdictionary = new HashSet (); private TreeSet miss_spelled_words = new TreeSet (); public SpellCheck() throws FileNotFoundException { // Add all of the words from "dictionary.txt" to the dictionary HashSet } public void checkSpelling(String fileName) throws FileNotFoundException { System.out.println("======== Spell checking " + fileName + " ========="); // Clear miss_spelled_words miss_spelled_words.clear(); // Read in each line from "fileName" // For each line, break the line into words using the following StringTokenizer StringTokenizer st = new StringTokenizer(line, " \t,.;:-%'\""); // lower case each word obtained from the StringTokenizer // skip word if the first character is not a letter // Skip over word if it can be found in either dictionary, or miss_spelled_words // If word ends with 's', then try the singular version of the word in the dictionary and miss_spelled_words ... skip if found // Print line containing miss-spelled word(make sure you only print it once if multiple miss-spelled words are found on this line) // Ask the user if he wants the word added to the dictionary or the miss-spelled words and add word as specified by the user } public void dump_miss_spelled_words() { // Print out the miss-spelled words } public static void main(String[] args) { try { SpellCheck spellCheck = new SpellCheck(); for (int i=0; i < args.length; i++) { spellCheck.checkSpelling(args[i]); spellCheck.dump_miss_spelled_words(); } } catch (FileNotFoundException e) { System.out.println(e); } } }
The text file for Russia.txt is below
"
The nation's history began with that of the East Slavs, who emerged as a recognizable group in Europe between the 3rd and 8th centuries AD. Founded and ruled by a Varangian warrior elite and their descendants, the medieval state of Rus arose in the 9th century. In 988 it adopted Orthodox Christianity from the Byzantine Empire, beginning the synthesis of Byzantine and Slavic cultures that defined Russian culture for the next millennium. Rus ultimately disintegrated into a number of smaller states; most of the Rus lands were overrun by the Mongol invasion and became tributaries of the nomadic Golden Horde. The Grand Duchy of Moscow gradually reunified the surrounding Russian principalities, achieved independence from the Golden Horde, and came to dominate the cultural and political legacy of Kievan Rus. By the 18th century, the nation had greatly expanded through conquest, annexation, and exploration to become the Russian Empire, which was the third largest empire in history, stretching from Poland in Europe to Alaska in North America. Following the Russian Revolution, the Russian Soviet Federative Socialist Republic became the largest and leading constituent of the Soviet Union, the world's first constitutionally socialist state and a recognized superpower, which played a decisive role in the Allied victory in World War II. The Soviet era saw some of the most significant technological achievements of the 20th century, including the world's first spacecraft, and the first astronaut. Following the dissolution of the Soviet Union in 1991, the Russian SFSR reconstituted itself as the Russian Federation and is recognized as the continuing legal personality of the Union state. The Russian economy ranks as the eighth largest by nominal GDP and sixth largest by purchasing power parity. Russia's extensive mineral and energy resources, the largest reserves in the world, have made it one of the largest producers of oil and natural gas globally. The country is one of the five recognized nuclear weapons states and possesses the largest stockpile of weapons of mass destruction. Russia is a great power and a permanent member of the United Nations Security Council, a member of the G8, G20, the Council of Europe, the Asia-Pacific Economic Cooperation, the Shanghai Cooperation Organisation, the Eurasian Economic Community, the Organisation for Security and Cooperation in Europe (OSCE), and the World Trade Organisation (WTO) and the leading member of the Commonwealth of Independent States."
The text file for Ukraine.txt is below
"
The territory of Ukraine was first inhabited at least 44,000 years ago, with the country being a candidate site for both the domestication of the horse and for the origins of the Indo-European language family. In the Middle Ages, the area became a key center of East Slavic culture, as epitomized by the powerful state of Kievan . Following its fragmentation in the 13th century, Ukraine was contested, ruled and divided by a variety of powers. A Cossack republic emerged and prospered during the 17th and 18th centuries, but Ukraine remained otherwise divided until its consolidation into a Soviet republic in the 20th century, becoming an independent nation-state only in 1991. Ukraine has long been a global breadbasket due to its extensive, fertile farmlands. As of 2011, it was the world's third-largest grain exporter with that year's harvest being much larger than average. Ukraine is one of ten most attractive agricultural land acquisition regions. Additionally, the country has a well-developed manufacturing sector, particularly in the area of aerospace and industrial equipment. Ukraine is a unitary state composed of 24 oblasts (provinces), one autonomous republic (Crimea) and two cities with special status: Kiev, its capital and largest city and Sevastopol, which houses the Russian Black Sea Fleet under a leasing agreement. Ukraine is a republic under a semi-presidential system with separate legislative, executive, and judicial branches. Since the dissolution of the Soviet Union, Ukraine continues to maintain the second-largest military in Europe, after that of Russia, when reserves and paramilitary personnel are taken into account. The country is home to 44.6 million people, 77.8% of whom are ethnic Ukrainians, with sizable minorities of ethnic Russians (17%), Belarusians, Tatars and Romanians. Ukrainian is the official language of Ukraine; its alphabet is Cyrillic. Russian is also widely spoken. The dominant religion in the country is Eastern Orthodox Christianity, which has strongly influenced Ukrainian architecture, literature and music. The name Ukraine means "borderland". "The Ukraine" was once the usual form in English but since the Declaration of Independence of Ukraine, the English-speaking world has largely stopped using the definite article."
I have the dictionary.txt file but can someone please help me achieve an output that looks something like this(below) when i input these files into the command line?
OUTPUT:
"======== Spell checking Russia.txt ========= 1 : The nation's history began with that of the East Slavs, who emerged as a recognizable group in Europe between the 3rd and 8th centuries AD. slavs not in dictionary. Add to dictionary? (y/n) n europe not in dictionary. Add to dictionary? (y/n) n 2 : Founded and ruled by a Varangian warrior elite and their descendants, the medieval state of Rus arose in the 9th century. varangian not in dictionary. Add to dictionary? (y/n) n rus not in dictionary. Add to dictionary? (y/n) n 3 : In 988 it adopted Orthodox Christianity from the Byzantine Empire, beginning the synthesis of Byzantine and Slavic cultures christianity not in dictionary. Add to dictionary? (y/n) y byzantine not in dictionary. Add to dictionary? (y/n) n slavic not in dictionary. Add to dictionary? (y/n) n 4 : that defined Russian culture for the next millennium. Rus ultimately disintegrated into a number of smaller states; russian not in dictionary. Add to dictionary? (y/n) n 6 : The Grand Duchy of Moscow gradually reunified the surrounding Russian principalities, achieved independence from the moscow not in dictionary. Add to dictionary? (y/n) n 7 : Golden Horde, and came to dominate the cultural and political legacy of Kievan Rus. By the 18th century, the nation had greatly kievan not in dictionary. Add to dictionary? (y/n) n 9 : stretching from Poland in Europe to Alaska in North America. poland not in dictionary. Add to dictionary? (y/n) n america not in dictionary. Add to dictionary? (y/n) n 10 : Following the Russian Revolution, the Russian Soviet Federative Socialist Republic became the largest and leading constituent of the federative not in dictionary. Add to dictionary? (y/n) n 11 : Soviet Union, the world's first constitutionally socialist state and a recognized superpower, which played a decisive role in the constitutionally not in dictionary. Add to dictionary? (y/n) n superpower not in dictionary. Add to dictionary? (y/n) n 12 : Allied victory in World War II. The Soviet era saw some of the most significant technological achievements of the 20th century, ii not in dictionary. Add to dictionary? (y/n) n 13 : including the world's first spacecraft, and the first astronaut. Following the dissolution of the Soviet Union in 1991, the Russian SFSR sfsr not in dictionary. Add to dictionary? (y/n) n 14 : reconstituted itself as the Russian Federation and is recognized as the continuing legal personality of the Union state. reconstituted not in dictionary. Add to dictionary? (y/n) n 15 : The Russian economy ranks as the eighth largest by nominal GDP and sixth largest by purchasing power parity. Russia's extensive mineral gdp not in dictionary. Add to dictionary? (y/n) n russia not in dictionary. Add to dictionary? (y/n) n 18 : Russia is a great power and a permanent member of the United Nations Security Council, a member of the G8, G20, the Council of Europe, g8 not in dictionary. Add to dictionary? (y/n) n g20 not in dictionary. Add to dictionary? (y/n) n 19 : the Asia-Pacific Economic Cooperation, the Shanghai Cooperation Organisation, the Eurasian Economic Community, the Organisation for asia not in dictionary. Add to dictionary? (y/n) n organisation not in dictionary. Add to dictionary? (y/n) n eurasian not in dictionary. Add to dictionary? (y/n) n ****** Miss spelled words ******* america asia byzantine constitutionally eurasian europe federative g20 g8 gdp ii kievan moscow organisation poland reconstituted rus russia russian sfsr slavic slavs superpower varangian ======== Spell checking Ukraine.txt ========= 2 : The territory of Ukraine was first inhabited at least 44,000 years ago, with the country being a candidate site ukraine not in dictionary. Add to dictionary? (y/n) n 3 : for both the domestication of the horse and for the origins of the Indo-European language family. indo not in dictionary. Add to dictionary? (y/n) n european not in dictionary. Add to dictionary? (y/n) n 4 : In the Middle Ages, the area became a key center of East Slavic culture, as epitomized by the powerful slavic not in dictionary. Add to dictionary? (y/n) n 5 : state of Kievan . Following its fragmentation in the 13th century, Ukraine was contested, ruled and divided by a variety of powers. kievan not in dictionary. Add to dictionary? (y/n) n 8 : Ukraine has long been a global breadbasket due to its extensive, fertile farmlands. As of 2011, it was the world's third-largest breadbasket not in dictionary. Add to dictionary? (y/n) n 13 : Kiev, its capital and largest city and Sevastopol, which houses the Russian Black Sea Fleet under a leasing agreement. kiev not in dictionary. Add to dictionary? (y/n) "
Step by Step Solution
There are 3 Steps involved in it
Step: 1
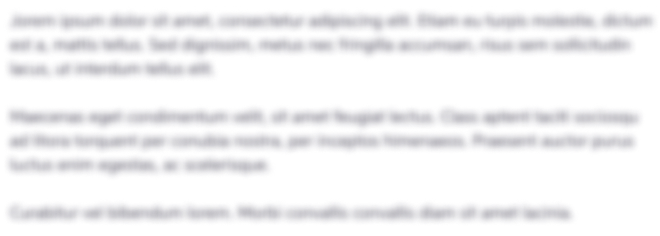
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started