Question
Java Problem: Need help with writing test cases. Plesee help if you can. I would greatly appreciate your helps Please help me write a series
Java Problem: Need help with writing test cases. Plesee help if you can. I would greatly appreciate your helps
Please help me write a series of "black box" test cases based on the Javadoc. The link to the package and Javadoc are provided at the bottom.
There are 13 implementations, and impl 2,8 and 11 are broken. The other implementations must pass all test cases, except impl 2, 8 and 11. This program is about PayRoll.
Here is the summary of the task:
The task is to add many @Test-annotated methods to PayrollTest.java and determine which of the many implementations are bad, and which one is good.
The code we're testing out tries to calculate various salary-related things based on employees and their (yearly) salaries. The implementor got a bit carried away and made a very generic Dict
We're only supplying the .class files for each implementation; you don't get to look for the bugs directly, you have to just think about what the purpose of the code is, and then write test cases based on that purpose. (You're writing "black box" test cases).
Running Your Tests
Note that running one test file against many implementations can be tedious if we're not smart about it. A typical (but bad) novice approach is to make many copies of the file which will quickly get out of sync as changes are made to one and the copies are not updated.
Instead, utilize the ability of the compiler and runtime of java to set a class path on the command line to cause a search for classes in the specified locations. Navigate to the testme/ directory and run commands like the following which runs tests on implementation 1 only:
demo$ javac -cp .:imp1/:junit-4.12.jar *.java demo$ java -cp .:imp1/:junit-4.12.jar PayrollTest
These commands tell Java that it should first compile all .java files it finds when looking in the current directory (.), in the imp1/ directory, and it should also look through the junit-4.12.jar file while compiling; then, it again needs access to code in all three of those locations, but it should run the main method of PayrollTest.
If you want to run tests on a different implementation, just change the imp1 to a different directory, as in
demo$ javac -cp .:imp2/:junit-4.12.jar *.java demo$ java -cp .:imp2/:junit-4.12.jar PayrollTest
For this task, the command line is likely superior to most IDEs. For instance, these commands will not work in DrJava. It's likely that most IDEs will struggle with this task. Take this as an opportunity to beef up your command line skills. It offers the ultimate flexibility and the only price to unlock that is a little practice (on the order of cut-pasting some ready-made commands).
Scripts to run tests
To ease the task of testing on the command line, two scripts are provided which will compile and run all implementations.
On Unix/Mac OS X use the unix-compile-test.sh script in a terminal.
> cd testme > unix-compile-test.sh
Results of testing all implementations are summarized by only showing how many tests failed (or that it was OK!). If you need to understand why a particualr implementation is behaving some way, then don't use the script here, use the just-one-implementation commands shown above!
On Windows use the windows-compile-test.cmd script in the cmd.exe terminal.
> cd testme > windows-compile-test.cmd
Results will be left in the results.txt text file due to the lack of a good grep on the Windows platform.
Goals for the Testing Problem
Multiple implementations of the payroll package are provided. One of these is correct while the remainder are crap. It is known that implementations imp2, imp8, and imp11 are broken.
To complete this project, do the following:
Create enough tests that all implementations but one are failing at least one test.
Write tests for both the Dict and Payroll classes. Problems may exist in one, the other, or both.
Document your test cases to indicate what they are testing. Part of your grade will be assigned based on the documentation of test cases.
Submit your PayrollTest.java file which we will run against all implementations.
Identify the general flaws in the behavior of each of the known broken implementations. Describe these flaws in a few sentences in the provided ANSWERS.txt file which will be submitted with your code.
----Here is the Demo---
Demo of Dict
The Dict class provides a quite simple dictionary implementation. Below is a demonstration of creating one and calling some of its methods.
> import payroll.*; > Dictd = new Dict (); > d.put("one",1); > d {(one:1)} > d.size() 1 > d.put("two",2); > d.put("three",3); > d.size() 3 > d.get("one") 1 > d.get("350") java.util.NoSuchElementException: "350" key not in dict. at payroll.Dict.complainNoKey(Dict.java:147) at payroll.Dict.get(Dict.java:70) > d.pop("two") 2 > d.toString() "{(one:1),(three:3)}" > d.keys() [one, three] > d.clear() > d {} >
Demo of Payroll
The Payroll class uses the Dict class in its implementation of some salary-related calculations. Below is a demonstration of some basic uses of Payroll.
> import payroll.*; > Payroll p = new Payroll() > p.hire("A",120); > p.hire("A",120) > p.hire("B",240) > p.employees() [A, B] > p.topSalary() 240 > p.hire("C",1500) > p.topSalary() 1500 > p.fire("C") true > p.employees() [A, B] > p.fire("C") false > p.giveRaise("A",0.5) > p.getSalary("A") 180 > p.giveRaise(1.0) // everyone gets 100% raise! > p.getSalary("A") 360 > p.getSalary("B") 480 > p.monthlyExpense() // for one month of twelve. 70 >
----Here is the java test that must be modified---
import org.junit.*; // Rule, Test import org.junit.rules.Timeout; import static org.junit.Assert.*;
import java.util.*;
import payroll.*;
public class PayrollTest { public static void main(String args[]){ org.junit.runner.JUnitCore.main("PayrollTest"); } // 1 second max per method tested @Rule public Timeout globalTimeout = Timeout.seconds(1);
// BEGIN TESTS HERE.
// Eliminate this test after you get the hang of things @Test public void example(){ assertEquals(5,5); assertFalse(5==6); assertTrue(6==6); } }
---Here is the link to the package of all the implementations and Javadoc---
https://paste.ee/p/6w7Tp
Please open and download the package.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
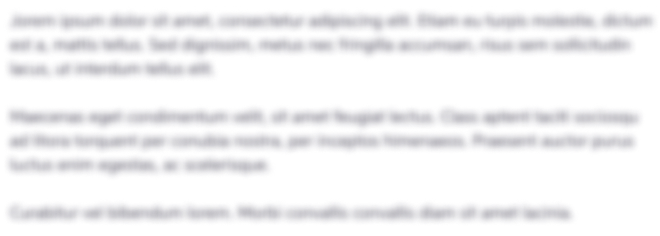
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started