Question
JAVA problem: Task 4: Reading Arrays public static int sumEvens(int[] xs) Given an array of integers, find all the even ones, and add them up;
JAVA problem:
Task 4: Reading Arrays
public static int sumEvens(int[] xs)
Given an array of integers, find all the even ones, and add them up; return this sum.
public static int sumTwoLargest(int[] xs)
Given an array of integers, find the largest two values in the array, and return their sum.
When the array is empty, return zero.
When there is only one item in the array, return that single number.
When there are duplicates of the highest value, use both of them. For example, feeding the array {1,4,5,5,2} to this method would return (5+5)==10. (It doesn't use the 4.)
Manual Inspection Criteria(5%) Solve this one with a single loop (any kind).
public static double projectsAverage(int[] scores, String[]kinds)
We have two array parameters of the same length; at the same index location, the scores array contains an integer from 0 to 100, and the kinds array contains one of these strings: "test", "project", "lab", and "quiz". Find all the project scores, and calculate their average. Return this double answer.
the length of the arrays might be zero.
there might not be any project scores recorded yet.
Manual Inspection Criteria(5%) Solve this one with a single loop (any kind).
Solutions go here:
public class Task4 { public static int sumEvens(int[] xs){ // delete this when you implement it. throw new RuntimeException("not implemented!"); } public static int sumTwoLargest(int[] xs){ // delete this when you implement it. throw new RuntimeException("not implemented!"); } public static double projectsAverage(int[] scores, String[]kinds){ // delete this when you implement it. throw new RuntimeException("not implemented!"); } }
Tester :
import org.junit.*; import static org.junit.Assert.*; import java.util.*; public class Task4Tests { public static void main(String args[]){ org.junit.runner.JUnitCore.main("Task4Tests"); } @Test public void task4_sumEvens_01() { assertEquals( 0, Task4.sumEvens( new int[]{} )); } @Test public void task4_sumEvens_02() { assertEquals( 10, Task4.sumEvens( new int[]{4,6} )); } @Test public void task4_sumEvens_03() { assertEquals( 0, Task4.sumEvens( new int[]{1,3,5} )); } @Test public void task4_sumEvens_04() { assertEquals( 10, Task4.sumEvens( new int[]{8,1,2,3} )); } @Test public void task4_sumEvens_05() { assertEquals( 44, Task4.sumEvens( new int[]{10,14,5,7,9,20} )); } @Test public void task4_sumEvens_06() { assertEquals( 30, Task4.sumEvens( new int[]{10,1,9,2,8,3,7,4,6,5} )); } @Test public void task4_sumEvens_07() { assertEquals( 20, Task4.sumEvens( new int[]{1,3,5,7,4,4,4,4,4} )); } @Test public void task4_sumEvens_08() { assertEquals( 50, Task4.sumEvens( new int[]{1,10,-1,-10, 50} )); } @Test public void task4_sumEvens_09() { assertEquals( -6, Task4.sumEvens( new int[]{-6} )); } @Test public void task4_sumEvens_10() { assertEquals( 100100, Task4.sumEvens( new int[]{100,100000} )); } @Test public void task4_sumTwoLargest_01() { assertEquals( 3, Task4.sumTwoLargest( new int[]{1,2} )); } @Test public void task4_sumTwoLargest_02() { assertEquals( 9, Task4.sumTwoLargest( new int[]{2,5,4} )); } @Test public void task4_sumTwoLargest_03() { assertEquals(11, Task4.sumTwoLargest( new int[]{6,4,5} )); } @Test public void task4_sumTwoLargest_04() { assertEquals( 7, Task4.sumTwoLargest( new int[]{1,2,2,4,3,1} )); } @Test public void task4_sumTwoLargest_05() { assertEquals(13, Task4.sumTwoLargest( new int[]{5,4,6,7} )); } @Test public void task4_sumTwoLargest_06() { assertEquals(14, Task4.sumTwoLargest( new int[]{8,3,4,5,6} )); } @Test public void task4_sumTwoLargest_07() { assertEquals(31, Task4.sumTwoLargest( new int[]{31} )); } @Test public void task4_sumTwoLargest_08() { assertEquals(40, Task4.sumTwoLargest( new int[]{4,3,20,20} )); } @Test public void task4_sumTwoLargest_09() { assertEquals(-7, Task4.sumTwoLargest( new int[]{-4,-6,-5,-3} )); } @Test public void task4_sumTwoLargest_10() { assertEquals( 0, Task4.sumTwoLargest( new int[]{} )); } // when deciding what amount of rounding error is acceptable for our double-typed answers, we choose none. public final double EXACT = 0; @Test public void task4_projectsAverage_01() { assertEquals( 0, Task4.projectsAverage( new int[]{}, new String[]{} ), EXACT); } @Test public void task4_projectsAverage_02() { assertEquals( 90, Task4.projectsAverage( new int[]{90}, new String[]{"project"} ), EXACT); } @Test public void task4_projectsAverage_03() { assertEquals( 0, Task4.projectsAverage( new int[]{100}, new String[]{"test"} ), EXACT); } @Test public void task4_projectsAverage_04() { assertEquals( 85, Task4.projectsAverage( new int[]{75,85}, new String[]{"lab","project"} ), EXACT); } @Test public void task4_projectsAverage_05() { assertEquals( 86, Task4.projectsAverage( new int[]{85,90,83}, new String[]{"project","project","project"} ), EXACT); } @Test public void task4_projectsAverage_06() { assertEquals( 94, Task4.projectsAverage( new int[]{95,97,93}, new String[]{"project","lab","project"} ), EXACT); } @Test public void task4_projectsAverage_07() { assertEquals( 95, Task4.projectsAverage( new int[]{55,76,24,95}, new String[]{"lab","test","quiz","project"} ), EXACT); } @Test public void task4_projectsAverage_08() { assertEquals(90.125, Task4.projectsAverage( new int[]{90,90,90,90,90,90,78,90,91}, new String[]{"project","project","project","project","project","project","lab","project","project"} ), EXACT); } @Test public void task4_projectsAverage_09() { assertEquals( 0, Task4.projectsAverage( new int[]{1,2,3,4}, new String[]{"quiz","quiz","lab","test"} ), EXACT); } @Test public void task4_projectsAverage_10() { assertEquals( 0, Task4.projectsAverage( new int[]{0,0,0}, new String[]{"project","project","project"} ), EXACT); }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
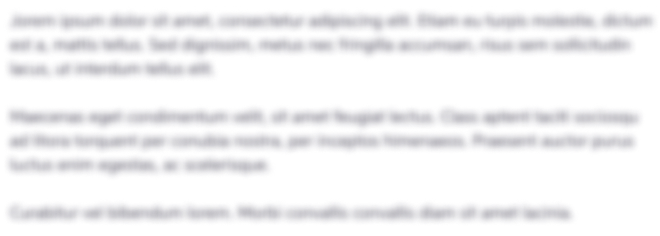
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started