Question
Java Program A Scavenger Hunt is a party game that people play in teams. They are given a list of items to find throughout the
Java Program
A Scavenger Hunt is a party game that people play in teams. They are given a list of items to find throughout the surrounding neighborhood. Our challenge is to find the best data structure to store the results of the hunt.
Create a list of 100 items that you might have people find on the hunt. These should be items that they have a chance of finding through out the neighboorhood. For example, yesterday's newspaper, a shoelace, a paperbag,.... Load the list anyway you want (console input, flatfile, etc.)
You will run the program two times, once using a set of ArrayLists, and once using a set of LinkedLists. Our pupose is to find the more efficient data structure to use for a scavenger hunt.
Use an Iterator to traverse the Collection from beginning to end. Then iterate through the Collection from end to beginning (backwards). Time the results. Which data structure is more efficient for looping through the entire Collection?
Ask the user how many teams will play the game. Create this number of teams. For each team load all of the items from the list. Shuffle the list after loading the items each time. Find the total time it takes to add the items to all of the teams.
Ask the user what position in the list to be used for retrieving and inserting elements. Retrieve that element from each of the teams. Find the total time it takes.
Next insert a new element at that position in each of the lists. Find the total time it takes.
Use the Random class to generate a number between 0 and the 100. Find the element in the scavenger hunt list at that position. Retrieve that element from each of the lists. Find the total time it takes.
Display the time in milliseconds for each of these operations.
Run the program several times with different input values and see how it affects the output.
Write a brief analysis of your findings along with your recommendations.
Make sure you use appropriate exception handling. This would be related to how you initially set up the list of items for the scanvenger hunt and how you have the user enter the valus for the number of teams and the element to work worth.
The following code snippet will help with the timing
Date today = new Date(); long time1, time2; time1=System.currentTimeMillis(); . . . . time2=System.currentTimeMillis(); System.out.println("Time for the operation is: " + (time2-time1));
If the time difference is too small, try:
long startTime = System.nanoTime(); // ... the code being measured ... long estimatedTime = System.nanoTime() - startTime;
For all programs, catch and handle the Exceptions appropriately and validate the input data where needed.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
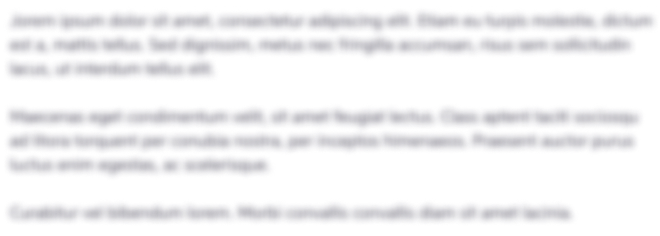
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started