Question
##JAVA PROGRAM. I NEED HELP CODING WHERE STUDENT IMPLEMENTATION IS REQUIRED. #JAVA LONG ARRAY SEQUENCE#NEED HELP CODING WHERE STUDENT IMPLEMENTATION IS REQUIRED public class LongArraySequence
##JAVA PROGRAM. I NEED HELP CODING WHERE STUDENT IMPLEMENTATION IS REQUIRED.
#JAVA LONG ARRAY SEQUENCE#NEED HELP CODING WHERE STUDENT IMPLEMENTATION IS REQUIRED
public class LongArraySequence implements Cloneable, Iterable {
// *************************************************** // ATTRIBUTES/PROPERTIES // ***************************************************
private int used; private int cursor; private long[] data;
// *************************************************** // CONSTRUCTORS // ***************************************************
/* default constructor */ public LongArraySequence( ) { this.used = 0; this.cursor = 0; this.data = new long[ 1 ]; }
/* parameterized constructor */ public LongArraySequence( int initialCapacity ) { if ( initialCapacity < 1) throw new IllegalArgumentException("initialCapacity must be > 0"); this.used = 0; this.cursor = 0; try { this.data = new long[ initialCapacity ]; } catch (OutOfMemoryError err) { throw new OutOfMemoryError("Could not accommodate capacity request"); } }
/* copy constructor */ public LongArraySequence( LongArraySequence other ) { if ( other == null ) { throw new NullPointerException("other must not be null"); }
// STUDENT IMPLEMENTATION GOES HERE
}
@Override public LongArraySequence clone( ) { LongArraySequence result;
try { result = (LongArraySequence) super.clone( ); result.data = this.data.clone( ); return result; } catch (CloneNotSupportedException e) { throw new RuntimeException("This class does not implement Cloneable"); } }
// *************************************************** // ACCESSORS // ***************************************************
@Override public boolean equals( Object other ) { if ( !(other instanceof LongArraySequence) ) return false; LongArraySequence candidate = (LongArraySequence) other; if ( candidate == null ) return false; if ( this == candidate ) return true;
System.out.printf("this %s ", this); System.out.printf("other %s ", candidate);
if ( this.used != candidate.used ) return false; if ( this.cursor != candidate.cursor ) return false; if ( this.hashCode() != candidate.hashCode() ) return false; boolean isEqual = true; //assume equal until proven otherwise int index = 0; while ( isEqual && index < this.used ) { if ( this.data[ index ] != candidate.data[ index ] ) isEqual = false; else index++; } return isEqual; }
@Override public int hashCode( ) { int hashValue = Objects.hash( this.used ) + Objects.hash( this.cursor ) ;
for ( int index = 0; index < this.used; ++index ) { hashValue += Objects.hash( this.data[ index ] ); } return hashValue; }
@Override public String toString( ) { StringBuffer sb = new StringBuffer(); sb.append("LongArraySequence: "); sb.append(this.used); sb.append(" items"); sb.append("-->[ "); if ( this.used > 0 ) { if ( this.cursor == 0 ) sb.append("^"); sb.append(this.data[ 0 ]); for (int index = 1; index < this.used; index++) { sb.append(", "); if ( index == this.cursor ) sb.append("^"); sb.append(this.data[ index ]); } } sb.append(" ]"); sb.append(" Capacity: "); sb.append(this.data.length);
return sb.toString(); }
public int size( ) { return this.used; }
public int getCapacity( ) { return this.data.length; }
public boolean isCurrent( ) { return this.cursor < this.used && this.cursor >= 0; }
public long getCurrent( ) { if ( !this.isCurrent() ) { throw new IllegalStateException("There is no current element"); } return this.data [ this.cursor ]; }
// *************************************************** // MUTATORS // ***************************************************
public void ensureCapacity( int minimumCapacity ) { if ( minimumCapacity < 1 ) throw new IllegalArgumentException("minimumCapacity must be >= 1"); // STUDENT IMPLEMENTATION GOES HERE }
public void trimToSize( ) { if ( this.used == 0 ) { this.data = new long[ 1 ]; this.cursor = 1; return; } // STUDENT IMPLEMENTATION GOES HERE
}
public LongArraySequence concatenation( LongArraySequence other ) { if ( other == null ) { throw new NullPointerException("other must be non-null"); }
LongArraySequence newSequence = new LongArraySequence(); // STUDENT IMPLEMENTATION GOES HERE
return newSequence; }
public void start( ) { this.cursor = 0; }
public void advance() { if ( this.cursor == this.used ) throw new IllegalStateException("Attempting to advance cursor beyond end of sequence"); else this.cursor++; }
public void addBefore( long newEntry ) { if ( this.data.length == this.used ) this.ensureCapacity( this.used * 2 ); // STUDENT IMPLEMENTATION GOES HERE
}
public void addAfter( long newEntry ) { if ( this.data.length == this.used ) this.ensureCapacity( this.used * 2 ); // STUDENT IMPLEMENTATION GOES HERE
}
public void removeCurrent( ) { if ( !this.isCurrent() ) throw new IllegalStateException("No current item defined");
// STUDENT IMPLEMENTATION GOES HERE
} public Iterator iterator() { return new SequenceIterator( )
Step by Step Solution
There are 3 Steps involved in it
Step: 1
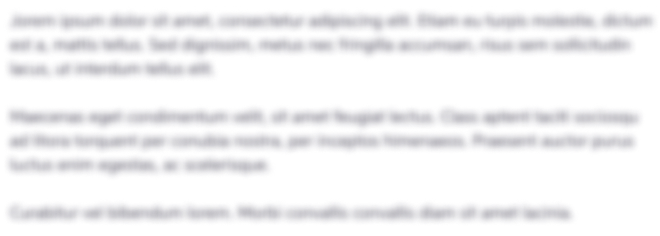
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started