Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Java program: Screenshot your output Use the program provided below then write a similar program for this exercise. (We cannot use libraries to do this,
Java program: Screenshot your output
Use the program provided below then write a similar program for this exercise. (We cannot use libraries to do this, everything must be coded) Note: The program below is a sample program for your reference. It cannot be used for coding.
1. BagInterface.java
public interface BagInterface{ /** Gets the current number of entries in this bag. * @return the integer number of entries currently in the bag */ public int getCurrentSize(); /** Sees whether this bag is full. * @return true if the bag is full, or false if not */ public boolean isFull(); /** Sees whether this bag is empty. * @return true if the bag is empty, or false if not */ public boolean isEmpty(); /** Adds a new entry to this bag. * @param newEntry the object to be added as a new entry * @return true if the addition is successful, or false if not */ public boolean add(T newEntry); /** Removes one unspecified entry from this bag, if possible. * @return either the removed entry, if the removal * was successful, or null */ public T remove(); /** Removes one occurrence of a given entry from this bag, if possible. * @param anEntry the entry to be removed @return true if the removal was successful, or false if not */ public boolean remove(T anEntry); /** Removes all entries from this bag. */ public void clear(); /** Counts the number of times a given entry appears in this bag. * @param anEntry the entry to be counted @return the number of times anEntry appears in the bag */ public int getFrequencyOf(T anEntry); /** Tests whether this bag contains a given entry. * @param anEntry the entry to locate @return true if the bag contains anEntry, or false otherwise */ public boolean contains(T anEntry); /** Creates an array of all entries that are in this bag. * @return a newly allocated array of all the entries in the bag */ public T[] toArray(); }//End Bag Interface
2. NodeBaseBag.java
public class NodeBasedBagimplements BagInterface { Node firstNode; int numberOfNodes = 0; public NodeBasedBag() { firstNode = null; numberOfNodes = 0; } @Override public int getCurrentSize() { return numberOfNodes; } @Override public boolean isEmpty() { return numberOfNodes == 0; } @Override public boolean add(T newEntry) { // add to beginning of chain: Node newNode = new Node(newEntry); newNode.next = firstNode; // make new node reference rest of chain // (firstNode is null if chain is empty) firstNode = newNode; // new node is at beginning of chain numberOfNodes++; return true; } @Override public T remove() { T result = null; if (firstNode != null) { result = firstNode.item; firstNode = firstNode.next; // remove first node from chain numberOfNodes--; } // end if return result; } private Node getReferenceTo(T anEntry) { boolean found = false; Node currentNode = firstNode; while (!found && (currentNode != null)) { if (anEntry.equals(currentNode.item)) { found = true; } else { currentNode = currentNode.next; } } // end while return currentNode; } // end getReferenceTo @Override public boolean remove(T anEntry) { boolean result = false; Node nodeN = getReferenceTo(anEntry); if (nodeN != null) { nodeN.item = firstNode.item; // replace located entry with entry // in first node remove(); // remove first node result = true; } // end if return result; } @Override public void clear() { while (!isEmpty()) { remove(); } } @Override public int getFrequencyOf(T anEntry) { int frequency = 0; int counter = 0; Node currentNode = firstNode; while ((counter 3. NodeBaseBagTest.java
public class NodeBasedBagimplements BagInterface { Node firstNode; int numberOfNodes = 0; public NodeBasedBag() { firstNode = null; numberOfNodes = 0; } @Override public int getCurrentSize() { return numberOfNodes; } @Override public boolean isEmpty() { return numberOfNodes == 0; } @Override public boolean add(T newEntry) { // add to beginning of chain: Node newNode = new Node(newEntry); newNode.next = firstNode; // make new node reference rest of chain // (firstNode is null if chain is empty) firstNode = newNode; // new node is at beginning of chain numberOfNodes++; return true; } @Override public T remove() { T result = null; if (firstNode != null) { result = firstNode.item; firstNode = firstNode.next; // remove first node from chain numberOfNodes--; } // end if return result; } private Node getReferenceTo(T anEntry) { boolean found = false; Node currentNode = firstNode; while (!found && (currentNode != null)) { if (anEntry.equals(currentNode.item)) { found = true; } else { currentNode = currentNode.next; } } // end while return currentNode; } // end getReferenceTo @Override public boolean remove(T anEntry) { boolean result = false; Node nodeN = getReferenceTo(anEntry); if (nodeN != null) { nodeN.item = firstNode.item; // replace located entry with entry // in first node remove(); // remove first node result = true; } // end if return result; } @Override public void clear() { while (!isEmpty()) { remove(); } } @Override public int getFrequencyOf(T anEntry) { int frequency = 0; int counter = 0; Node currentNode = firstNode; while ((counter Homework 04 om In this project you will write the code to implement a dynamic (node-based) bag. Use the Node-based BAG code as a starting point and add the required code to fully implement it. For this assignment we will use a bag of coins. A bag of coins is a simply a bag that contains a set of coins objects. The user enters the coin value. Then, you create a coin object and add it to the bag. Specifications: I. Class Coin: There is only four coin values: 10 cents, 25 cents, 75 cents and 100 cents. Other values are not allowed. The class is very simple has only one data member coin Value. But you might need to override methods such as: toString, equal, and compare To. The menu that appears to user should like this: [1] Adding a coin [2] Removing a coin [3] Removing a specific coin [4] Clear the bag [5] Get the frequency of a given coin [6] Check if specific coin exists in the bag [7] Get minimum coin value [8] Get maximum 19] Print bag contents [10] Print bag contents ordered [11] Exit II. A bag of coins: Use the node based BAG. The bag should support the following Operations: (a) Add a coin : When called the following steps happen 1. the user is asked to enter the value of the coin then the coin created with the specific value and added to the bag. 2. Add the coin object to the bag (b) Removing a coin: When called, you should remove the last coin added to the bag (c) Removing a specific coin: When called you should ask the user to specify the value of the coin you want to remove, based in that you search the bag and remove a coin of the same value. If the coin doesn't exist then you display a message indicating that the specific coin doesn't exist in the bag (d) Clear the bag: This method resets every thing i.e. clears the bag. (e) Get frequency: When called, you should ask the user to specify the coin value. Based on that, you return the number of occurrences of that value. (f) Check if Exist: When called, you ask the user the coin value he/she want to check. You search for it, if the coin of that value exist you display a message indicating its existence, otherwise you display a message that the coin is not in the bay. (g) Get minimum coin : returns the smallest coin value in the bag and print it (h) Get maximum coin: returns the largest coin value found in the bag and print it (i) Print contents: You print the bag contents as is. 6) Print contents sorted: You print the content sorted in ascending order ( Note: you should not change the original bag content, just print them on order based on their value)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
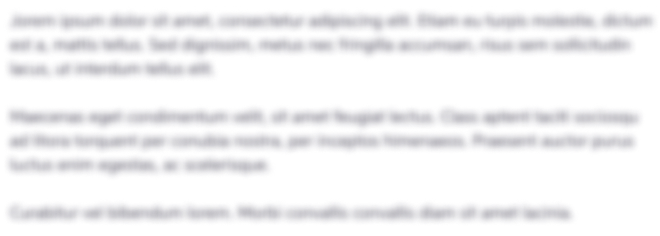
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started