Question
Java Program Specification You are to write a class called MovieCollection following the diagram below. - means private, + means public. MovieCollection - movies: Movie[]
Java
Program Specification
You are to write a class called MovieCollection following the diagram below. - means private, + means public.
MovieCollection |
---|
- movies: Movie[] |
- movieCount: int |
+ MovieCollection() |
+ getTotalMovies(): int |
+ addMovie(movie,Movie): boolean |
+ addMovieAt(movie, Movie: index, int): boolean |
+ findMovie(movie, Movie): int |
+ getMovieAt(index, int): Movie |
+ removeMovie(movie, Movie): boolean |
+ removeMovieAt(index, int): Movie |
- shiftCollectionLeft(index, int) |
+findBestMovie(): Movie |
+ moviesToAvoid() |
+ moviesToWatch() |
+ PrintOutMovieList() |
- shiftCollectionRight(index, int) |
Movie |
---|
- name: String |
- minutes: int |
- tomatoScore: int |
+ Movie {name: String, minutes: int, tomatoScore: int) |
+ getName(): String |
+ getMinutes(): int |
+ getTomatoScore(): int |
+ setTomatoScore(score: int) |
+ isFresh(): boolean |
+ toString(): String |
+ equals(other: Object): boolean |
Requirements
Your Movie objects are stored in an array in the MovieCollection class. You are only allowed to hold 10 movies at a time, even though in real life you may own many more. Another instance variable you have to manage is the movieCount variable. The movieCount variable is meant to tell how many movies you currently have, and where the next movie is to be added if it can. The movieCount variable may only increment or decrement by one when necessary. Also, no null elements are allowed between the start of the array to movieCount, ie no empty movie slots. The movies do not need to be sorted. They are simply added to where movieCount is currently pointing or where specified by addMovieAt(int index). If you have 10 movies, it should not add any more movies unless you remove a movie. In the assignment description I have added many comments above each method to give further descriptions. I have provided Junit testing for most methods and a simple driver you may use to test your code. Below is a sample out. One last comment about the Movie class from lab, it may not have been as obvious from seeing the code below, but toString() converts the minutes to hours and minutes. For example, 125 converts to 2hrs 5min. You may add a private helper method to do the conversion if you wish.
Driver Output
Total movies: 7
All of my movies: Movie 1: Crazy Rich Asians
Movie 2: Oceans 8
Movie 3: The Happytime Murders Movie
4: Mission Impossible: Fallout
Movie 5: Slender man
Movie 6: The Big Sick
Movie 7: Mile 22
Can I add the The Big Sick again?
No, it is already in your collection.
Is A Quiet Place in my collection?
It is not there.
Time to remove Oceans 8. Oceans 8 was removed.
Can I remove Oceans 8 again?
Nope, it was already removed.
All of my movies:
Movie 1: Crazy Rich Asians
Movie 2: The Happytime Murders
Movie 3: Mission Impossible: Fallout
Movie 4: Slender man
Movie 5: The Big Sick
Movie 6: Mile 22
Time to add Jurassic World: Fallen Kingdom at the end of collection.
Wonder Woman at the beginning.
Goodfellas at slot 8.
Finally A Quiet Place at the end.
All of my movies:
Movie 1: Wonder Woman
Movie 2: Crazy Rich Asians
Movie 3: The Happytime Murders
Movie 4: Mission Impossible: Fallout
Movie 5: Slender man
Movie 6: The Big Sick
Movie 7: Mile 22
Movie 8: Goodfellas
Movie 9: Jurassic World: Fallen Kingdom
Movie 10: A Quiet Place
Movies to avoid:
Movie 1:
Name: The Happytime Murders
Length : 1hrs 31min
Tomato Rating : Rotten
Movie 2:
Name: Slender man
Length : 1hrs 33min
Tomato Rating : Rotten
Movie 3: Name: Mile 22
Length : 1hrs 30min
Tomato Rating : Rotten
Movie 4:
Name: Jurassic World: Fallen Kingdom
Length : 2hrs 9min
Tomato Rating : Rotten
Movies to watch:
Movie 1: Name: Wonder Woman
Length : 2hrs 21min
Tomato Rating : Fresh
Movie 2:
Name: Crazy Rich Asians
Length : 2hrs 0min
Tomato Rating : Fresh
Movie 3:
Name: Mission Impossible: Fallout
Length : 2hrs 27min
Tomato Rating : Fresh
Movie 4:
Name: The Big Sick Length : 1hrs 59min
Tomato Rating : Fresh
Movie 5:
Name: Goodfellas
Length : 2hrs 26min
Tomato Rating : Fresh
Movie 6:
Name: A Quiet Place
Length : 1hrs 30min
Tomato Rating : Fresh
The Best Movie is?
The Big Sick
Time to remove the best movie.
Success, it was removed correctly.
All of my movies:
Movie 1: Wonder Woman
Movie 2: Crazy Rich Asians
Movie 3: The Happytime Murders
Movie 4: Mission Impossible: Fallout
Movie 5: Slender man
Movie 6: Mile 22
Movie 7: Goodfellas
Movie 8: Jurassic World: Fallen Kingdom
Movie 9: A Quiet Place
Can I remove a movie at impossible indexes?
Nope, negative indexes do not work.
Nope, index is too large.
---------------------------------------------------------------
Code Provided
-------------------------------------------------------
JUnit Class
import static org.junit.Assert.*;
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
public class JUnit {
MovieCollection a;
MovieCollection b;
MovieCollection c;
MovieCollection d;
MovieCollection e;
MovieCollection f;
@Before
public void setUp() {
a = new MovieCollection();
b = new MovieCollection();
c = new MovieCollection();
d = new MovieCollection();
e = new MovieCollection();
f = new MovieCollection();
}
Movie crazyRichAsians = new Movie("Crazy Rich Asians",120,93);
Movie oceans8 = new Movie("Oceans 8",110,68);
Movie happytimeMurders = new Movie("The Happytime Murders",91,22);
Movie fallout = new Movie("Mission Impossible: Fallout",147,97);
Movie slenderMan = new Movie("Slender man",93,7);
Movie bigSick = new Movie("The Big Sick",119,98);
Movie mile22 = new Movie("Mile 22",90,22);
Movie fallenKingdom = new Movie("Jurassic World: Fallen Kingdom",129,49);
Movie wonderWoman = new Movie("wonder Woman",141,93);
Movie goodfellas = new Movie("Goodfellas",146,96);
Movie quietPlace = new Movie("A Quiet Place", 90, 95);
@After
public void tearDown(){
a = null;
b = null;
c = null;
d = null;
e = null;
f = null;
}
@Test
public void testAddMovie() {
assertEquals(true, a.addMovie(crazyRichAsians));
assertEquals(false, a.addMovie(crazyRichAsians));
assertEquals(true, a.addMovie(oceans8));
assertEquals(true, a.addMovie(happytimeMurders));
assertEquals(true, a.addMovie(fallout));
assertEquals(4, a.getTotalMovies());
assertEquals(true, a.addMovie(slenderMan));
assertEquals(true, a.addMovie(bigSick));
assertEquals(true, a.addMovie(mile22));
assertEquals(false, a.addMovie(slenderMan));
assertEquals(true, a.addMovie(fallenKingdom));
assertEquals(true, a.addMovie(wonderWoman));
assertEquals(true, a.addMovie(goodfellas));
assertEquals(false, a.addMovie(quietPlace));
assertEquals(10, a.getTotalMovies());
}
@Test
public void testFindMovieAndGetMovieAt(){
b.addMovie(crazyRichAsians);
b.addMovie(oceans8);
b.addMovie(happytimeMurders);
b.addMovie(fallout);
b.addMovie(slenderMan);
b.addMovie(bigSick);
b.addMovie(mile22);
b.addMovie(fallenKingdom);
b.addMovie(wonderWoman);
b.addMovie(goodfellas);
assertEquals(0, b.findMovie(crazyRichAsians));
assertEquals(1, b.findMovie(oceans8));
assertEquals(2, b.findMovie(happytimeMurders));
assertEquals(3, b.findMovie(fallout));
assertEquals(4, b.findMovie(slenderMan));
assertEquals(5, b.findMovie(bigSick));
assertEquals(6, b.findMovie(mile22));
assertEquals(7, b.findMovie(fallenKingdom));
assertEquals(8, b.findMovie(wonderWoman));
assertEquals(9, b.findMovie(goodfellas));
assertEquals(-1, b.findMovie(quietPlace));
assertEquals(10, b.getTotalMovies());
Movie tmpMovie = b.getMovieAt(1);
assertTrue(oceans8.equals(tmpMovie));
tmpMovie = b.getMovieAt(10);
assertTrue(tmpMovie == null);
tmpMovie = b.getMovieAt(6);
assertTrue(mile22.equals(tmpMovie));
tmpMovie = b.getMovieAt(-1);
assertTrue(tmpMovie == null);
}
@Test
public void testAddMovieAt(){
c.addMovie(crazyRichAsians);
c.addMovie(oceans8);
c.addMovie(slenderMan);
c.addMovie(goodfellas);
assertFalse(c.addMovieAt(crazyRichAsians, 3));
assertFalse(c.addMovieAt(bigSick, -1));
assertFalse(c.addMovieAt(bigSick, 5));
assertTrue(c.addMovieAt(happytimeMurders, 1));
assertTrue(happytimeMurders.equals(c.getMovieAt(1)));
assertTrue(c.addMovieAt(mile22, 1));
assertTrue(mile22.equals(c.getMovieAt(1)));
assertEquals(6,c.getTotalMovies());
assertTrue(c.addMovieAt(wonderWoman, 1));
assertTrue(wonderWoman.equals(c.getMovieAt(1)));
assertTrue(c.addMovieAt(fallout, 0));
assertTrue(fallout.equals(c.getMovieAt(0)));
assertEquals(8,c.getTotalMovies());
assertTrue(c.addMovieAt(bigSick, 6));
assertTrue(bigSick.equals(c.getMovieAt(6)));
assertTrue(c.addMovieAt(fallenKingdom, 9));
assertTrue(fallenKingdom.equals(c.getMovieAt(9)));
assertTrue(fallout.equals(c.getMovieAt(0)));
assertTrue(crazyRichAsians.equals(c.getMovieAt(1)));
assertTrue(wonderWoman.equals(c.getMovieAt(2)));
assertTrue(mile22.equals(c.getMovieAt(3)));
assertTrue(happytimeMurders.equals(c.getMovieAt(4)));
assertTrue(oceans8.equals(c.getMovieAt(5)));
assertTrue(bigSick.equals(c.getMovieAt(6)));
assertTrue(slenderMan.equals(c.getMovieAt(7)));
assertTrue(goodfellas.equals(c.getMovieAt(8)));
assertTrue(fallenKingdom.equals(c.getMovieAt(9)));
assertFalse(c.addMovieAt(bigSick, 5));
assertFalse(c.addMovieAt(fallenKingdom, 5));
assertEquals(10,c.getTotalMovies());
}
@Test
public void testRemoveMovie(){
d.addMovie(slenderMan);
d.addMovie(bigSick);
d.addMovie(mile22);
d.addMovie(fallenKingdom);
assertEquals(4,d.getTotalMovies());
assertEquals(true, d.removeMovie(bigSick));
assertEquals(3,d.getTotalMovies());
assertEquals(false, d.removeMovie(bigSick));
assertEquals(true, d.removeMovie(fallenKingdom));
assertEquals(2, d.getTotalMovies());
assertEquals(true, d.removeMovie(slenderMan));
assertEquals(true, d.removeMovie(mile22));
assertEquals(0, d.getTotalMovies());
assertEquals(false, d.removeMovie(mile22));
}
@Test
public void testRemoveMovieAT(){
f.addMovie(slenderMan);
f.addMovie(bigSick);
f.addMovie(goodfellas);
assertEquals(null, f.removeMovieAt(-1));
assertEquals(null, f.removeMovieAt(3));
assertTrue(slenderMan.equals(f.removeMovieAt(0)));
assertTrue(goodfellas.equals(f.removeMovieAt(1)));
assertTrue(bigSick.equals(f.removeMovieAt(0)));
assertEquals(0,f.getTotalMovies());
}
@Test
public void testFindBestMovie(){
e.addMovie(crazyRichAsians);
e.addMovie(oceans8);
e.addMovie(happytimeMurders);
assertEquals(3, e.getTotalMovies());
assertTrue(crazyRichAsians.equals(e.findBestMovie()));
e.addMovie(fallout);
assertEquals(4, e.getTotalMovies());
assertTrue(fallout.equals(e.findBestMovie()));
e.addMovie(slenderMan);
assertEquals(5, e.getTotalMovies());
assertTrue(fallout.equals(e.findBestMovie()));
e.addMovie(bigSick);
assertEquals(6, e.getTotalMovies());
assertTrue(bigSick.equals(e.findBestMovie()));
e.removeMovie(bigSick);
assertEquals(5, e.getTotalMovies());
assertTrue(fallout.equals(e.findBestMovie()));
}
}
--------------------------------------------------
MovieCollection Class
public class MovieCollection {
//TODO
//Instance Variables
/**
* Constructor.
* You are only allowed to hold 10 movies.
* Initialize the array.
*/
public MovieCollection(){
//TODO
}
/**
* Return the total number of movies.
* @return int
*/
public int getTotalMovies(){
//TODO
}
/**
* Add the passed in Movie to your collection if there is space for it.
* You are not allowed duplicate copies of a movie in the array.
* Make sure to check movieCount to make sure it can be added.
* If the movie is added return true. Else return false.
*
* @param movie
* @return boolean
*/
public boolean addMovie(Movie movie){
//TODO
}
/**
* Add a movie at the specifed index if the index is valid.
* You must verify the index. Remember, no duplicate movies are allowed.
* You will need to shift all your movies to the right to make room for the new movie.
* Return true if movie was added, false otherwise.
*
* @param movie
* @param index
* @return boolean
*/
public boolean addMovieAt(Movie movie, int index){
//TODO
}
/**
* Shift all movies to the right based on the index passed in.
* This will create 'space' for a new movie to be added.
* Should only be called by addMovieAt().
* Private methods of a class are considered helper methods.
*
* @param index
*/
private void shiftCollectionRight(int index) {
//TODO
}
/**
* Find location of the passed in Movie and return its location in the array.
* This means a value from [0, movieCount) could be returned if it is in the array.
* If the movie is not there, return -1 as a value.
* To compare movies, you only need to compare the name and runtime.
* Remember, you can use methods in Movie class to easily compare two movies now.
*
* @param movie
* @return boolean
*/
public int findMovie(Movie movie){
//TODO
}
/**
* Get the movie at the specified index and return it.
* If the index passed in is invalid, return null.
* No shifting required.
*
* @param index
* @return Movie
*/
public Movie getMovieAt(int index) {
//TODO
}
/**
* Remove the passed in Movie if it is there.
* If the movie is there, you will need to "shift" the array backwards one location to "remove" the movie.
* Remember, no null spaces are allowed in the array.
* You will need to call shiftCollectionLeft if you remove a movie.
* This method returns true if the movie is removed, false otherwise.
*
* @param movie
* @return boolean
*/
public boolean removeMovie(Movie movie){
//TODO
}
/**
* Remove the Movie at the specified index.
* Make sure the index is a valid index.
* You will need to call shiftCollectionLeft to remove the movie.
* Return the Movie that was at this location, or null if an invalid index was used.
*
* @param index
* @return Movie
*/
public Movie removeMovieAt(int index) {
//TODO
}
/**
* Move all elements after the index one location backwards in the array.
* This method is meant to be called by removeMovie() and removeMovieAt() and is private.
* Private methods of a class are considered helper methods.
* The parameter index is meant to be the location of the element to be removed.
*
* @param index
*/
private void shiftCollectionLeft(int index){
//TODO
}
/**
* Find the best movie according to Rotten Tomato score and return it.
* If the array is empty, meaning there are no movies, it returns null.
*
* @return Movie or null
*/
public Movie findBestMovie(){
//TODO
}
/**
* Print out all movies that are considered rotten in the array.
* Remember you can use methods in the Movie class to determine this.
* Refer to driver and handout for output format.
*
*/
public void moviesToAvoid(){
//TODO
}
/**
* Print out all movies that are considered fresh in the array.
* Remember you can use methods in the Movie class to determine this.
* Refer to driver and handout for output format.
*
*/
public void moviesToWatch(){
//TODO
}
/**
* Print out all movies in the array.
* You only need to print out the name of the movie, nothing more.
* Refer to driver and handout for output format.
*
*/
public void printOutMovieList(){
//TODO
}
}
----------------------------------------------
Movie Class
public class Movie {
//TODO
//You may use your Movie class from lab, but a few small things have changed.
//Look at the driver and UML diagram for changes.
}
--------------------------------------------------
MovieDriver Class
public class MovieDriver {
public static void main(String [] args){
MovieCollection myMovies = new MovieCollection();
Movie crazyRichAsians = new Movie("Crazy Rich Asians",120,93);
Movie oceans8 = new Movie("Oceans 8",110,68);
Movie happytimeMurders = new Movie("The Happytime Murders",91,22);
Movie fallout = new Movie("Mission Impossible: Fallout ",147,97);
Movie slenderMan = new Movie("Slender man",93,7);
Movie bigSick = new Movie("The Big Sick",119,98);
Movie mile22 = new Movie("Mile 22",90,22);
Movie fallenKingdom = new Movie("Jurassic World: Fallen Kingdom",129,49);
Movie wonderWoman = new Movie("Wonder Woman",141,93);
Movie goodfellas = new Movie("Goodfellas",146,96);
Movie quietPlace = new Movie("A Quiet Place", 90, 95);
myMovies.addMovie(crazyRichAsians);
myMovies.addMovie(oceans8);
myMovies.addMovie(happytimeMurders);
myMovies.addMovie(fallout);
myMovies.addMovie(slenderMan);
myMovies.addMovie(bigSick);
myMovies.addMovie(mile22);
System.out.println("Total movies: " + myMovies.getTotalMovies());
myMovies.printOutMovieList();
System.out.println("Can I add the " + bigSick.getName() + " again?");
if(myMovies.addMovie(bigSick)){
System.out.println("It was added.");
} else {
System.out.println("No, it is already in your collection.");
}
System.out.println();
System.out.println("Is " + quietPlace.getName() + " in my collection?");
if(myMovies.findMovie(quietPlace) == -1){
System.out.println("It is not there.");
} else {
System.out.println("It is there.");
}
System.out.println();
System.out.println("Time to remove " + oceans8.getName() + ".");
if(myMovies.removeMovie(oceans8)){
System.out.println(oceans8.getName() + " was removed.");
} else {
System.out.println(oceans8.getName() + " was not removed.");
}
System.out.println();
System.out.println("Can I remove " + oceans8.getName() + " again?");
if(myMovies.removeMovie(oceans8)){
System.out.println("The movie was removed.");
} else {
System.out.println("Nope, it was already removed.");
}
System.out.println();
myMovies.printOutMovieList();
System.out.println("Time to add " + fallenKingdom.getName() + " at the end of collection, "
+ wonderWoman.getName() + " at the beginning, "
+ goodfellas.getName() + " at slot 8, and "
+ "and finally" + quietPlace.getName() + " at the end.");
myMovies.addMovie(fallenKingdom);
myMovies.addMovieAt(wonderWoman,0);
myMovies.addMovieAt(goodfellas, 7);
myMovies.addMovie(quietPlace);
System.out.println();
myMovies.printOutMovieList();
myMovies.moviesToAvoid();
System.out.println();
myMovies.moviesToWatch();
System.out.println();
System.out.println("The Best Movie is?");
System.out.println(myMovies.findBestMovie().getName());
System.out.println(" Time to remove the best movie.");
Movie removedMovieAt = myMovies.removeMovieAt(myMovies.findMovie(myMovies.findBestMovie()));
if(bigSick.equals(removedMovieAt)) {
System.out.println("Success, it was removed correctly.");
myMovies.printOutMovieList();
} else {
System.out.println("Nope, wrong movie or no movie was removed.");
}
System.out.println();
System.out.println("Can I remove a movie at impossible indexes?");
if(myMovies.removeMovieAt(-1) == null) {
System.out.println("Nope, negative indexes do not work.");
} else {
System.out.println("Hmmm, something isn't right here. It should be null.");
}
if(myMovies.removeMovieAt(10) == null) {
System.out.println("Nope, index is too large.");
} else {
System.out.println("Hmmm, something isn't right here. It should be null.");
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
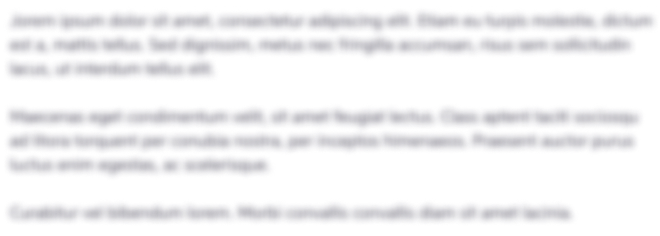
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started