Question
Java programming.. About You will implement the cabbage, goat, wolf game in a program. This program will also check to make sure each state is
Java programming..
About
You will implement the "cabbage, goat, wolf" game in a program. This program will also check to make sure each state is valid.
Turn in
Turn in all source files and a screenshot of your program running. This can be implemented in Java programming language.
Requirements
The program must:
Display the positions of all characters, on either island 1 or island 2.
Allow the user to move just the traveller, or the traveller/goat, traveller/wolf, traveller/cabbage.
Validate user input; don't let the user move an object if the traveller is not in the same position as that object.
Check for bad states; if the cabbage+goat are left alone (without traveller), or the goat+wolf are left alone (without traveller), the game ends.
If all 4 characters make it to island 2, the game has been won.
Program
Storing character information
There are many ways you can store where characters are currently at. For example:
Option A: Four position variables
int travelerPosition = 0; int cabbagePosition = 0; int goatPosition = 0; int wolfPosition = 0;
Option B: Two separate island arrays
char island1[4] = { 't', 'c', 'g', 'w' }; char island2[4] = { ' ', ' ', ' ', ' ' };
Option C: One 2D island array
You might start out with a 2D array, where the row is the "island" and the column is the "object". For example:
char islandStates[2][4]; // First island islandStates[0][0] = 'c'; islandStates[0][1] = 'g'; islandStates[0][2] = 'w'; islandStates[0][3] = 't'; // Second island islandStates[1][0] = ' '; islandStates[1][1] = ' '; islandStates[1][2] = ' '; islandStates[1][3] = ' ';
Option D: A struct
You can also write a struct to store the island states and contain the functions to intelligently move characters around
Game loop
You should have a standard game loop like this:
bool done = false; while ( !done ) { // Program contents }
Subtask: Display current positions
At the beginning of the loop, display each character's position.
You will probably want a function to do this.
ISLAND 1: TRAVELLER CABBAGE GOAT WOLF ISLAND 2:
Pseudocode:
The implementation here will depend on whether you're storing positions in arrays or integer variables.
function DisplayPositions( islands ): output "ISLAND 1: " for each item in island1: display value output "ISLAND 2: " for each item in island1: display value
Subtask: Get user input
Then ask the user whether they want to...
coutGet their input, and decide how to handle each scenario.
Pseudocode:
In a function, you can display the menu options, get the user's input, and return that value.
function GetInput(): display "1. Move traveler" display "2. Move cabbage" display "3. Move goat" display "4. Move wolf" get user input as an integer return user inputSubtask: Moving characters
You will probably want to implement a function that will handle checking if a character can move, and moving it to the other island. How you do this depends on how you're storing the character positions.
1. Go to other island
For this choice, only the Traveller moves between islands. If the traveller is on island 0, they should move to island 1, and vice versa.
2. Move cabbage/goat/wolf
For this choice, make sure to first check if the traveller and the item-to-move are on the same island. If they are NOT, display an error message and ignore the command.
If they ARE on the same island, move both that item and the traveller to the opposite island.
Pseudocode:
function MoveCharacter( islands, characterToMove ): if ( character position == traveler position ): # Can move the character if ( character position == island 1 ): move character to island 2 move traveler to island 2 else: move character to island 1 move traveler to island 1 else: display "Error: Cannot move because traveler is on different island!"Subtask: Checking win/lose state
You will probably want this to be a function as well.
After the player has chosen their move, make sure to check if the cabbage+goat are on the same island, but the traveller isn't, OR check if the goat+wolf are on the same island, but the traveller isn't. In these cases, the game is over - the player loses.
Also, check to see if the cabbage, wolf, goat, and traveller are all on the same island. If this is the case, then the game is over - the player wins.
Pseudocode:
function GameOver( islands ): if ( goat position == wolf position and goat position != traveler position ): display "The wolf and goat were left alone - the wolf ate the goat. GAME OVER." return true else if ( goat position == cabbage position and goat position != traveler position ): display "The goat and the cabbage were left alone - the goat ate the cabbage. GAME OVER." return true else return falsefunction GameWon( islands ): if ( traveler position == island 2 and cabbage position == island 2 and goat position == island 2 and wolf position == island 2 ): return true else return false
CAUsers svc-student DesktoplCabb..- Island1: cg w t Is land 2 Traveller is on island 1. Go to other island 2. Move Cabbage 3. Move Goat 4. Move Wol Island 1: c w Island 2: g t Traveller is on island 1 1. Go to other island 2. Move Cabbage 3. Move Goat 4. Move Wol Island1: ct w Island 2: g Traveller is on island 1. Go to other island 2. Move Cabbage 3. Move Goat 4. Move Wol Island 1 c Island 2: g w t Traveller is on island 1 1. Go to other island 2. Move Cabbage 3. Move Goat 4. Move Wol THE WOLF ATE THE GOAT Game over Bye
Step by Step Solution
There are 3 Steps involved in it
Step: 1
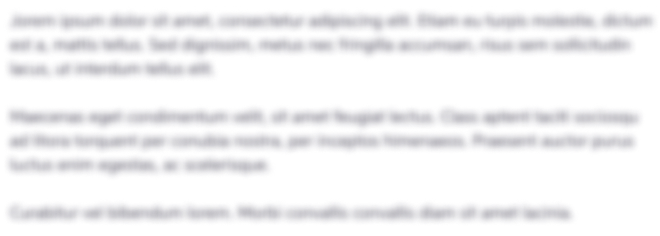
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started