Question
Java Programming Assignment: In this programming assignment, you'll write a small simulation that involves three types of entities: boats, cars, and airplanes. These three entities
Java Programming Assignment:
In this programming assignment, you'll write a small simulation that involves three types of entities: boats, cars, and airplanes. These three entities are, of course, types of vehicles, which can move at specific speeds, but only in left-right, up-down directions on a rectangular grid. Boats are special vehicles that can travel only on water. Cars are special vehicles that can travel only on roads. Airplanes are special vehicles that can only travel between two airports.
The vehicles should be implemented as a class hierarchy, with an abstract base class Vehicle, which keeps track of the vehicle's speed and (x, y) location on the grid. The Vehicle class has a constructor that initializes the vehicle's speed and location on the grid. The vehicle class has getter methods for its internal data items, but no setter methods. The Vehicle class also has a move() method, which is abstract.
Boat, Car, and Airplane are sub-classes of Vehicle. The Boat class has an internal data item which defines the boats' direction of travel (either left, right, up or down). The Car has an internal data item which also defines the car's direction of travel (either left-up or right-down). The Airplane has two internal data items which are the coordinates of the origin and destination airports.
The rectangular grid is also represented as a class, called Grid. It's internal data are the size of the grid as an (x, y) pair, the location of a body of water (as a pair of x values representing the left and right boundaries and a pair of y values representing the up and down boundaries. the location of a road (an array of contiguous (x,y) values), and the locations of two airports as (x, y) values. The Grid class has a constructor to initialize the grid and its other component. See the figure below for an example (Note: you do not have to implement the grid graphically; you need only use Java arrays, although the ArrayList class could be used to keep track of the roads).:
The figure shows a 55 x 40 grid. The boundaries of the blue body of water are: left 5, right 14, up 34, down 30. The sequence of locations that form the red road are (20, 15), (21, 15), (22, 15), (22, 16), (22, 17), (22, 18), (23, 18), (24, 18), (24, 19), (24, 20), (24, 21), (24, 22), (24, 23), and (25, 23). The green airports are located at (5, 5) and (40, 30). The size of the grid, the location of the body of water, the road, and the airports are initialized using parameters given to the Grid class constructor. You to not need to verify that the locations for the road are contiguous; your Grid class can assume this. You may also assume that the airports, the road and the body of water do not overlap. Also assume that road will always move right and up from their initial left-most position.
Below are the required interfaces for the four classes described above:
Vehicle: abstract base class
public Vehicle(Grid grid, int speed, int xLoc, int yLoc)
An implemented constructor that initializes the speed and location components of the vehicle, along with the grid to be used.
public Grid getGrid()
A getter method for the grid.
public int getSpeed()
A getter method for the vehicle's speed.
public int [] getLoc()
A getter method for the vehicle's location, with the X coordinate at index 0 of the returned array and the Y coordinate at index 1
public void setXLoc(int x)
A setter method for the X coordinate. Should throw an exception if the value is outside the grid boundaries.
public void setYLoc(int y)
A setter method for the Y coordinate. Should throw an exception if the value is outside the grid boundaries.
public abstract void move() throws Exception
An abstract method to be implemented in the base classes. The implementations will probably use functions (setXLoc() and setYLoc() of Vehicle) that throw exceptions; if the abstract method does not declare that it throws exceptions, the implementation in the sub-classes can't either.
public String toString()
An override of the toString method that returns a String version of the Vehicle data.
Boat sub-class of Vehicle
public Boat(Grid grid, int speed, int xLoc, int yLoc, int direction)
A constructor for boat. The direction parameter sets the direction of the boat: 0 for left, 1 for up, 2 for right, and 3 for down. Boat checks that the speed is in the range 1 to 3, inclusive and throws an exception otherwise. You may assume that the location given in the parameters is in the body of water.
public String getDirectionString()
A getter method for the boat's direction, returned as a String for convenience.
public int getDirection()
A getter metod for the boat's direction as the stored integer.
public void move()
Boat's implementation of move (see below).
public String toString()
An override of the toString method that returns a String version of the Boat data (including the Vehicle instance).
Car sub-class of Vehicle
public Car(Grid grid, int speed, int xLoc, int yLoc, int direction)
A constructor for Car, The direction parameter sets the direction of the car: 0 for right-up and 1 for left-down. Car checks that the speed is between 3 and 8, inclusive and throws an exception otherwise. You may assume that the location given is one of the road elements.
public String getDirectionString()
A getter method for the boat's direction, returned as a String for convenience.
public int getDirection()
A getter method for the boat's direction as the stored integer.
public void move()
Car's implementation of move (see below).
public String toString()
An override of the toString method that returns a String version of the Car data (including the Vehicle instance).
Airplane sub-class of Vehicle
public Airplane(Grid grid, int speed, int xLoc, int yLoc, int [] origin, int [] destination)
A constructor for Airplane. The last two parameters are for the (x,y) coordinates of the origin and destination airports. Airplane checks that the speed is between 10 and 15, inclusive, and throws an exception otherwise. You may assume that the origin and destination parameters contain the coordinates of the airports in the grid and that they are different.
public int [] getOrigin()
A getter method for the coordinates of the origin airport.
public int [] getDestination()
A get method for the coordinates of the destination airport.
public void move()
Airplane's implementation of move (see below).
public String toString()
An override of the toString method that returns a String version of the Airplane data (including the Vehicle instance).
Grid
public Grid(int xSize, int ySize, int [] water, int [][] road, int [] airport1, int [] airport2)
A constructor for grid with parameters for the size of the grid, the location of the left, right, up, and down
boundaries of the body of water (as a four element array), the location of the road as an array of (x,y) pairs, and the location of the two airports as two element arrays.
public int getXSize()
A getter method that returns the X axis size of the grid.
public int getYSize()
A getter method that returns the Y axis size of the grid
public int getWaterLeft()
A getter method that returns the left boundary of the body of water. getWaterLeft() would return 5 in our example.
public int getWaterRight()
A getter method that returns the right boundary of the body of water. getWaterRight() would return 5 in our example.
public int getWaterUp()
A getter method that returns the up boundary of the body of water. getWaterUp() would return 34 in our example.
public int getWaterDown()
A getter method that returns the down boundary of the body of water. getWaterDown() would return 30 in our example.
public int [] getRoadLeft()
A getter method that returns the (x,y) coordinates for the leftmost element of the road ((20,15) in the example).
public int [] getRoadRight()
A getter method that returns the (x,y) coordinates for the rightmost element of the road ((25, 23) in the example).
public int [] getRoad(int i)
A getter method that returns the (x, y) coordinates for the ith element of the road, zero-based. getRoad(4) would return (22, 17).
public int getRoadIndex(int [] coordinates)
A getter method that returns the index (zero-based) for the road element with the (x,y) coordinates or -1 if the coordinates are not part of the road.
public int getRoadLength()
Returns the number of elements in the road.
public int [] getAirport1()
A getter method that returns the (x,y) coordinates for airport 1 in the grid.
public int [] getAirport2()
A getter method that returns the (x,y) coordinates for airport 2 in the grid.
You should implement your own exceptions for the constructors and the setXloc and setYLoc methods to call. You may call them anything you like, but match the standard conventions for exception names. They should be checked exceptions.
Implementations of the move() method
The move() method is implemented differently in each of the Vehicle sub-classes. This section describes the how each of the vehicle's move() method should work.
Boat: Suppose that speed is s. In each call to move(), the boat method moves s blocks in what ever direction it is traveling, unless that is past the boundary of the body of water for the direction that it is traveling. If so, the boat reverses direction, remains at the boundary. In our example, if the boat is traveling at speed 3 at (6, 7) heading down, a call to move() changes the boat's location to (6, 4), which is past the down boundary. Therefore, the location is set to (6, 5) and the direction switches to up. If the speed is 2 with the boat at (6,7), heading down, move() changes the location to (6, 5).
Car: Let the car's speed be s. In each call to move(), the car moves s road elements in the direction its traveling, unless it moves past the end of the road for the direction it is traveling. It this case, the car reverses direction at the end of the road. For example, if the is traveling left-down and the car is current at road element 3(with coordinates (22, 16) with speed 5, move() notes that this will move past the end of the road and sets the location to (20, 15) and reverses the direction to right-up. If the car is at road element 3 traveling left-down at speed 3, move() changes the location to (21, 15).
Airplane: Let the airplane's speed be s. The airplane moves s blocks on the x axis, toward the destination airport until it meets or passes the destination airport's x coordinates. Subsequent calls to move() move s blocks along the y axis until the destination airport's y coordinate is met or past. The airplane then switches the origin and destination airports. For example, suppose the speed of the airplane is 15 and the destination airport is at (40, 30) and it is starting at the airport at (5, 5). The first call to move() changes the airplane location to (20, 5). The next call to move() changes the location to (35, 5). The next call to move() changes the location to (40, 5), since destination airports x coordinate is passed. The next call to move() changes the location to (40, 15). The next call to move changes the location to (40, 30) and since the y coordinate of the destination airport is met, (5, 5) becomes the destination airport.
Deliverables
I will provide a sample driver program for your classes. It is important that your classes match the names and method signatures that I have outline in this description. Your classes should be part of a package called vehiclesim.
Template for VehicleSim and DisplayGrids:
1)VehicleSim
import vehiclesim.*;
public class VehicleSim {
static String coords2Str(int [] c) { if(c.length != 2) return null; return "(" + c[0] + ", " + c[1] + ")"; }
public static void main(String [] args) { int [] water = {50, 90, 80, 40}; // left, right, up, down water boundaries int [][] road = { {10, 5}, // road element 0 going right {11, 5}, // road element 1 {12, 5}, // road element 2 {13, 5}, // road element 3 {13, 6}, // road element 4 going up {13, 7}, // road element 5 {13, 8}, // road element 6 {14, 8}, // road element 7 going right {15, 8}, // road element 8 {16, 8}, // road element 9 {17, 8}, // road element 10 {17, 9}, // road element 11 going up {17, 10}, // road element 12 {17, 11}, // road element 13 {18, 11}, // road element 14 going right {19, 11}, // road element 15 {19, 12}, // road element 16 {19, 13}, // road element 17 going up {19, 14} // road element 18 }; // end of road coordinates
int [] airport1 = {5, 5}; int [] airport2 = {100, 100};
Grid grid = new Grid(150, 160, water, road, airport1, airport2); System.out.println("Grid size is " + grid.getXSize() + "x" + grid.getYSize()); System.out.println(); System.out.println("Water is at left[" + grid.getWaterLeft() + "] right[" + grid.getWaterRight() + "] up[" + grid.getWaterUp() + "] down[" + grid.getWaterDown() + "]"); System.out.println(); System.out.println("The road has " + grid.getRoadLength() + " elements."); System.out.println("The left-most road element is at " + coords2Str(grid.getRoadLeft()) + "."); System.out.println("The right-most road element is at " + coords2Str(grid.getRoadRight()) + "."); int [] testElement = { 17, 8}; System.out.println("The road element at (" + testElement[0] + ", " + testElement[1] + ") has is road element index " + grid.getRoadIndex(testElement) + "."); testElement[0] = 180; System.out.println("The road element at (" + testElement[0] + ", " + testElement[1] + ") has is road element index " + grid.getRoadIndex(testElement) + "."); System.out.println("The road elements in order are :"); for(int i = 0; i 0 && i % 7 == 0) System.out.println(); } System.out.println(); System.out.println(); System.out.println("Airport 1 is at coordinates " + coords2Str(grid.getAirport1()) + "."); System.out.println("Airport 2 is at coordinates " + coords2Str(grid.getAirport2()) + ".");
System.out.println();
Boat b = null; Car c = null; Airplane a = null; try { b = new Boat(grid, 3, 65, 65, 0); c = new Car(grid, 5, 13, 6, 0); a = new Airplane(grid, 12, 5, 5, grid.getAirport1(), grid.getAirport2()); } catch (Exception e) { System.out.println(e.getMessage()); if(e.getClass().getSimpleName() == "BadVehicleParametersException") ((BadVehicleParametersException) (e)).getBadParameter(); e.printStackTrace(); }
System.out.println("Created a boat: " + b); System.out.println("Created a car: " + c); System.out.println("Created an airplane: " + a);
int cycles = 50; System.out.println("Run vehicle simulation with these objects for " + cycles + " cycles: ");
for(int i = 0; i
} }
2)DisplayGrids:
import java.util.Arrays; import java.util.Scanner; import java.util.ArrayList; import javafx.application.Application; import javafx.application.Platform; import javafx.stage.Stage; import javafx.scene.Scene; import javafx.scene.control.*; import javafx.scene.layout.*; import javafx.scene.shape.*; import javafx.geometry.*; import javafx.scene.paint.*; import javafx.event.*;
public class DisplayGrids extends Application {
private static final String [] WTR_BNDRS = {"Left", "Right", "Up", "Down"}; private static final int LEFT = 0; private static final int RIGHT = 1; private static final int UP = 2; private static final int DOWN = 3; private static final String [] AIRPORTS = {"Airport 1 x,y format", "Airport 2 x,y format"}; private static final int AIRPORT1 = 0; private static final int AIRPORT2 = 1; private static final String ROAD_ELEMENTS = "Road Elements: enter pairs of x,y coordinates," + " separated by colons: 1, 2 : 3, 4 : 5, 6"; private static final String [] GRID_SIZES = {"X Size", "Y Size"}; private static final int XSIZE = 0; private static final int YSIZE = 1; private static final String [] CONTROLS = {"Clear", "Draw Grid", "Quit"}; private static final int CLEAR = 0; private static final int DRAWGRID = 1; private static final int QUIT = 2; private static final int DEFAULT_GRID_WIDTH = 500; private static final int DEFAULT_GRID_HEIGHT = 500;
private static int[][] parseRoadElements(String re) { //System.out.println("Parsing string " + re); Scanner in = new Scanner(re); in.useDelimiter("[,: ]"); ArrayList public static int[][] parseAirportCoords(String ac) { int [][] a = parseRoadElements(ac); if(a.length != 2) return null; return a; } public void start(Stage s) { BorderPane bpMainForm = new BorderPane(); VBox vbRoadElements = new VBox(); Label lbRoadElements = new Label(ROAD_ELEMENTS); TextArea taRoadElements = new TextArea(); vbRoadElements.getChildren().addAll(lbRoadElements, taRoadElements); VBox vbAirports = new VBox(); Label [] lbAirports = new Label[2]; TextField [] tfAirports = new TextField[2]; for(int i = 0; i VBox vbWater = new VBox(); Label [] lbWater = new Label[4]; TextField [] tfWater = new TextField[4]; for(int i = 0; i VBox vbGridSizes = new VBox(); Label [] lbGridSizes = new Label[2]; TextField [] tfGridSizes = new TextField[2]; for(int i = 0; i VBox vbAirportsGridSizes = new VBox(); vbAirportsGridSizes.getChildren().addAll(vbAirports, vbGridSizes); GridPane gpGrid = new GridPane(); gpGrid.setPrefWidth(DEFAULT_GRID_WIDTH); gpGrid.setPrefHeight(DEFAULT_GRID_HEIGHT); HBox hbControls = new HBox(); Button btClear = new Button(CONTROLS[CLEAR]); Button btDrawGrid = new Button(CONTROLS[DRAWGRID]); Button btQuit = new Button(CONTROLS[QUIT]); hbControls.getChildren().addAll(btClear, btDrawGrid, btQuit); bpMainForm.setTop(vbRoadElements); bpMainForm.setLeft(vbWater); bpMainForm.setRight(vbAirportsGridSizes); bpMainForm.setCenter(gpGrid); bpMainForm.setBottom(hbControls); btQuit.setOnAction(e -> System.exit(1)); btClear.setOnAction(e -> { taRoadElements.clear(); for(int i = 0; i { int xSize = Integer.parseInt(tfGridSizes[XSIZE].getText()); int ySize = Integer.parseInt(tfGridSizes[YSIZE].getText()); Button [][] g = new Button[xSize][ySize]; gpGrid.getChildren().clear(); for(int i = 0; i int left = Integer.parseInt(tfWater[LEFT].getText()); int right = Integer.parseInt(tfWater[RIGHT].getText()); int up = Integer.parseInt(tfWater[UP].getText()); int down = Integer.parseInt(tfWater[DOWN].getText()); if(left xSize -1 || left >= right) { left = 0; tfWater[LEFT].setText(Integer.toString(left)); } if(right xSize -1) { right = (left + xSize) / 2; tfWater[RIGHT].setText(Integer.toString(right)); } if(down ySize -1 || down >= up) { down = 0; tfWater[DOWN].setText(Integer.toString(down)); } if(up ySize -1) { up = (down + ySize) / 2; tfWater[UP].setText(Integer.toString(up)); } int temp = ySize - up - 1; down = temp + (up - down); up = temp; for(int i = left; i g[re[i][0]][ySize-re[i][1] - 1].setStyle("-fx-background-color: Red"); } String ac = tfAirports[AIRPORT1].getText() + ":" + tfAirports[AIRPORT2].getText(); int [][]a = parseAirportCoords(ac); if(a != null) { g[a[0][0]][ySize - a[0][1] - 1].setStyle("-fx-background-color: Green"); g[a[1][0]][ySize - a[1][1] - 1].setStyle("-fx-background-color: Green"); } }); Scene scene = new Scene(bpMainForm); s.setTitle("Display Vehicle Simulation Grids"); s.setScene(scene); s.show(); } } Final result should be same as below: Grid size is 150x160 Water is at left[50] right[90] up[80] down[40] The road has 19 elements. The left-most road element is at (10, 5). The right-most road element is at (19, 14). The road element at (17, 8) has is road element index 10. The road element at (180, 8) has is road element index -1. The road elements in order are : (10, 5), (11, 5), (12, 5), (13, 5), (13, 6), (13, 7), (13, 8), (14, 8), (15, 8), (16, 8), (17, 8), (17, 9), (17, 10), (17, 11), (18, 11), (19, 11), (19, 12), (19, 13), (19, 14) Airport 1 is at coordinates (5, 5). Airport 2 is at coordinates (100, 100). Created a boat: speed[3] X[65] Y[65] direction[left] Created a car: speed[5] X[13] Y[6] direction[right-up] Created an airplane: speed[12] X[5] Y[5] origin[X[5] Y[5]] destination[X[100] Y[100]] Run vehicle simulation with these objects for 50 cycles: Boat: speed[3] X[65] Y[65] direction[left] at time 0 Car: speed[5] X[13] Y[6] direction[right-up] on road element 4 at (13, 6) at time 0 Aiplane: speed[12] X[5] Y[5] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 0 Boat: speed[3] X[62] Y[65] direction[left] at time 1 Car: speed[5] X[16] Y[8] direction[right-up] on road element 9 at (16, 8) at time 1 Aiplane: speed[12] X[17] Y[5] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 1 Boat: speed[3] X[59] Y[65] direction[left] at time 2 Car: speed[5] X[18] Y[11] direction[right-up] on road element 14 at (18, 11) at time 2 Aiplane: speed[12] X[29] Y[5] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 2 Boat: speed[3] X[56] Y[65] direction[left] at time 3 Car: speed[5] X[19] Y[14] direction[left-down] on road element 18 at (19, 14) at time 3 Aiplane: speed[12] X[41] Y[5] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 3 Boat: speed[3] X[53] Y[65] direction[left] at time 4 Car: speed[5] X[17] Y[11] direction[left-down] on road element 13 at (17, 11) at time 4 Aiplane: speed[12] X[53] Y[5] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 4 Boat: speed[3] X[50] Y[65] direction[right] at time 5 Car: speed[5] X[15] Y[8] direction[left-down] on road element 8 at (15, 8) at time 5 Aiplane: speed[12] X[65] Y[5] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 5 Boat: speed[3] X[53] Y[65] direction[right] at time 6 Car: speed[5] X[13] Y[5] direction[left-down] on road element 3 at (13, 5) at time 6 Aiplane: speed[12] X[77] Y[5] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 6 Boat: speed[3] X[56] Y[65] direction[right] at time 7 Car: speed[5] X[10] Y[5] direction[right-up] on road element 0 at (10, 5) at time 7 Aiplane: speed[12] X[89] Y[5] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 7 Boat: speed[3] X[59] Y[65] direction[right] at time 8 Car: speed[5] X[13] Y[7] direction[right-up] on road element 5 at (13, 7) at time 8 Aiplane: speed[12] X[100] Y[5] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 8 Boat: speed[3] X[62] Y[65] direction[right] at time 9 Car: speed[5] X[17] Y[8] direction[right-up] on road element 10 at (17, 8) at time 9 Aiplane: speed[12] X[100] Y[17] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 9 Boat: speed[3] X[65] Y[65] direction[right] at time 10 Car: speed[5] X[19] Y[11] direction[right-up] on road element 15 at (19, 11) at time 10 Aiplane: speed[12] X[100] Y[29] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 10 Boat: speed[3] X[68] Y[65] direction[right] at time 11 Car: speed[5] X[19] Y[14] direction[left-down] on road element 18 at (19, 14) at time 11 Aiplane: speed[12] X[100] Y[41] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 11 Boat: speed[3] X[71] Y[65] direction[right] at time 12 Car: speed[5] X[17] Y[11] direction[left-down] on road element 13 at (17, 11) at time 12 Aiplane: speed[12] X[100] Y[53] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 12 Boat: speed[3] X[74] Y[65] direction[right] at time 13 Car: speed[5] X[15] Y[8] direction[left-down] on road element 8 at (15, 8) at time 13 Aiplane: speed[12] X[100] Y[65] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 13 Boat: speed[3] X[77] Y[65] direction[right] at time 14 Car: speed[5] X[13] Y[5] direction[left-down] on road element 3 at (13, 5) at time 14 Aiplane: speed[12] X[100] Y[77] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 14 Boat: speed[3] X[80] Y[65] direction[right] at time 15 Car: speed[5] X[10] Y[5] direction[right-up] on road element 0 at (10, 5) at time 15 Aiplane: speed[12] X[100] Y[89] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 15 Boat: speed[3] X[83] Y[65] direction[right] at time 16 Car: speed[5] X[13] Y[7] direction[right-up] on road element 5 at (13, 7) at time 16 Aiplane: speed[12] X[100] Y[100] origin[X[100] Y[100]] destination[X[5] Y[5]] at time 16 Boat: speed[3] X[86] Y[65] direction[right] at time 17 Car: speed[5] X[17] Y[8] direction[right-up] on road element 10 at (17, 8) at time 17 Aiplane: speed[12] X[88] Y[100] origin[X[100] Y[100]] destination[X[5] Y[5]] at time 17 Boat: speed[3] X[89] Y[65] direction[right] at time 18 Car: speed[5] X[19] Y[11] direction[right-up] on road element 15 at (19, 11) at time 18 Aiplane: speed[12] X[76] Y[100] origin[X[100] Y[100]] destination[X[5] Y[5]] at time 18 Boat: speed[3] X[90] Y[65] direction[left] at time 19 Car: speed[5] X[19] Y[14] direction[left-down] on road element 18 at (19, 14) at time 19 Aiplane: speed[12] X[64] Y[100] origin[X[100] Y[100]] destination[X[5] Y[5]] at time 19 Boat: speed[3] X[87] Y[65] direction[left] at time 20 Car: speed[5] X[17] Y[11] direction[left-down] on road element 13 at (17, 11) at time 20 Aiplane: speed[12] X[52] Y[100] origin[X[100] Y[100]] destination[X[5] Y[5]] at time 20 Boat: speed[3] X[84] Y[65] direction[left] at time 21 Car: speed[5] X[15] Y[8] direction[left-down] on road element 8 at (15, 8) at time 21 Aiplane: speed[12] X[40] Y[100] origin[X[100] Y[100]] destination[X[5] Y[5]] at time 21 Boat: speed[3] X[81] Y[65] direction[left] at time 22 Car: speed[5] X[13] Y[5] direction[left-down] on road element 3 at (13, 5) at time 22 Aiplane: speed[12] X[28] Y[100] origin[X[100] Y[100]] destination[X[5] Y[5]] at time 22 Boat: speed[3] X[78] Y[65] direction[left] at time 23 Car: speed[5] X[10] Y[5] direction[right-up] on road element 0 at (10, 5) at time 23 Aiplane: speed[12] X[16] Y[100] origin[X[100] Y[100]] destination[X[5] Y[5]] at time 23 Boat: speed[3] X[75] Y[65] direction[left] at time 24 Car: speed[5] X[13] Y[7] direction[right-up] on road element 5 at (13, 7) at time 24 Aiplane: speed[12] X[5] Y[100] origin[X[100] Y[100]] destination[X[5] Y[5]] at time 24 Boat: speed[3] X[72] Y[65] direction[left] at time 25 Car: speed[5] X[17] Y[8] direction[right-up] on road element 10 at (17, 8) at time 25 Aiplane: speed[12] X[5] Y[88] origin[X[100] Y[100]] destination[X[5] Y[5]] at time 25 Boat: speed[3] X[69] Y[65] direction[left] at time 26 Car: speed[5] X[19] Y[11] direction[right-up] on road element 15 at (19, 11) at time 26 Aiplane: speed[12] X[5] Y[76] origin[X[100] Y[100]] destination[X[5] Y[5]] at time 26 Boat: speed[3] X[66] Y[65] direction[left] at time 27 Car: speed[5] X[19] Y[14] direction[left-down] on road element 18 at (19, 14) at time 27 Aiplane: speed[12] X[5] Y[64] origin[X[100] Y[100]] destination[X[5] Y[5]] at time 27 Boat: speed[3] X[63] Y[65] direction[left] at time 28 Car: speed[5] X[17] Y[11] direction[left-down] on road element 13 at (17, 11) at time 28 Aiplane: speed[12] X[5] Y[52] origin[X[100] Y[100]] destination[X[5] Y[5]] at time 28 Boat: speed[3] X[60] Y[65] direction[left] at time 29 Car: speed[5] X[15] Y[8] direction[left-down] on road element 8 at (15, 8) at time 29 Aiplane: speed[12] X[5] Y[40] origin[X[100] Y[100]] destination[X[5] Y[5]] at time 29 Boat: speed[3] X[57] Y[65] direction[left] at time 30 Car: speed[5] X[13] Y[5] direction[left-down] on road element 3 at (13, 5) at time 30 Aiplane: speed[12] X[5] Y[28] origin[X[100] Y[100]] destination[X[5] Y[5]] at time 30 Boat: speed[3] X[54] Y[65] direction[left] at time 31 Car: speed[5] X[10] Y[5] direction[right-up] on road element 0 at (10, 5) at time 31 Aiplane: speed[12] X[5] Y[16] origin[X[100] Y[100]] destination[X[5] Y[5]] at time 31 Boat: speed[3] X[51] Y[65] direction[left] at time 32 Car: speed[5] X[13] Y[7] direction[right-up] on road element 5 at (13, 7) at time 32 Aiplane: speed[12] X[5] Y[5] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 32 Boat: speed[3] X[50] Y[65] direction[right] at time 33 Car: speed[5] X[17] Y[8] direction[right-up] on road element 10 at (17, 8) at time 33 Aiplane: speed[12] X[17] Y[5] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 33 Boat: speed[3] X[53] Y[65] direction[right] at time 34 Car: speed[5] X[19] Y[11] direction[right-up] on road element 15 at (19, 11) at time 34 Aiplane: speed[12] X[29] Y[5] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 34 Boat: speed[3] X[56] Y[65] direction[right] at time 35 Car: speed[5] X[19] Y[14] direction[left-down] on road element 18 at (19, 14) at time 35 Aiplane: speed[12] X[41] Y[5] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 35 Boat: speed[3] X[59] Y[65] direction[right] at time 36 Car: speed[5] X[17] Y[11] direction[left-down] on road element 13 at (17, 11) at time 36 Aiplane: speed[12] X[53] Y[5] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 36 Boat: speed[3] X[62] Y[65] direction[right] at time 37 Car: speed[5] X[15] Y[8] direction[left-down] on road element 8 at (15, 8) at time 37 Aiplane: speed[12] X[65] Y[5] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 37 Boat: speed[3] X[65] Y[65] direction[right] at time 38 Car: speed[5] X[13] Y[5] direction[left-down] on road element 3 at (13, 5) at time 38 Aiplane: speed[12] X[77] Y[5] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 38 Boat: speed[3] X[68] Y[65] direction[right] at time 39 Car: speed[5] X[10] Y[5] direction[right-up] on road element 0 at (10, 5) at time 39 Aiplane: speed[12] X[89] Y[5] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 39 Boat: speed[3] X[71] Y[65] direction[right] at time 40 Car: speed[5] X[13] Y[7] direction[right-up] on road element 5 at (13, 7) at time 40 Aiplane: speed[12] X[100] Y[5] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 40 Boat: speed[3] X[74] Y[65] direction[right] at time 41 Car: speed[5] X[17] Y[8] direction[right-up] on road element 10 at (17, 8) at time 41 Aiplane: speed[12] X[100] Y[17] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 41 Boat: speed[3] X[77] Y[65] direction[right] at time 42 Car: speed[5] X[19] Y[11] direction[right-up] on road element 15 at (19, 11) at time 42 Aiplane: speed[12] X[100] Y[29] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 42 Boat: speed[3] X[80] Y[65] direction[right] at time 43 Car: speed[5] X[19] Y[14] direction[left-down] on road element 18 at (19, 14) at time 43 Aiplane: speed[12] X[100] Y[41] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 43 Boat: speed[3] X[83] Y[65] direction[right] at time 44 Car: speed[5] X[17] Y[11] direction[left-down] on road element 13 at (17, 11) at time 44 Aiplane: speed[12] X[100] Y[53] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 44 Boat: speed[3] X[86] Y[65] direction[right] at time 45 Car: speed[5] X[15] Y[8] direction[left-down] on road element 8 at (15, 8) at time 45 Aiplane: speed[12] X[100] Y[65] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 45 Boat: speed[3] X[89] Y[65] direction[right] at time 46 Car: speed[5] X[13] Y[5] direction[left-down] on road element 3 at (13, 5) at time 46 Aiplane: speed[12] X[100] Y[77] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 46 Boat: speed[3] X[90] Y[65] direction[left] at time 47 Car: speed[5] X[10] Y[5] direction[right-up] on road element 0 at (10, 5) at time 47 Aiplane: speed[12] X[100] Y[89] origin[X[5] Y[5]] destination[X[100] Y[100]] at time 47 Boat: speed[3] X[87] Y[65] direction[left] at time 48 Car: speed[5] X[13] Y[7] direction[right-up] on road element 5 at (13, 7) at time 48 Aiplane: speed[12] X[100] Y[100] origin[X[100] Y[100]] destination[X[5] Y[5]] at time 48 Boat: speed[3] X[84] Y[65] direction[left] at time 49 Car: speed[5] X[17] Y[8] direction[right-up] on road element 10 at (17, 8) at time 49 Aiplane: speed[12] X[88] Y[100] origin[X[100] Y[100]] destination[X[5] Y[5]] at time 49 Final state of boat after 50 cycles: speed[3] X[81] Y[65] direction[left] Final state of car after 50 cycles: speed[5] X[19] Y[11] direction[right-up] Final state of airplane after 50 cycles speed[12] X[76] Y[100] origin[X[100] Y[100]] destination[X[5] Y[5]]
Step by Step Solution
There are 3 Steps involved in it
Step: 1
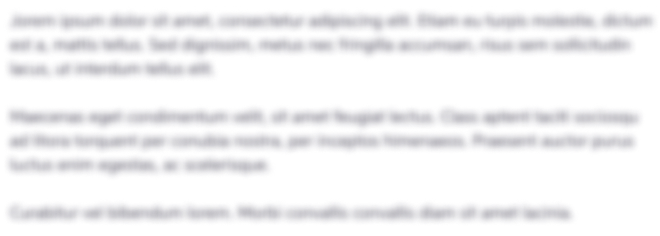
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started