Question
Java programming FoodItem class= protected int itemCode; protected String itemName; protected float itemPrice; protected int itemQuantityinStock; protected float itemCost; class Preserve extends FoodItem; private int
-
- Java programming
- FoodItem class= protected int itemCode; protected String itemName; protected float itemPrice; protected int itemQuantityinStock; protected float itemCost;
- class Preserve extends FoodItem; private int jarSize;
- class Vegetable extends FoodItem; private String farmName;
- class Fruit extends FoodItem; private String orchardName;
- class Sweetener extends FoodItems; check the output and decide about data members
- class Inventory; protected static int numItems; protected ArrayList
inventory; - All data members must never be public. You should make them all private unless they are in a base class AND they are needed in the child classes
- For this assignment, we will limit the inventory array to a maximum of 20 entries
- Notes on the FoodItem class:
Method Additional Information toString Displays the all data members in the class
addItem Reads from the Scanner object passed in and fills the data member fields of the class with valid data; Method returns true if program successfully reads in all fields, otherwise returns false
updateItem Updates the quantity field by amount (note amount could be positive or negative); Method returns true if successful, otherwise returns false Note: itemQuantityInStock field can never be less than 0.
isEqual Method returns true if the itemCode of the object being acted on and the item object parameter are the same value inputCode Reads a valid itemCode from the Scanner object and returns true/false if successful - Notes on the Fruit, Vegetable and Preserve classes, see above table for additional information on the methods with the same name.
- Notes on the Inventory class:
Method Additional Information addItem Adds an item to the inventory array (uses polymorphism to call addItem method in the correct derived FoodItem class for input of the fields of the FoodItem) alreadyExists Returns the index of a FoodItem in the inventory array with the same itemCode as the FoodItem object in the parameter list, else returns -1 updateQuantity Reads in an itemCode to update and quantity to update by and updates that item by the input quantity in the inventory array. The boolean parameter is used to denote whether buying operation (true) or selling operation (false) is occurring. Method returns true/false on whether update was successful or not - Notes on Assign1 class:
Method Additional Information main Main method of the program displayMenu Displays the main menu to the console
- Create a console application that will display the following menu repeatedly until the user selects the exit item. Ensure that your program will never crash nor exit in any other circumstances. The menu should be displayed again if an incorrect value is entered (i.e. a number that is not between 1-5 or any other character) after displaying the error message "Incorrect value entered". The menu should look exactly like this:
Please select one of the following: 1: Add Item to Inventory 2: Display Current Inventory 3: Buy Item(s) 4: Sell Item(s) 5: To Exit >
- When '1' is chosen by the user, the user needs to enter additional information. When the user choses to enter a fruit, the program should ask the user which kind and then ask for follow up data as in the following table:
Do you wish to add a fruit(f), vegetable(v), Sweetener(s) or a preserve(p)? If f selected
Enter the code for the item: Enter the name for the item: Enter the quantity for the item: Enter the cost of the item: Enter the sales price of the item: Enter the name of the orchard supplier:
If s selected
Enter the code for the item: Enter the name for the item: Enter the quantity for the item: Enter the cost of the item: Enter the sales price of the item: Enter the name of the farm supplier:
If v selected Enter the code for the item: Enter the name for the item: Enter the quantity for the item: Enter the cost of the item: Enter the sales price of the item: Enter the name of the farm supplier:
If p selected Enter the code for the item: Enter the name for the item: Enter the quantity for the item: Enter the cost of the item: Enter the sales price of the item: Enter the size of the jar in millilitres:
If item code exists Enter the code for the item: Item code already exists
- When '2' is chosen by the user, the content of the inventory array. Starting with the header "Inventory:" and then one item per line in the following format:
For a fruit item Item: price: $ cost: $ orchard supplier: For a vegetable item Item: price: $ cost: $ farm supplier: For a preserve item Item: price: $ cost: $ size: mL - When '3' is chosen by the user, the program will ask the user to enter the code and then the quantity if the code is present in the inventory array. See table for full details:
When code doesn't exist Enter valid item code: Code not found in inventory... Error...could not buy item
When code is found Enter valid item code: Enter valid quantity to buy:
If quantity is not valid Enter valid item code: Enter valid quantity to buy: Invalid quantity... Error...could not buy item
- When '4' is chosen by the user, the program will ask the user to enter the code and then the quantity if the code is present in the inventory array. See table for full details:
When code doesn't exist Enter valid item code: Code not found in inventory... Error...could not sell item
When code is found Enter valid item code: Enter valid quantity to sell:
If quantity is not valid Enter valid item code: Enter valid quantity to sell: Invalid quantity... Error...could not sell item
If quantity too large Enter valid item code: Enter valid quantity to sell: Insufficient stock in inventory... Error...could not sell item
- When '5' is chosen by the user, the message "Exiting..." is displayed and the program terminates.
Sample Output: green is user input
Please select one of the following: 1: Add Item to Inventory 2: Display Current Inventory 3: Buy Item(s) 4: Sell Item(s) 5: To Exit > 2 Inventory: Please select one of the following: 1: Add Item to Inventory 2: Display Current Inventory 3: Buy Item(s) 4: Sell Item(s) 5: To Exit > 3 Error...could not buy item Please select one of the following: 1: Add Item to Inventory 2: Display Current Inventory 3: Buy Item(s) 4: Sell Item(s) 5: To Exit > 4 Error...could not sell item Please select one of the following: 1: Add Item to Inventory 2: Display Current Inventory 3: Buy Item(s) 4: Sell Item(s) 5: To Exit > 1 Do you wish to add a fruit(f), vegetable(v),sweetener(s) or a preserve(p)? w Invalid entry Do you wish to add a fruit(f), vegetable(v),sweetener(s) or a preserve(p)? 1 Invalid entry Do you wish to add a fruit(f), vegetable(v),sweetener(s) or a preserve(p)? f Enter the code for the item: 111 Enter the name for the item: Granny Smith Apple Enter the quantity for the item: 30 Enter the cost of the item: .10 Enter the sales price of the item: .25 Enter the name of the orchard supplier: Apple Orchard Please select one of the following: 1: Add Item to Inventory 2: Display Current Inventory 3: Buy Item(s) 4: Sell Item(s) 5: To Exit > 1 Do you wish to add a fruit(f), vegetable(v), sweetener(s) or a preserve(p)? s Enter the code for the item: 555 Enter the name for the item: Brown Honey Enter the quantity for the item: 40 Enter the cost of the item: .40 Enter the sales price of the item: 0.80 Enter the name of the farm supplier: James Farm Please select one of the following: 1: Add Item to Inventory 2: Display Current Inventory 3: Buy Item(s) 4: Sell Item(s) 5: To Exit > 2 Inventory: Item: 111 Granny Smith Apple 30 price: $0.25 cost: $0.10 orchard supplier: Apple Orchard Item: 555 Brown Honey Sweetener 40 price: $0.80 cost: $0.40 Sweetener supplier: James Farm Please select one of the following: 1: Add Item to Inventory 2: Display Current Inventory 3: Buy Item(s) 4: Sell Item(s) 5: To Exit > 1 Do you wish to add a fruit(f), vegetable(v), sweetener(s) or a preserve(p)? v Enter the code for the item: 222 Enter the name for the item: Orange Carrot Enter the quantity for the item: 100 Enter the cost of the item: .25 Enter the sales price of the item: 1.00 Enter the name of the farm supplier: McDonald's Farm Please select one of the following: 1: Add Item to Inventory 2: Display Current Inventory 3: Buy Item(s) 4: Sell Item(s) 5: To Exit > 1 Do you wish to add a fruit(f), vegetable(v), sweetener(s) or a preserve(p)? p Enter the code for the item: 333 Enter the name for the item: Raspberry Jam Enter the quantity for the item: 10 Enter the cost of the item: 4.50 Enter the sales price of the item: 5.50 Enter the size of the jar in millilitres: 500 Please select one of the following: 1: Add Item to Inventory 2: Display Current Inventory 3: Buy Item(s) 4: Sell Item(s) 5: To Exit > 1 Do you wish to add a fruit(f), vegetable(v),sweetener(s) or a preserve(p)? f Enter the code for the item: ee Invalid code Enter the code for the item: -123 Enter the name for the item: Test Enter the quantity for the item: ee Invalid entry Enter the quantity for the item: -19 Invalid entry Enter the quantity for the item: 20 Enter the cost of the item: -3 Invalid entry Enter the cost of the item: h Invalid entry Enter the cost of the item: 3.4 Enter the sales price of the item: -9 Invalid entry Enter the sales price of the item: e Invalid entry Enter the sales price of the item: ! Invalid entry Enter the sales price of the item: 3.50 Enter the name of the orchard supplier: Test Orchard Please select one of the following: 1: Add Item to Inventory 2: Display Current Inventory 3: Buy Item(s) 4: Sell Item(s) 5: To Exit > 2 Inventory: Item: 111 Granny Smith Apple 30 price: $0.25 cost: $0.10 orchard supplier: Apple Orchard Item: 222 Orange Carrot 100 price: $1.00 cost: $0.25 farm supplier: McDonald's Farm Item: 333 Raspberry Jam 10 price: $5.50 cost: $4.50 size: 500mL Item: -123 Test 20 price: $3.50 cost: $3.40 orchard supplier: Test Orchard Please select one of the following: 1: Add Item to Inventory 2: Display Current Inventory 3: Buy Item(s) 4: Sell Item(s) 5: To Exit > 3 Enter the code for the item: 444 Error...could not buy item Please select one of the following: 1: Add Item to Inventory 2: Display Current Inventory 3: Buy Item(s) 4: Sell Item(s) 5: To Exit > 3 Enter the code for the item: 111 Enter valid quantity to buy: 20 Please select one of the following: 1: Add Item to Inventory 2: Display Current Inventory 3: Buy Item(s) 4: Sell Item(s) 5: To Exit > 4 Enter the code for the item: 333 Enter valid quantity to sell: 15 Error...could not sell item Please select one of the following: 1: Add Item to Inventory 2: Display Current Inventory 3: Buy Item(s) 4: Sell Item(s) 5: To Exit > 4 Enter the code for the item: 333 Enter valid quantity to sell: 9 Please select one of the following: 1: Add Item to Inventory 2: Display Current Inventory 3: Buy Item(s) 4: Sell Item(s) 5: To Exit > 2 Inventory: Item: 111 Granny Smith Apple 50 price: $0.25 cost: $0.10 orchard supplier: Apple Orchard Item: 222 Orange Carrot 100 price: $1.00 cost: $0.25 farm supplier: McDonald's Farm Item: 333 Raspberry Jam 1 price: $5.50 cost: $4.50 size: 500mL Item: -123 Test 20 price: $3.50 cost: $3.40 orchard supplier: Test Orchard Please select one of the following: 1: Add Item to Inventory 2: Display Current Inventory 3: Buy Item(s) 4: Sell Item(s) 5: To Exit > 5 Exiting...
Step by Step Solution
There are 3 Steps involved in it
Step: 1
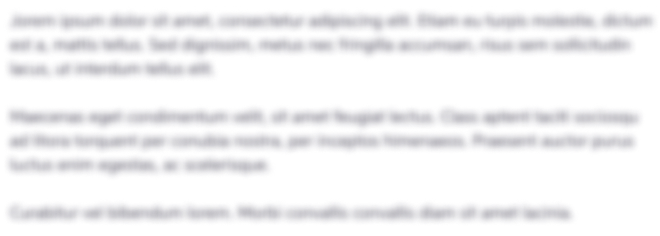
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started