Question
Java Programming Have to complete Task1. I have listed all of my code, the layout for the code as well as the expected output for
Java Programming
Have to complete Task1. I have listed all of my code, the layout for the code as well as the expected output for Task1Results.txt at the very end.
Classroom.java
package org.university.hardware;
import org.university.software.Course;
import org.university.hardware.Department;
import java.util.ArrayList;
public class Classroom {
private String room;
private ArrayList
public Classroom() {
room = "Unknown";
courses = new ArrayList
}
private int[] getTimeSlots()
{
int[] toReturn = new int[30];
int index = 0;
for (int i = 100; i
for (int j = 1; j
toReturn[index] = i + j;
index++;
}
}
return toReturn;
}
public void addCourse(Course aCourse)
{
boolean flag = false;
for (Course crs : courses) {
if (crs.compareSchedules(aCourse)) {
System.out.println(aCourse.getDepartment().getDepartmentName()
+ aCourse.getCourseNumber() + " conflicts with "
+ crs.getDepartment().getDepartmentName() + crs.getCourseNumber()
+ ". Conflicting time slot " + aCourse.getConflictSlots(crs).get(0)
+ ". " + aCourse.getDepartment().getDepartmentName()
+ aCourse.getCourseNumber() + " course cannot be added to "
+ room + "'s Schedule.");
flag = true;
break;
}
}
if (!flag)
{
courses.add(aCourse);
}
}
public void setRoomNumber(String newRoom)
{
room = newRoom;
}
public String getRoomNumber()
{
return room;
}
public void printSchedule() {
int[] timeSlots = getTimeSlots();
for (int time : timeSlots) {
String slot = "";
String className = "";
for (Course crs : courses) {
if (crs.getMeetingTime(time) != "") {
slot = crs.getMeetingTime(time);
className = crs.getDepartment().getDepartmentName()
+ crs.getCourseNumber() + " " + crs.getName();
break;
}
}
if (slot != "")
System.out.println(slot + " " + className);
}
}
}
Department.java
package org.university.hardware;
import org.university.software.Course;
import org.university.people.Student;
import org.university.people.Professor;
import org.university.people.Staff;
import java.util.ArrayList;
public class Department {
private String departmentName;
private ArrayList
private ArrayList
private ArrayList
private ArrayList
public Department()
{
departmentName = "unknown";
Courses = new ArrayList
Students = new ArrayList
Professors = new ArrayList
Staffers = new ArrayList
}
public String getDepartmentName()
{
return departmentName;
}
public void setDepartmentName(String adepartmentname)
{
departmentName = adepartmentname;
}
public void addStaff(Staff stf){
Staffers.add(stf);
if(stf.getDepartment() == null || stf.getDepartment() != this){
stf.setDepartment(this);
}
}
public ArrayList
return Staffers;
}
public void addProfessor(Professor prof){
Professors.add(prof);
if(prof.getDepartment() == null || prof.getDepartment() != this){
prof.setDepartment(this);
}
}
public ArrayList
return Professors;
}
public void addStudent(Student student){
Students.add(student);
if(!student.getDepartment().equals(this))
student.setDepartment(this);
}
public ArrayList
return Students;
}
public void addCourse(Course course){
Courses.add(course);
if(!course.getDepartment().equals(this))
course.setDepartment(this);
}
public ArrayList
return Courses;
}
public void printStudentList(){
for(Student s : Students){
System.out.println(s.getName());
}
}
public void printProfessorList(){
for(Professor p : Professors){
System.out.println(p.getName());
}
}
public void printStaffList(){
for(Staff st : Staffers){
System.out.println(st.getName());
}
}
public void printCourseList(){
for(Course c : Courses){
System.out.println(c.getName() + c.getCourseNumber());
}
}
}
Employee.java
package org.university.people;
public abstract class Employee extends Person {
private double payRate;
public Employee() {
payRate = 0.0;
}
public double getPayRate()
{
return payRate;
}
public void setPayRate(double pr)
{
payRate = pr;
}
public void raise(double percent) {
payRate += (percent / 100.0) * payRate;
}
public abstract double earns();
}
Person.java
package org.university.people;
import java.util.ArrayList; import org.university.software.Course;
public abstract class Person { private String name; private ArrayList
public Person(){ courses = new ArrayList
private int[] getTimeSlots(){ int[] toReturn = new int[30]; int index = 0; for(int i = 100; i
public void setName(String newName){ name = newName; }
public String getName(){ return name; }
public ArrayList
public boolean detectConflict(Course aCourse){ for(Course course : courses){ if(course.compareSchedules(aCourse)){ ArrayList
public void printSchedule(){ for(int time : getTimeSlots()) for(Course crs : courses) if(crs.getMeetingTime(time) != "") System.out.println(crs.getMeetingTime(time) + " " + crs.getDepartment().getDepartmentName() + crs.getCourseNumber() + " " + crs.getName()); }
public abstract void addCourse(Course aCourse); }
Professor.java
package org.university.people;
import org.university.hardware.Department; import org.university.software.Course;
public class Professor extends Employee { private Department dept;
public Professor(){ dept = null; }
public void setDepartment(Department newDept){ dept = newDept; }
public Department getDepartment(){ return dept; }
@Override public double earns() { return 200 * super.getPayRate(); }
@Override public void addCourse(Course aCourse) { if(aCourse.getProfessor() != null){ System.out.println("The professor cannot be assigned to this course" + " because professor " + aCourse.getProfessor().getName() + " is already assigned to the course " + aCourse.getName() + "."); } else if(!detectConflict(aCourse)){ aCourse.setProfessor(this); super.getCourses().add(aCourse); } }
}
Staff.java
package org.university.people; import org.university.software.Course; import org.university.hardware.Department;
public class Staff extends Employee { private Department dept; private double hoursWorked;
public Staff(){ hoursWorked = 0.0; dept = null; }
public void setMonthlyHours(double hours){ hoursWorked = hours; }
public double getMonthlyHours(){ return hoursWorked; }
public void setDepartment(Department dept){ this.dept = dept; }
public Department getDepartment(){ return dept; }
@Override public double earns() { return hoursWorked * super.getPayRate(); }
@Override public void addCourse(Course aCourse) { if(super.getCourses().size() > 0){ System.out.println(super.getCourses().get(0).getDepartment().getDepartmentName() + super.getCourses().get(0).getCourseNumber() + " is removed from " + super.getName() + "'s schedule(Staff can only take one class at a time). " + aCourse.getDepartment().getDepartmentName() + aCourse.getCourseNumber() + " has been added to " + super.getName() + "'s Schedule."); super.getCourses().get(0).removeStudent(this); super.getCourses().remove(0); } super.getCourses().add(aCourse); aCourse.addStudent(this); }
} Student.java
package org.university.people;
import org.university.software.Course; import org.university.hardware.Department;
public class Student extends Person {
private Department dept; private int unitsCompleted; private int totalUnitsNeeded;
public Student(){ dept = new Department(); unitsCompleted = 0; totalUnitsNeeded = 0; }
public void setCompletedUnits(int units){ unitsCompleted = units; }
public int getUnitsCompleted(){ return unitsCompleted; }
public int requiredToGraduate(){ return totalUnitsNeeded - unitsCompleted; }
public void setRequiredCredits(int units){ totalUnitsNeeded = units; }
public int getTotalUnits(){ return totalUnitsNeeded; }
public void setDepartment(Department dept){ this.dept = dept; if(!this.dept.getStudentList().contains(this)){ this.dept.addStudent(this); } }
public Department getDepartment(){ return dept; }
public void addCourse(Course crs){ if(!detectConflict(crs)){ super.getCourses().add(crs); if(!crs.getStudentRoster().contains(this)) crs.addStudent(this); } }
public void dropCourse(Course crs){ if(super.getCourses().contains(crs)){ super.getCourses().remove(crs); if(crs.getStudentRoster().contains(this)) crs.removeStudent(this); } else{ System.out.println("The course " + crs.getDepartment().getDepartmentName() + crs.getCourseNumber() + " could not be " + "dropped because " + super.getName() + " is not enrolled in " + crs.getDepartment().getDepartmentName() + crs.getCourseNumber() + "."); } }
}
Course.java
package org.university.software;
import java.util.ArrayList; import org.university.people.Person; import org.university.hardware.Department; import org.university.hardware.Classroom; import org.university.people.Professor; import org.university.people.Staff; import org.university.people.Student;
public class Course { private String[] Week = {"Mon", "Tue", "Wed", "Thu", "Fri"}; private String[] Slot = {"8:00am to 9:15am", "9:30am to 10:45am", "11:00am to 12:15pm", "12:30pm to 1:45pm", "2:00pm to 3:15pm", "3:30pm to 4:45pm"}; private String name; private int number; private ArrayList
public Course(){ name = ""; number = -1; prof = null; schedule = new ArrayList
public String getMeetingTime(int timeSlot){ if(schedule.contains(timeSlot)) return Week[timeSlot / 100 - 1] + " " + Slot[timeSlot % 10 - 1]; return ""; }
public void printSchedule(){ for(int timeSlot : schedule){ System.out.println(getMeetingTime(timeSlot) + " " + room.getRoomNumber()); } }
public void printRoster(){ for(Person snt : roster){ System.out.println(snt.getName()); } }
public void setRoomAssigned(Classroom newRoom){ newRoom.addCourse(this); room = newRoom; }
public Classroom getRoomAssigned(){ return room; }
public boolean compareSchedules(Course other){ for(Integer timeSlot : other.schedule){ if(schedule.contains(timeSlot)) return true; } return false; }
public void setName(String name){ this.name = name; }
public String getName(){ return name; }
public void setProfessor(Professor prof){ this.prof = prof; }
public Professor getProfessor(){ return prof; }
public void setCourseNumber(int num){ number = num; }
public int getCourseNumber(){ return number; }
public void setSchedule(int addNum){ schedule.add(addNum); }
public ArrayList
public void setDepartment(Department dept){ this.dept = dept; if(!this.dept.getCourseList().contains(this)) this.dept.addCourse(this); }
public Department getDepartment(){ return dept; }
public void addStudent(Person student){ roster.add(student); if(!student.getCourses().contains(this)){ student.addCourse(this); } }
public void removeStudent(Student student){ roster.remove(student); if(student.getCourses().contains(this)){ student.dropCourse(this); } }
public void removeStudent(Staff student){ roster.remove(student); }
public ArrayList
public ArrayList
Driver1.java
package org.university.software;
import org.university.hardware.Classroom;
import org.university.hardware.Department;
import org.university.people.Professor;
import org.university.people.Student;
import org.university.people.Staff;
/* Test driver by Lahiru Ariyananda and Peter Hall */
public class Driver1
{
public static void main(String[] args)
{
/* Initialize University */
University univ = new University();
/*
* Create University of department, classrooms, professors,
* students, staff.
*/
/* Set Department */
Department dept1 = new Department();
Department dept2 = new Department();
/* Set Student */
Student s1 = new Student();
Student s2 = new Student();
Student s3 = new Student();
Student s4 = new Student();
/* Set Course */
Course c1 = new Course();
Course c2 = new Course();
Course c3 = new Course();
Course c4 = new Course();
Course c5 = new Course();
Course c6 = new Course();
Course c7 = new Course();
Course c8 = new Course();
/* Set Room */
Classroom cr1 = new Classroom();
Classroom cr2 = new Classroom();
Classroom cr3 = new Classroom();
Classroom cr4 = new Classroom();
/* Set Professor */
Professor p1 = new Professor();
Professor p2 = new Professor();
Professor p3 = new Professor();
Professor p4 = new Professor();
Professor p5 = new Professor();
/* Set Staff */
Staff sf1 = new Staff();
/* Set Attributes */
dept1.setDepartmentName("ECE");
dept2.setDepartmentName("CS");
s1.setName("Lahiru");
dept1.addStudent(s1);
s2.setName("Daz");
dept1.addStudent(s2);
s3.setName("Ben");
dept2.addStudent(s3);
s4.setName("Jerry");
dept2.addStudent(s4);
c1.setCourseNumber(387);
c1.setName("Enterprise Web Applications");
dept2.addCourse(c1);
c2.setCourseNumber(372);
c2.setName("Comparative Programming Languages");
dept2.addCourse(c2);
c3.setCourseNumber(345);
c3.setName("Discrete Structures");
dept2.addCourse(c3);
c4.setCourseNumber(426);
c4.setName("Computer Networks");
dept2.addCourse(c4);
c5.setCourseNumber(275);
c5.setName("Computer Programming II");
dept1.addCourse(c5);
c6.setCourseNumber(320);
c6.setName("Circuits Analysis");
dept1.addCourse(c6);
c7.setCourseNumber(373);
c7.setName("Object Oriented Software Design");
dept1.addCourse(c7);
c8.setCourseNumber(107);
c8.setName("Experimental Course");
dept1.addCourse(c8);
cr1.setRoomNumber("Harvill 101");
cr2.setRoomNumber("Harvill 203");
cr3.setRoomNumber("Gould Simpson 102");
cr4.setRoomNumber("Gould Simpson 105");
p1.setName("Regan");
dept1.addProfessor(p1);
p2.setName("RosenBlit");
dept1.addProfessor(p2);
p3.setName("Tharp");
dept1.addProfessor(p3);
p4.setName("Kececioglu");
dept2.addProfessor(p4);
p5.setName("Homer");
dept2.addProfessor(p5);
sf1.setName("Carol");
dept2.addStaff(sf1);
univ.departmentList.add(dept2);
univ.departmentList.add(dept1);
univ.classroomList.add(cr1);
univ.classroomList.add(cr2);
univ.classroomList.add(cr3);
univ.classroomList.add(cr4);
/* Initialization ending */
/* Test1 beginning */
c1.setSchedule(201);
c1.setSchedule(401);
c2.setSchedule(202);
c2.setSchedule(402);
c3.setSchedule(303);
c3.setSchedule(503);
c4.setSchedule(102);
c4.setSchedule(302);
c5.setSchedule(303);
c5.setSchedule(503);
c6.setSchedule(102);
c6.setSchedule(302);
c7.setSchedule(201);
c7.setSchedule(401);
c8.setSchedule(102);
/* Sign up professors */
/* Sign up Prof. Tharp for class ECE320 */
p3.addCourse(c6);
/* Sign up Prof. Kececioglu for class CS387 */
p4.addCourse(c1);
/* Sign up Prof. Kececioglu for class CS372 */
p4.addCourse(c2);
/* Sign up Prof. Homer for class CS345 */
p5.addCourse(c3);
/* Sign up Prof. Homer for class CS426 */
p5.addCourse(c4);
/* Set classrooms for courses */
c1.setRoomAssigned(cr2);
c2.setRoomAssigned(cr4);
c3.setRoomAssigned(cr3);
c4.setRoomAssigned(cr3);
c6.setRoomAssigned(cr1);
/* Add course to students */
s1.addCourse(c4);
s1.addCourse(c1);
s2.addCourse(c2);
s2.addCourse(c3);
s2.addCourse(c8);
s3.addCourse(c1);
s3.addCourse(c2);
s3.addCourse(c3);
s3.addCourse(c4);
s4.addCourse(c1);
s4.addCourse(c2);
s4.addCourse(c6);
System.out.println("Testing Professor Conflicts ...");
p3.addCourse(c1);
p4.addCourse(c3);
System.out.println(" Testing dropCourse method in Student class ...");
s1.dropCourse(c7);
s1.addCourse(c5);
s1.dropCourse(c5);
s2.dropCourse(c8);
/* Test conflicts of student schedule */
System.out.println(" Conflicts:");
/* Test conflicts of classroom schedule */
c8.setRoomAssigned(cr1);
/* Test conflicts of professor schedule */
p3.addCourse(c8);
p5.addCourse(c5);
/* Try to add ECE320 to student Lahiru */
s1.addCourse(c6);
/* Try to add ECE373 to student Lahiru */
s1.addCourse(c7);
s2.addCourse(c5);
/* Add course to staff */
sf1.addCourse(c1);
/* Test adding more than 1 course */
sf1.addCourse(c2);
/* Schedules */
System.out.println(" The schedule for Prof. " + univ.departmentList.get(0).getProfessorList().get(2).getName() + ":");
univ.getdepartmentList().get(0).getProfessorList().get(2).printSchedule();
System.out.println(" The schedule for Prof. " + univ.departmentList.get(1).getProfessorList().get(0).getName() + ":");
univ.getdepartmentList().get(1).getProfessorList().get(0).printSchedule();
System.out.println(" The schedule for Prof. " + univ.departmentList.get(1).getProfessorList().get(1).getName() + ":");
univ.getdepartmentList().get(1).getProfessorList().get(1).printSchedule();
/* Print schedule of classroom 1 */
System.out.println(" The schedule for " + univ.classroomList.get(0).getRoomNumber() + ":");
univ.classroomList.get(0).printSchedule();
/* Print schedule of classroom 2 */
System.out.println(" The schedule for " + univ.classroomList.get(1).getRoomNumber() + ":");
univ.classroomList.get(1).printSchedule();
/* Print schedule of classroom 3 */
System.out.println(" The schedule for " + univ.classroomList.get(2).getRoomNumber() + ":");
univ.classroomList.get(2).printSchedule();
/* Print schedule of classroom 4 */
System.out.println(" The schedule for " + univ.classroomList.get(3).getRoomNumber() + ":");
univ.classroomList.get(3).printSchedule();
/* Student schedules */
System.out.println(" The schedule for Student " + univ.departmentList.get(0).getStudentList().get(0).getName() + ":");
univ.departmentList.get(0).getStudentList().get(0).printSchedule();
System.out.println(" The schedule for Student " + univ.departmentList.get(1).getStudentList().get(0).getName() + ":");
univ.departmentList.get(1).getStudentList().get(0).printSchedule();
System.out.println(" The schedule for Student " + univ.departmentList.get(1).getStudentList().get(1).getName() + ":");
univ.departmentList.get(1).getStudentList().get(1).printSchedule();
/* Staff schedules */
System.out.println(" The schedule for Employee " + univ.departmentList.get(1).getStaffList().get(0).getName() + ":");
univ.departmentList.get(1).getStaffList().get(0).printSchedule();
/* Print schedule of classroom 4 */
System.out.println(" The schedule for course " + univ.departmentList.get(1).getCourseList().get(0).getName() + ":");
univ.departmentList.get(1).getCourseList().get(0).printSchedule();
}
}
University.java
package org.university.software;
import java.util.ArrayList;
import org.university.hardware.Department;
import org.university.hardware.Classroom;
public class University {
public ArrayList
public ArrayList
public University(){
departmentList = new ArrayList
classroomList = new ArrayList
}
public ArrayList
{
return departmentList;
}
public void printStudentList(){
for(Department dept : departmentList){
dept.printStudentList();
}
}
public void printStaffList(){
for(Department dept : departmentList){
dept.printStaffList();
}
}
public void printProfessorList(){
for(Department dept : departmentList){
dept.printProfessorList();
}
}
public void printCourseList(){
for(Department dept : departmentList){
dept.printCourseList();
}
}
}
Here is Task1Results.txt :
Before Serialization: List of departments: ECE CS Classroom list: Harvill 101 Harvill 203 Gould Simpson 102 Gould Simpson 105 The professor list for department ECE Regan RosenBlit Tharp The professor list for department CS Kececioglu Homer The course list for department ECE ECE275 ECE320 ECE373 ECE107 The course list for department CS CS387 CS372 CS345 CS426 The schedule for classroom Harvill 101 Mon 9:30am to 10:45am ECE320 Circuits Analysis Wed 9:30am to 10:45am ECE320 Circuits Analysis The schedule for classroom Harvill 203 Tue 8:00am to 9:15am CS387 Enterprise Web Applications Thu 8:00am to 9:15am CS387 Enterprise Web Applications The schedule for classroom Gould Simpson 102 Mon 9:30am to 10:45am CS426 Computer Networks Wed 9:30am to 10:45am CS426 Computer Networks Wed 11:00am to 12:15pm CS345 Discrete Structures Fri 11:00am to 12:15pm CS345 Discrete Structures The schedule for classroom Gould Simpson 105 Tue 9:30am to 10:45am CS372 Comparative Programming Languages Thu 9:30am to 10:45am CS372 Comparative Programming Languages Department ECE Printing Professor schedules: The schedule for Prof. Regan: The schedule for Prof. RosenBlit: The schedule for Prof. Tharp: Mon 9:30am to 10:45am ECE320 Circuits Analysis Wed 9:30am to 10:45am ECE320 Circuits Analysis Printing Student Schedules: The schedule for Student Lahiru: Mon 9:30am to 10:45am CS426 Computer Networks Tue 8:00am to 9:15am CS387 Enterprise Web Applications Wed 9:30am to 10:45am CS426 Computer Networks Thu 8:00am to 9:15am CS387 Enterprise Web Applications The schedule for Student Daz: Tue 9:30am to 10:45am CS372 Comparative Programming Languages Wed 11:00am to 12:15pm CS345 Discrete Structures Thu 9:30am to 10:45am CS372 Comparative Programming Languages Fri 11:00am to 12:15pm CS345 Discrete Structures Printing Staff Schedules: The rosters for courses offered by ECE The roster for course ECE275 The roster for course ECE320 Jerry The roster for course ECE373 The roster for course ECE107 Department CS Printing Professor schedules: The schedule for Prof. Kececioglu: Tue 8:00am to 9:15am CS387 Enterprise Web Applications Tue 9:30am to 10:45am CS372 Comparative Programming Languages Thu 8:00am to 9:15am CS387 Enterprise Web Applications Thu 9:30am to 10:45am CS372 Comparative Programming Languages The schedule for Prof. Homer: Mon 9:30am to 10:45am CS426 Computer Networks Wed 9:30am to 10:45am CS426 Computer Networks Wed 11:00am to 12:15pm CS345 Discrete Structures Fri 11:00am to 12:15pm CS345 Discrete Structures Printing Student Schedules: The schedule for Student Ben: Mon 9:30am to 10:45am CS426 Computer Networks Tue 8:00am to 9:15am CS387 Enterprise Web Applications Tue 9:30am to 10:45am CS372 Comparative Programming Languages Wed 9:30am to 10:45am CS426 Computer Networks Wed 11:00am to 12:15pm CS345 Discrete Structures Thu 8:00am to 9:15am CS387 Enterprise Web Applications Thu 9:30am to 10:45am CS372 Comparative Programming Languages Fri 11:00am to 12:15pm CS345 Discrete Structures The schedule for Student Jerry: Mon 9:30am to 10:45am ECE320 Circuits Analysis Tue 8:00am to 9:15am CS387 Enterprise Web Applications Tue 9:30am to 10:45am CS372 Comparative Programming Languages Wed 9:30am to 10:45am ECE320 Circuits Analysis Thu 8:00am to 9:15am CS387 Enterprise Web Applications Thu 9:30am to 10:45am CS372 Comparative Programming Languages Printing Staff Schedules: The schedule for Employee Carol: Tue 8:00am to 9:15am CS387 Enterprise Web Applications Thu 8:00am to 9:15am CS387 Enterprise Web Applications Staff : Carol earns $3200.00 this month The rosters for courses offered by CS The roster for course CS387 Lahiru Ben Jerry Carol The roster for course CS372 Daz Ben Jerry The roster for course CS345 Daz Ben The roster for course CS426 Lahiru Ben Post Serialization: List of departments: ECE CS Classroom list: Harvill 101 Harvill 203 Gould Simpson 102 Gould Simpson 105 The professor list for department ECE Regan RosenBlit Tharp The professor list for department CS Kececioglu Homer The course list for department ECE ECE275 ECE320 ECE373 ECE107 The course list for department CS CS387 CS372 CS345 CS426 The schedule for classroom Harvill 101 Mon 9:30am to 10:45am ECE320 Circuits Analysis Wed 9:30am to 10:45am ECE320 Circuits Analysis The schedule for classroom Harvill 203 Tue 8:00am to 9:15am CS387 Enterprise Web Applications Thu 8:00am to 9:15am CS387 Enterprise Web Applications The schedule for classroom Gould Simpson 102 Mon 9:30am to 10:45am CS426 Computer Networks Wed 9:30am to 10:45am CS426 Computer Networks Wed 11:00am to 12:15pm CS345 Discrete Structures Fri 11:00am to 12:15pm CS345 Discrete Structures The schedule for classroom Gould Simpson 105 Tue 9:30am to 10:45am CS372 Comparative Programming Languages Thu 9:30am to 10:45am CS372 Comparative Programming Languages Department ECE Printing Professor schedules: The schedule for Prof. Regan: The schedule for Prof. RosenBlit: The schedule for Prof. Tharp: Mon 9:30am to 10:45am ECE320 Circuits Analysis Wed 9:30am to 10:45am ECE320 Circuits Analysis Printing Student Schedules: The schedule for Student Lahiru: Mon 9:30am to 10:45am CS426 Computer Networks Tue 8:00am to 9:15am CS387 Enterprise Web Applications Wed 9:30am to 10:45am CS426 Computer Networks Thu 8:00am to 9:15am CS387 Enterprise Web Applications The schedule for Student Daz: Tue 9:30am to 10:45am CS372 Comparative Programming Languages Wed 11:00am to 12:15pm CS345 Discrete Structures Thu 9:30am to 10:45am CS372 Comparative Programming Languages Fri 11:00am to 12:15pm CS345 Discrete Structures Printing Staff Schedules: The rosters for courses offered by ECE The roster for course ECE275 The roster for course ECE320 Jerry The roster for course ECE373 The roster for course ECE107 Department CS Printing Professor schedules: The schedule for Prof. Kececioglu: Tue 8:00am to 9:15am CS387 Enterprise Web Applications Tue 9:30am to 10:45am CS372 Comparative Programming Languages Thu 8:00am to 9:15am CS387 Enterprise Web Applications Thu 9:30am to 10:45am CS372 Comparative Programming Languages The schedule for Prof. Homer: Mon 9:30am to 10:45am CS426 Computer Networks Wed 9:30am to 10:45am CS426 Computer Networks Wed 11:00am to 12:15pm CS345 Discrete Structures Fri 11:00am to 12:15pm CS345 Discrete Structures Printing Student Schedules: The schedule for Student Ben: Mon 9:30am to 10:45am CS426 Computer Networks Tue 8:00am to 9:15am CS387 Enterprise Web Applications Tue 9:30am to 10:45am CS372 Comparative Programming Languages Wed 9:30am to 10:45am CS426 Computer Networks Wed 11:00am to 12:15pm CS345 Discrete Structures Thu 8:00am to 9:15am CS387 Enterprise Web Applications Thu 9:30am to 10:45am CS372 Comparative Programming Languages Fri 11:00am to 12:15pm CS345 Discrete Structures The schedule for Student Jerry: Mon 9:30am to 10:45am ECE320 Circuits Analysis Tue 8:00am to 9:15am CS387 Enterprise Web Applications Tue 9:30am to 10:45am CS372 Comparative Programming Languages Wed 9:30am to 10:45am ECE320 Circuits Analysis Thu 8:00am to 9:15am CS387 Enterprise Web Applications Thu 9:30am to 10:45am CS372 Comparative Programming Languages Printing Staff Schedules: The schedule for Employee Carol: Tue 8:00am to 9:15am CS387 Enterprise Web Applications Thu 8:00am to 9:15am CS387 Enterprise Web Applications Staff : Carol earns $3456.00 this month The rosters for courses offered by CS The roster for course CS387 Lahiru Ben Jerry Carol The roster for course CS372 Daz Ben Jerry The roster for course CS345 Daz Ben The roster for course CS426 Lahiru BenTaskl This task will involve serializing and de-serializing objects. Note this task will not involve any GUIs. Run Driver1.java. Your output should match TasklResults.txt. Results can be displayed in the eclipse console. .The University class should contain 2 new methods saveData(univ:University) and loadData). The saveData(univ:University) is a static method that should save all the serialized object data of the University into a file called "university.ser" that should be saved under project root folder o o The loadData0 method should be able to read from the university.ser file and restore the object data. o Hint you will need to make all relevant classes under org.university.hardware, org.university.people and org.university.software serializable. The University class will also need an additional method printAll0. It should report the information about all courses, departments, classrooms, etc. as depicted in the TasklResults.txt file
Step by Step Solution
There are 3 Steps involved in it
Step: 1
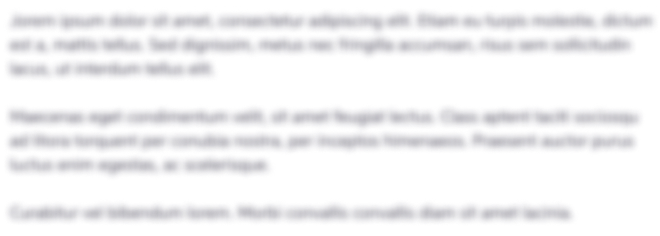
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started