Question
JAVA PROGRAMMING HELP WITH KeyListener GUI I need to create a program that allows the user to paint a pixel picture by using a grid
JAVA PROGRAMMING HELP WITH KeyListener GUI
I need to create a program that allows the user to paint a pixel picture by using a grid of panels. The user can use the arrow keys on the keyboard to move a highlighted space around the grid(yellow). When the user presses the spacebar the selected panel will be set to black, if space is pressed again on a black panel then it will go back to white.
HERE IS MY CODE SO FAR PLEASE COPY AND PASTE IT INTO A COMPILER AND HELP ME FIX THE FUNCTIONS
package demo;
import java.awt.*;
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
public class JKeyDemo extends JFrame implements KeyListener {
private JTextField textField = new JTextField(10);
private final int ROWS = 16;
private final int COLS = 16;
private final int GAP = 2;
private final int NUM = ROWS * COLS;
private int currentX = 8;
private int currentY = 8;
private JFrame fram = new JFrame();
private JPanel pane = new JPanel(new GridLayout(ROWS,COLS,GAP,GAP));
private JPanel[][] panel = new JPanel[ROWS][COLS];
private Color color1 = Color.WHITE;
private Color color2 = Color.YELLOW;
private Color color3 = Color.BLACK;
private Color tempColor;
public JKeyDemo()
{
super ("Pixel Painter");
setLayout(new BorderLayout());
add(pane);
for (int x = 0; x < ROWS; ++x)
{
for(int y = 1; y < ROWS; ++y)
{
panel[x][y] = new JPanel();
pane.add(panel[x][y]);
panel[x][y].setBackground(color1);
}
}
panel[currentX][currentY].setBackground(color2);
}
public void keyPressed(KeyEvent e) {
int keyCode = e.getKeyCode();
if( keyCode == KeyEvent.VK_UP)
{
currentY++;
panel[currentX][currentY].setBackground(color2);
}
else if ( keyCode == KeyEvent.VK_DOWN) {
currentY--;
panel[currentX][currentY].setBackground(color2);
}
else if (keyCode == KeyEvent.VK_SPACE) {
panel[currentX][currentY].setBackground(color3);
}
else if ( keyCode == KeyEvent.VK_LEFT) {
currentX++;
panel[currentX][currentY].setBackground(color2);
}
else if ( keyCode == KeyEvent.VK_RIGHT) {
currentX--;
panel[currentX][currentY].setBackground(color2);
}
}
public static void main(String[] args) {
JKeyDemo frame = new JKeyDemo();
frame.setSize(250,100);
frame.setVisible(true);
}
@Override
public void keyTyped(KeyEvent e) {
// TODO Auto-generated method stub
}
@Override
public void keyReleased(KeyEvent e) {
// TODO Auto-generated method stub
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
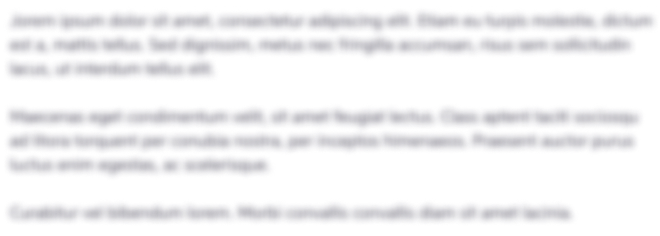
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started