Question
Java programming question. A very simple company system. Note this is a 2 part Question. Part 1 has been posted. Here is the link: https://www.chegg.com/homework-help/questions-and-answers/codes-provided-public-class-policy-private-static-int-nextpolicynumber-1-private-int-polic-q27203199
Java programming question. A very simple company system. Note this is a 2 part Question. Part 1 has been posted. Here is the link: https://www.chegg.com/homework-help/questions-and-answers/codes-provided-public-class-policy-private-static-int-nextpolicynumber-1-private-int-polic-q27203199
codes required:
import java.util.*;
public abstract class Client { private static final int MAX_POLICIES_PER_CLIENT = 10;
private static int NEXT_CLIENT_ID = 1;
private String name; private int id; protected Policy[] policies; protected int numPolicies;
public Client(String n) { name = n; id = NEXT_CLIENT_ID++; policies = new Policy[MAX_POLICIES_PER_CLIENT]; numPolicies = 0; }
public String getName() { return name; } public int getId() { return id; } public Policy[] getPolicies() { return policies; } public int getNumPolicies() { return numPolicies; }
public String toString() { return String.format("Client: %06d amount: %s", id, name); } }
***************************************
import java.util.GregorianCalendar;
public class ClientTester { public static void main(String args[]) { // Create an individual client, open some policies and then make some claims IndividualClient ic = new IndividualClient("Bob B. Pins"); ic.openPolicyFor(100); ic.openPolicyFor(200, 0.10f); ic.openPolicyFor(300, new GregorianCalendar(2020, 0, 2, 23, 59).getTime()); ic.openPolicyFor(400, new GregorianCalendar(2009, 5, 4, 12, 00).getTime()); Policy p = new Policy(500); System.out.println("Here are the Individual Client's policies:"); for (int i=0; i System.out.println(" " + ic.getPolicies()[i]);
System.out.println("Making claims:"); System.out.println(String.format(" Claim for policy 0001: $%6.2f",ic.makeClaim(1))); System.out.println(String.format(" Claim for policy 0002: $%6.2f",ic.makeClaim(2))); System.out.println(String.format(" Claim for policy 0003: $%6.2f",ic.makeClaim(3))); System.out.println(String.format(" Claim for policy 0004: $%6.2f",ic.makeClaim(4))); System.out.println(String.format(" Claim for policy 0005: $%6.2f",ic.makeClaim(5))); System.out.println("Here are the Individual Client's policies after claims:"); for (int i=0; i System.out.println(" " + ic.getPolicies()[i]); System.out.println(String.format("The total policy coverage for this client: $%1.2f",ic.totalCoverage()));
// Create a company client, open some policies and then make some claims CompanyClient cc = new CompanyClient("The Pillow Factory"); cc.openPolicyFor(1000); cc.openPolicyFor(2000, 0.10f); cc.openPolicyFor(3000, new GregorianCalendar(2020, 0, 2, 23, 59).getTime()); cc.openPolicyFor(4000, new GregorianCalendar(2009, 5, 4, 12, 00).getTime()); System.out.println(" Here are the Company Client's policies:"); for (int i=0; i System.out.println(" " + cc.getPolicies()[i]);
System.out.println("Making claims:"); System.out.println(String.format(" Claim for policy 0006: $%7.2f",cc.makeClaim(6))); System.out.println(String.format(" Claim for policy 0007: $%7.2f",cc.makeClaim(7))); System.out.println(String.format(" Claim for policy 0008: $%7.2f",cc.makeClaim(8))); System.out.println(String.format(" Claim for policy 0009: $%7.2f",cc.makeClaim(9))); System.out.println(String.format(" Claim for policy 0005: $%7.2f",cc.makeClaim(5)));
System.out.println("Here are the Company Client's policies after claims:"); for (int i=0; i System.out.println(" " + cc.getPolicies()[i]); System.out.println(String.format("The total policy coverage for this client: $%1.2f",cc.totalCoverage())); System.out.println("Cancelling policy #12 ... did it work: " + cc.cancelPolicy(12)); System.out.println("Cancelling policy #8 ... did it work: " + cc.cancelPolicy(8)); System.out.println(String.format("The total policy coverage for this client: $%1.2f",cc.totalCoverage())); } }
(2) The Clients We will now define the following hierarchy: To begin, copy the code from the following Client class import java.util.*; public abstract class Client f Object Client IndividualClient CompanyClient private static final int MAX POLICIES PER CLIENT = 10; private static int NEXTCLIENTID 1; rivate String private int protected Policy [] protected int name id; policies numPolicies; public Client (String n) i name = n ; id = NEXT CLIENT ID++; policies = new Policy [MAX POLICIES PER CLIENT] ; numPolicies = 0; public String getName) return name; ) public int getId) return id; 1 public Policy getPolicies ) return policies; ) public int getNumPolicies ) return numPolicies; public String toString) return String . forma t ("Client : %06d amount: %s", id, name); Create the following in the Client class a public method called totalCoverage) which returns a float containing the total amount of coverage of all the client's policies provided that the limit has not been reached and returns the Policy object, or null if not added specified in the parameter and adds it to the client using (and returning the result from) the . a public method called addPolicy (Policy p) which adds the given Policy to the array of policies . a public method called openPolicyFor(float amt) which creates a new Policy for the amount addPolicy() methodStep by Step Solution
There are 3 Steps involved in it
Step: 1
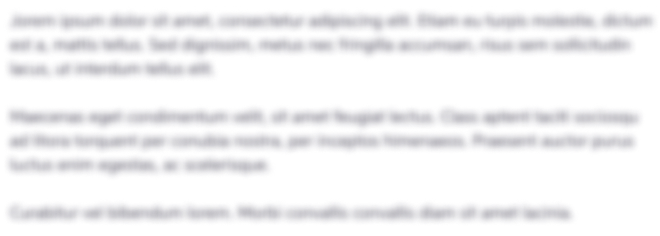
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started