Question
Java Programming question: Develop an online store which sells dvds and books execpt this time, we will also be selling AudioBooks which are specialized versions
Java Programming question:
Develop an online store which sells dvds and books execpt this time,
we will also be selling AudioBooks which are specialized versions of Books (i.e., extension will come into play here). Use of multiple classes is a must for this Assignment and the perspective will be that of a store owner maintaining a Catalog.
The updated requirements for entities are as follows:
Each CatalogItem has a title of type String.
Each CatalogItem has a price of type double.
Each Book (is a CatalogItem) has an author which is of type String.
Each Book has an ISBN number which is of type int. Every book has a unique ISBN number.
Each AudioBook has all of the properties of a Book, and in addition has a property named
runningTime of type double (conceptually you can think of this as minutes, but it doesn't matter
for the purposes of the assignment).
Each DVD (is a CatalogItem) has director which is of type String.
Each DVD has a year which is of type int.
Each DVD has an dvdcode which is of type int and is unique for all DVDs.
Each of the properties is mandatory (cannot be a default) and private, and only retrieved via public getter methods (for example: getPrice which would return the price of a Book or DVD), and the properties can only be set via constructor functions.
Additionally, AudioBooks are special - when the price is retrieved via the method getPrice, the method in
the parent (Book) class is overridden, and instead the price is retrieved as the regular price of the book with a 10% discount (i.e., 90% of the price).
Each of the classes must define a toString method which *returns* information about the entity as follows:
For Books it would be:
Title:
For AudioBooks it would be the same for books, except the price would be the discounted price and we
would append: | RunningTime:
For DVDs it would be:
Title:
We do not have a limit on the number of Books/AudioBooks/DVDs that our store can hold, and thus, ArrayLists would be useful here. You should have two ArrayLists: one for Books and one for DVDs and the ArrayLists should be typed accordingly (i.e., the code should not be declared unsafe upon compilation). You are *not allowed* to have a separate ArrayList for AudioBooks.
The distinction between Books and AudioBooks should be easy enough using the instanceof operator. Also there is no such thing as a standalone CatalogItem [Hint: this isn't a concrete class]. Meaning it's Abstract!
The menu option displayed would now look like:
**Welcome to the Comets Books and DVDs Store (Catalog Section)**
Choose from the following options:
1 - Add Book
2 - Add AudioBook
3 - Add DVD
4 - Remove Book
5 - Remove DVD
6 - Display Catalog
9 - Exit store
Then, within a loop, if the user enters an option that is not 1, 2, 3, 4, 5, 6, or 9, display the message "This option is not acceptable" and loop back to redisplay the menu. Continue in this manner until a correct option is input.
You may implement the methods however you want as long as you satisfy the class relationships, and satisfy the following behavior:
If options 1, 2 or 3 are entered, follow-up by asking the User to enter the required details. Invalid values are not allowed: for example, a book must have a non-empty title and author, and a positive running time and price. An AudioBook must also have a positive running time. Similar restrictions apply on DVDs. If the User enters something invalid, prompt them to enter it again. If the User tries to add a Book or DVD that already exists (i.e., we already have a Book with that ISBN number or a DVD with that dvdcode) then let the User know that this is the case and return to the main menu. [Hint: Therefore, when options 1, 2 or 3 are entered, the first thing to ask the User for would be the ISBN number or the DVD code respectively].
If option 4 is selected, ask the User to enter the ISBN number to remove: if it exists in the Catalog,t hen remove it; and if it doesn't then let the User know by saying "The Book doesn't exist in the Catalog" (and then return to the main menu). Similar behavior applies to option 5, except this time with DVDs. After successful removal, display the Catalog (i.e., the behavior of option 6, and redisplay the main menu).
If option 6 is selected then display the entire Catalog to the User by displaying all the Book
information followed by all the DVD information, with the following line to separate the two.
The information for each Book or DVD must be displayed using the respective toString methods.
Continue the looping until option 9 is selected, and when option 9 is entered, end the program.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
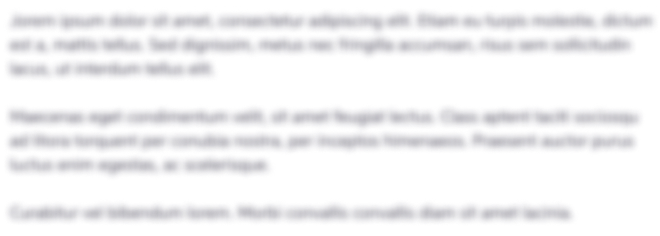
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started