Question
Java programming question: Two files are required, a data class named Player and an executable class named PlayerTest. Class Player (a data class for a
Java programming question:
Two files are required, a data class named Player and an executable class named PlayerTest.
Class Player (a data class for a hockey player, no main method)
- has five instance data members, all private:
- String name, String position, double salary, int goals, int assists.
- one public static, or class, member of type int named roster assigned an initial value of zero.
- a no-arg constructor, and another parameterized constructor that sets values in all data members when called.
- Both constructors should increment the roster static data member.
- has setters and getters for all data members.
- a void method named applyBonus() defined with one int parameter for a scoring bonus. This method will apply the specified bonus to a player's salary based on total scoring points (goals plus assists).
- a toString() method that returns the state of all data members of a Player instance
Class PlayerTest (executable class with a main method)
In main:
- using the array initializer syntax, create an array of at least six Player objects using the parameterized constructor. Feel free to make up values for the Player attributes.
- Using a method of class Arrays, code an ArrayList of Player objects from the array.
- Use class Player's no-arg constructor to make another Player instance. Add this new Player to the ArrayList. Use the setter methods of Player to assign values to its attributes.
- Report the roster size, then use a foreach loop to display all Players in the ArrayList.
- Pass the ArrayList to a custom, void method named salaryBonus().
In the salaryBonus(int bonus) method:
- process the list and execute class Player's applyBonus() method for high-scoring players.
- Defense players scoring 50 or more points (goals + assists) get a $1,500,000 bonus.
- all other positions get a $2,500,000 bonus if they score 90 or more points.
- output the name of each Player getting a bonus.
- Finish PlayerTest with a for loop to process the ArrayList again to verify the salary bonuses.
Example Output
Team roster size is 7 players
Players before applying salary bonuses Stamkos, Center, salary $9,500,000, 40 goals, 42 assists
Kucherov, R Wing, salary $5,550,000, 37 goals, 62 assists
Killorn, L Wing, salary $4,450,000, 21 goals, 25 assists
Point, Center, salary $650,000, 45 goals, 52 assists
Hedman, Defense, salary $8,000,000, 10 goals, 43 assists
Johnson, Center, salary $6,750,000, 37 goals, 30 assists
McDonagh, Defense, salary $5,300,000, 7 goals, 41 assists
Players getting a salary bonus: Kucherov Point Hedman
Players after applying salary bonuses
Stamkos, Center, salary $9,500,000, 40 goals, 42 assists
Kucherov, R Wing, salary $7,550,000, 37 goals, 62 assists
Killorn, L Wing, salary $4,450,000, 21 goals, 25 assists
Point, Center, salary $2,650,000, 45 goals, 52 assists
Hedman, Defense, salary $9,500,000, 10 goals, 43 assists
Johnson, Center, salary $6,750,000, 37 goals, 30 assists
McDonagh, Defense, salary $5,300,000, 7 goals, 41 assists
Step by Step Solution
There are 3 Steps involved in it
Step: 1
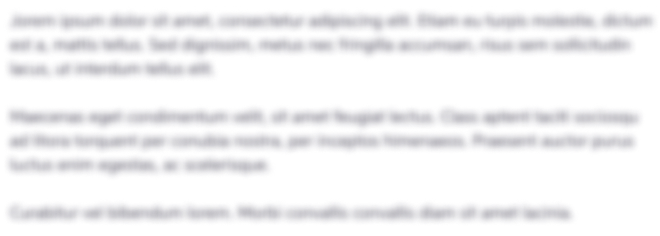
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started