Question
Java Programming Using the code from BallPane.java and BounceBallControl.java (chapter 15), create a Pong for One game - a rectangular paddle moves back and forth
Java Programming
Using the code from BallPane.java and BounceBallControl.java (chapter 15), create a "Pong for One" game - a rectangular paddle moves back and forth via mouse drag along the bottom of the pane; the bottom of the paddle should be about 1/2 the diameter of the ball off the bottom. If the ball connects with the paddle, then it bounces at a 90 degree angle back into the pane space. If the ball misses the paddle, then the score is decremented by 1. The game ends when 20 points are lost.
Nice things:
A label that displays the score (you can start at 20 and go to zero if you want...)
For every 10 paddle connections in a row, the ball moves faster
For every 10 paddle connections in a row, the ball changes color
For every (2?) paddle misses in a row, the paddle grows in length
There is MATH involved in figuring out the connection between the paddle and the ball. You probably want to pay a lot of attention to how moveBall() works.
------------------------
BallPane.java
import javafx.animation.KeyFrame; import javafx.animation.Timeline; import javafx.beans.property.DoubleProperty; 4 import javafx.scene.layout.Pane; import javafx.scene.paint.Color; import javafx.scene.shape.Circle; import javafx.util.Duration; public class BallPane extends Pane {
public final double radius = 20;
private double x = radius, y = radius;
private double dx = 1, dy = 1;
private Circle circle = new Circle(x, y, radius);
private Timeline animation;
public BallPane() {
circle.setFill(Color.GREEN); // Set ball color getChildren().add(circle); // Place a ball into this pane animation = new Timeline(
new KeyFrame(Duration.millis(50), e -> moveBall()));
animation.setCycleCount(Timeline.INDEFINITE);
animation.play(); // Start animation
} public void play(){
animation.play();
}
public void pause() {
animation.pause();
}
public void increaseSpeed() {
animation.setRate(animation.getRate() + 0.1);
}
public void decreaseSpeed() {
animation.setRate(
animation.getRate() > 0 ? animation.getRate() - 0.1 : 0);
}
public DoubleProperty rateProperty() {
return animation.rateProperty();
}
protected void moveBall() {
if (x < radius || x > getWidth() - radius) {
dx *= -1;}
if (y < radius || y > getHeight() - radius) {
dy *= -1; }
x += dx;
y += dy;
circle.setCenterX(x);
circle.setCenterY(y);
} }
-----------------------------------------
BounceBallControl.java
import javafx.application.Application;
import javafx.stage.Stage; import javafx.scene.Scene; import javafx.scene.input.KeyCode;
public class BounceBallControl extends Application {
@Override // Override the start method in the Application class
public void start(Stage primaryStage) {
BallPane ballPane = new BallPane(); // Create a ball pane
ballPane.setOnMousePressed(e -> ballPane.pause()); ballPane.setOnMouseReleased(e -> ballPane.play());
ballPane.setOnKeyPressed(e -> {
if (e.getCode() == KeyCode.UP) {
ballPane.increaseSpeed();
}
else if (e.getCode() == KeyCode.DOWN) {
ballPane.decreaseSpeed();
}
});
Scene scene = new Scene(ballPane, 250, 150);
primaryStage.setTitle("BounceBallControl"); // Set the stage title
primaryStage.setScene(scene); // Place the scene in the stage
primaryStage.show(); // Display the stage
ballPane.requestFocus();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
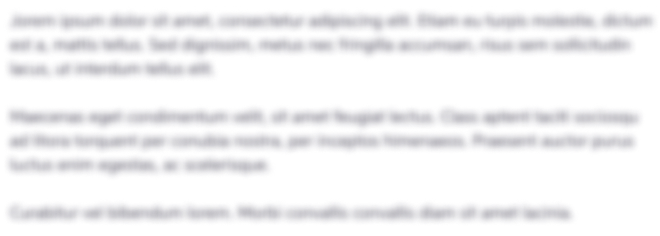
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started