Question
Java Programming: Write a class (RPSGame) that represents the game. This class only represents the game itself. This class does not interact with the userr.
Java Programming:
Write a class (RPSGame) that represents the game.
This class only represents the game itself. This class does not interact with the userr.
A game is made up of many matches. (A match is a single selection of rock, paper, or scissors). Thus, the RPSGame object is described by three characteristics: number of computer wins, number of user wins, and number of ties.
Write the instance data variables for these three characteristics and appropriate getters/setters.
Write a generateComputerPlay method that generates a random move by the computer.
Write a findWinner method that takes in two moves (the user and the computer) and determines the outcome (user wins, computer wins, or tie).
Determining the winner will require you to compare a lot of possible match-ups through a series of nested conditionals.
The method should update the appropriate win/loss/tie count (as instance data) depending on the outcome.
Include constants to represent the game states (user wins, computer wins, tie) and the moves (rock, paper, scissors).
You can represent these as Strings, ints, or something else- it's up to you.
Think through your decision and make sure it is a good design choice.
Declare and use your constants. (For example, create a constant called ROCK- this allows you to compare whether userMove == ROCK rather than userMove == 1, which is much more meaningful!)
In RPSGame:
Add instance data to represent the amount the user is betting (the same amount on each match) and the users balance of money. Write appropriate getters and setters for these instance data.
In the findWinner method, when the number of wins/losses is updated, also update the users balance by adding or subtracting their bet amount.
Write the GUI program.
This class will create the interface to interact with the user. It will also create an object of type RPSGame and invoke the methods in that class.
The constructor sets up the display and create the instance of RPSGame.
Because I dont want you to spend the majority of your time thinking through the layout, I have provided a shell file you can use as a starting point. You are not required to use this- if you want to start from scratch, you are welcome to do so. You are also welcome to make changes to the shell.
You can use my images or use your own.
The listener classes will respond to the user selecting a move and then invoke the appropriate method in the RPS game class. Write helper methods that are invoked from the listener class rather than putting all the code inside a single method.
Here is some pseudocode for the listener class. You are not required to follow this, but it's here if it's helpful.
get the move from the user (determine which button was clicked)
update the display of the users move
generate a move by the computer (by invoking a method on the RPSGame object)
update the display of the computers move
determine the winner (by invoking a method on the RPS object)
update the display of the outcome and the game stats (obtained from RPS object)
Part of this assignment is making sure that each class handles the appropriate tasks.
The RPSGame class should only handle the game- it shouldn't have any interaction with the user.
The RPSGUI class should only interact with the user- it doesn't know how to play the game at all. It only knows how to get info from the user, send that info to the game, get info back from the game, and display that info to the user.
Make sure you preserve this object-oriented idea throughout your program!
Add support for betting.
In the GUI program:
Use the JOptionPane class to ask the user about betting prior to the game starting. This code should go inside the main method.
Use the JOptionPane.showConfirmDialog method to determine whether the user wants to bet.
If the user wants to bet, use the JOptionPane.showInputDialog method to find out how much the user wants to bet.
Change the RPSGUIGame constructor so that it takes one parameter: the bet amount.
Create a new JLabel to display the users balance. I recommend adding the label to the statusPanel. You can choose another place if you want.
If the user is betting, when the display of the stats is updated, update the display of the balance. If the user is not betting, no betting or balance information should be displayed.
This is how the program works:
The user clicks a button to make their move (rock, paper, or scissors).
The program randomly generates a move for the computer.
*The program uses images to display the user's move and the computer's move.
The program displays the outcome of the match.
The program keeps track of and displays the number of computer wins, user wins, and ties.
Hope some genius can help me!
I really appreciate it.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
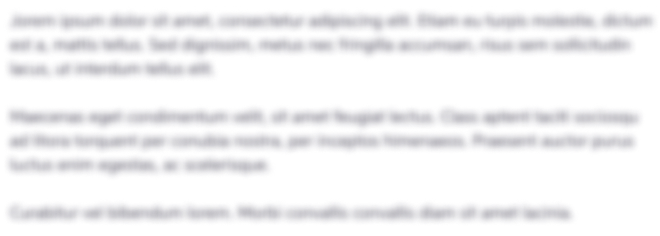
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started