Question
Java Programming You are a programmer in the IT department of an important law firm. Your job is to create a program that will report
Java Programming
You are a programmer in the IT department of an important law firm. Your job is to create a program that will report gross salary amounts and other compensation.
There are three types of employees in your firm:
Programmers Lawyers Accountants
Your computer-based solution will use inheritance to reflect the general-to-specific nature of your employee hierarchy.
Common attributes for all employees are: Name
Salary Attributes for specific employee types are:
Lawyers: Stock options earned (type int)
Accountants Parking allowance amount (type double)
Programmers: Bus pass (type boolean)
The three specific classes of employees should extend the abstract Employee class (nobody is a generic employee) and implement their own reportSalary() method.
The pay schedule is: o Employees earn the base salary of $40K per year o Accountants earn the base employee salary per year o Programmers earn the base employee salary plus $20K per year o Lawyers earn the base employee salary plus $30K per year
Requirements:
o Each subclass of Employee must implement its own reportSalary() method. The specific implementations of reportSalary() are limited to a printed line:
System.out.println(I am a lawyer. I get + getSalary() + , and I have + getOptions() + shares of stock.);
System.out.println(I am an accountant. I make + getSalary() + plus a parking allowance of + getParking());
System.out.println("I am a programmer. I make " + getSalary() + " and I" + ((getBusPass())?" get a bus pass.":" do not get a bus pass."));
o Salaries for specific types of employees are in addition to the base employee salary. Raising the base employee salary should automatically raise salaries for all types of employees (hmmm...).
o An object of the Employee class cannot be instantiated because it would be too general.
o Attribute(s) that belong to a super or subclass should be initialized in the corresponding class constructor.
Please do what is above as well as sort the Employees into natural order, salary, report, and name
Given Main**
package lab7.cscd211lab7;
import java.io.*; import java.util.*;//array list, collectio0n sort import lab7.cscd211comparators.*; import lab7.cscd211inheritance.*;
public class CSCD211Lab7 { public static void main(String [] args) { ArrayList
System.out.println("Employee List"); for(Employee e : myList) System.out.println(e);
System.out.println(); System.out.println("Employee Report"); for(Employee e : myList) //foreach loop e.report(); System.out.println(); Collections.sort(myList); //built in class and method System.out.println("Employee List: Natural Order"); for(Employee e : myList) System.out.println(e.getType() + " - " + e.getName() + " - " + e.getSalary());
System.out.println(); Collections.sort(myList, new SalaryComparator()); System.out.println("Employee List by Salary"); for(Employee e : myList) System.out.println(e.getType() + " - " + e.getName() + " - " + e.getSalary());
System.out.println(); Collections.sort(myList, new NameComparator()); System.out.println("Employee List by Name"); for(Employee e : myList) System.out.println(e.getType() + " - " + e.getName() + " - " + e.getSalary());
System.out.println();
}//end main }// end class
desired output*
Step by Step Solution
There are 3 Steps involved in it
Step: 1
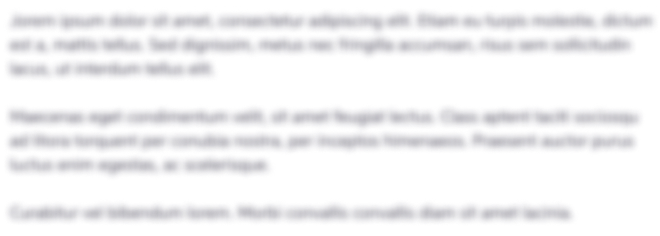
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started