Question
JAVA Programming. Your program should take 2 inputs from the user, a minimum year and a maximum year, and print out any leap years that
JAVA Programming.
Your program should take 2 inputs from the user, a minimum year and a maximum year, and print out any leap years that exist between them. Make sure that you create a new Project and Java class file for this assignment. Your file should be named Main.java.
this program should prompt the user to enter two years as input. The minimum and maximum years to be included are 1500 and 2020, like last time.
You will use a for loop to loop through each year between (and including) the years the user inputs. You'll check each year and print out a message for each leap year found.
In order to check each year, you will create a new function called checkLeapYear. This is where you'll want to check individual years. You'll want to pass in one year to that function, and check if it's a leap year. You'll print a message from checkLeapYear for each leap year found.
Instructions
- Note that the logic to check for a leap year should now be in a separate function (not the main() function).
- main() function will take the input from the user.
- Use a for loop to go through each year requested by the user, using the first input from the user as the first year, and the second input from the user as the last year to check. Pass each year you check to the checkLeapYear() function.
- The checkLeapYear() function will check a single year given to it, and print out a message if the year is in fact a leap year.
- No return value is required, but if you wish to use one you may.
- If the year checked is not a leap year, you DON'T have to print out a message for it. You are only required to print out a message for a leap year.
- Use a counter based for loop to solve the problem.
Use the following code snippet as a guide:
public static void main(String[] args) { //use Scanner to get user input for( ; ; ) { checkLeapYear(someYear); }//end loop }//end main void checkLeapYear(int someYear) { // leap year checks go here. }//end function
How to check if a year is a leap year?
There are a few things to check:
- First, if the year can be divided by 4 evenly, it is a leap year.
- HOWEVER! If the year can be divided by 100 evenly, it is NOT a leap year! (If this occurs, check if the year can divided by 400 evenly. If it is divisible by both 100 evenly, and 400 evenly, it will be considered a leap year.) You'll need to do this to handle centurial years, i.e., years ending in 00, e.g., 1900, 1800, 1700, 2000 etc.
Goals
- more experience in FOR loops
- experience using Scanner for input
- experience in Java functions
- logical thinking
Sample Runs
Sample Program Run (user input is underlined)
What years do you want to test? (make sure it's between 1500 and 2020): 1400 2020
The provided ranges are incorrect.
Sample Program Run (user input is underlined)
What years do you want to test? (make sure it's between 1500 and 2020): 2000 2021
The provided ranges are incorrect.
Sample Program Run (user input is underlined)
What years do you want to test? (make sure it's between 1500 and 2020): 2001 2003
Sample Program Run (user input is underlined)
What years do you want to test? (make sure it's between 1500 and 2020): 2000 2020
2000 is a leap year.
2004 is a leap year.
2008 is a leap year.
2012 is a leap year.
2016 is a leap year.
2020 is a leap year.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
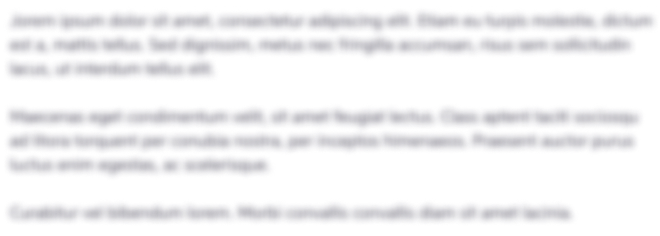
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started