Question
Java project 1. Create a Main class and put it in a package called project.circle. 2. Create a new class named Circle and store it
Java project
1. Create a Main class and put it in a package called project.circle.
2. Create a new class named Circle and store it in the project.circle package.
3. In the Circle class, add an instance variable for the radius of the circle. Provide get and set methods for this variable.
4. Add a constructor to the Circle class that doesnt take any arguments. This constructor should set the instance variable for the radius to 0.
5. Add a method named getDiameter that returns the diameter of the circle. To do that, this method can multiply the radius by 2.
6. Add code to the main method that gets the radius of the circle from the user.
7. Add code that creates a Circle object. Then, add code that uses the setRadius to set the radius in the Circle object.
8. Add code that uses the getDiameter method to get the diameter from the Circle object. Then, add code that displays the diameter.
9. Add a method named getCircumference that calculates and returns the circumference of the circle. To do that, you can multiply Pi by 2. Use 3.14159 as the value of Pi.
10. Add a method named getArea that calculates and returns the area of the circle. To do that, the code for this method can multiply Pi by the radius squared, which is the radius multiplied by the radius.
11. Add code to the main method that uses the getCircumference and getArea methods to display the circumference and area of the Circle object.
12. Modify the code so it prompts the user to enter one or more radiuses on the same line.
13. Modify the code so it parses the line of text that the user enters to get an array of String objects with one String object for each entry. To do that, you can use the split method of the String class like this: String entries[] = line.split(" ");
14. Create a loop that creates an array of Circle objects where each circle object corresponds with an entry in the array of String objects.
15. Create another loop that loops through the array of Circle objects and displays the data for each Circle object on the console. This data should include the radius. To do this, use a StringBuilder object to store the string that contains the data for the Circle objects.
16. Run the application to make sure it works correctly. This application should also work if the user enters one radius or if the user enters more than two radii. If the user enters 100 and 200, the data thats printed to the console should look something like this:
Enter one or more radiuses: 100 200
Radius: 100.0
Area: 31415.899999999998
Circumference: 628.318
Diameter: 200.0
Radius: 200.0
Area: 125663.59999999999
Circumference: 1256.636
Diameter: 400.0
Step by Step Solution
There are 3 Steps involved in it
Step: 1
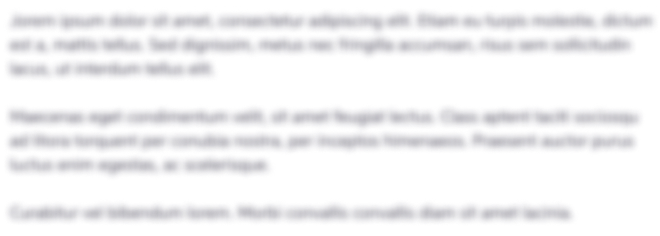
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started