Question
**** JAVA**** Project Three, Truck Loading This Programming Project allows you to demonstrate your basic knowledge of Object-Oriented Programming and the use of text files
****JAVA****
Project Three, Truck Loading
This Programming Project allows you to demonstrate your basic knowledge of Object-Oriented Programming and the use of text files for input and output. Your Java program will simulate loading trucks with various items. You will define and use two classes. The first class will be called Truck. This class will simulate the carrying capacity and contents of a truck. The second class will be called Item. The Item class will be used to simulate the items carried in each truck.
You will use information read from two text files. The first file contains the information you will need to create instances of the class named Truck. The second text file contains the information you will need to create instances of the Item class and load them into the previously created Truck objects. Your program will then use the information in the collection of Truck objects you created and updated to prepare a report written to an output file. The file will summarize the contents and value of each truck in the form of a report.
Start by designing a class called Truck. This class contains the following private fields:
- id_number, unique numeric identification number for each truck.
- plate, text description of the trucks license plate.
- contents, collection of Item objects in the truck.
- capacity, maximum carrying capacity for each truck (in pounds).
The Truck class has the following methods:
- A constructor that can be used to create an instance of the Truck class. The id_number, plate, and capacity fields must be specified as arguments. This method will define the initial value of the contents field as an empty collection, such as an ArrayList.
- An addItem method that accepts as an argument an Item object. Adding this item to the contents of the truck must not exceed the maximum weight limit of that truck. If adding an item would potentially exceed the weight limit, display a warning, and do not add the item to the trucks contents field.
- A removeItem method that accepts an sku number as a parameter and attempts to remove the associated Item object from the contents of a truck. If the truck does not contain an item with the sku number, display a warning message, and make no change to the contents.
- A getContents method that returns a reference to the contents field.
- A getIdNnumber method that returns the value of the trucks id_number.
- A getPlate method that returns the value of the trucks plate.
- A getCapacity method that returns the value of the trucks capacity.
- A getTotalWeight method that returns the total weight of all the Item objects in the contents collection.
- A getTotalValue method that returns the total dollar value of all the items contained in the truck expressed as dollars and cents.
The Item class contains the following private fields:
- sku, the stock keeping unit number for this item.
- description, the text description of the item.
- weight, the weight, in pounds, as a floating-point value (double).
- value, the value of this item in dollars and cents.
The Item class has the following methods:
- A constructor that can be used to create an instance of the Item class. All the fields in the class must be specified as arguments. This method will guard against the values of weight and value having a numeric value that is less than or equal to zero. If one or more of the weight or value numeric values is less than or equal to zero, display a warning message and set the offending field value to zero.
- A getSku method that returns the value of the stock keeping unit number.
- A getDescription method that returns the value of the items description.
- A getWeight method that returns the weight of the item in pounds.
- A getValue method that returns the value of the item in dollars and cents.
Place the class definition for the Truck class in a separate file named Truck.java. In similar fashion, place the class definition for the Item class in a separate file named Item.java.
Your Java program will read and process two input files and create a single output file in addition to limited console output. Your program will read the contents of a file named fleet.txt and use the contents of this file to initially create and define the empty Truck objects.
The fleet.txt text file contains the definitions of the Truck objects to be created. Each record in this file holds the following information in this order:
- ID number
- plate
- capacity (in pounds)
The fields in this file are comma-delimited. That is, each field is separated from the next field by a comma. Example records for this file look like this:
12345,ABC-123,7500 56789,ABX-234,7800 24680,BGT-674,80000 13579,FDU-776,6500
Consider reading each line of the file as a single string variable. Then use the split method to create an array of strings. Afterwards, strings that need to be converted to numeric values can be converted.
After creating instances of the Truck class, display a line reporting how many trucks were created. Your program will then read and process a file named loading.txt. The loading.txt text file contains transactions that will potentially change the contents of individual trucks. These are the transaction codes used for the possible truck loading and unloading activities:
- A Add an Item object to a truck (Creates an Item object).
- R Remove an Item object (identified by sku) from a truck.
There are two types of records within the loading.txt text file. To add an item to a truck, the definition of the Item object must be part of the record. Records intended to add an item to a truck contain the following comma-separated fields:
- Transaction code (A)
- Truck ID number
- Item sku number
- Item description
- Item weight (in pounds)
- Item value (in dollars and cents)
Example records intended to add an item to a truck look like this:
A,12345,1013,Toilet paper,10.5,29.97 A,12345,1008,Motor oil,40.5,45.25 A,12345,4567,Machine parts,2400,6789.45 A,56789,987,Autobile tire set,90,598.75 A,56789,8432,Pickup truck tire set,140,699.89
The second type of record that is contained in the loading.txt file is intended to remove an item from a truck. These records need only refer to the truck ID number and the items sku number. Records intended to remove an item from a truck contain the following comma-separated fields:
- Transaction code (R)
- Truck ID number
- Item sku number
Example records intended to remove an item from a truck look like this:
R,12345,1013 R,12345,1008 R,12345,4567 R,56789,7854 R,56789,987
The loading.txt file will contain both types of records. Process only records in the file that contain a transaction code of A or R. Skip records that do not contain either of these valid transaction codes. Keep track of the total number of records skipped.
Process only records in the loading.txt file that refer to trucks and items you defined earlier (using the fleet.txt file and the loading.txt file). For example, if a record in theloading.txt file refers to a truck that your program did not create, skip that record, and increment the count of skipped records. Likewise, if a record in the loading.txt file refers to an items sku number that is not contained in the specified truck, skip that record, and increment the count of skipped records. Keep track of the total number of records processed as well as the total number of records that were skipped for any reason.
After processing the entries in the loading.txt file your program can now display two items on the console (screen) using print statements. You will know and can display the total number of transaction records processed as well as the total number of records skipped.
The final step in this programming project is to calculate, display, and summarize the loading of the trucks. This information will be written to an output text file named Report.txt. The first line of your inventory report will contain your full name and a made-up name for the company such as The Albatross Shipping Company, Ltd. The second line in your inventory report will contain the phrase, Prepared on: followed by the current date and time. (This is the one and only thing I want you to look up on the Internet.) The third line will be blank. The fourth line in your report is where the heading information begins. Efficiency is a trucks total load divided by the capacity expressed as a percentage. Your loading report will look as much like this as possible:
Joe E. Bagadonutz for the Albatross Shipping Company, Ltd.
Prepared On: Monday, July 27, 2020 at 05:36:15
L O A D I N G R E P O R T
Truck License Actual
ID Number Plate Capacity Load Efficiency Value
--------- ------- -------- -------- ---------- ------------
12345 ABC-123 7,500 6,200 82.7% $4,314.00
56789 ABC-234 7,800 5,600 71.8% $2,878.89
24680 BGT-674 80,000 45,020 56.3% $21,456.87
13579 FDU-776 6,500 5,400 83.1% $1,897.43
======== ============
Total 62,220 $30,547.19
Once the loading report is complete, display the overall total weight loaded into all the trucks as well as the dollar worth of all items loaded into all trucks on the screen.
Summary of expected screen display messages:
NN Trucks have been created.
XXX Total loading records processed. YY records skipped.
Total load carried for all trucks is ZZ,ZZZ.ZZ pounds.
Total value for all items in all trucks is $DD,DDD.DD
Your program will substitute the number of Truck objects created for NN. The total number of loading records processed will be used in place of XXX. The number of records skipped for any reason will be shown in place of YY. The total weight of all items carried by all trucks will be displayed in place of ZZ,ZZZ.ZZ. The total dollar value of all items carried by all trucks will be shown in place of DD,DDD.DD.
For this assignment you will create and submit three (3) files. The first file will be named Truck.java and contain the class definition for the Truck class. The second file will be named Item.txt and contain the class definition for the Item class. The third file is the program you wrote to solve this problem. The third file will be named XYProjectThree.java, where X and Y are your first and last initials. For example, a student named Robert Wilberforce will name the file he submits RWProjectThree.java.
Your assignment will be graded by your instructor using different files than the sample data files provided. Your instructor may run their own program to evaluate how well you designed both the Truck class and the Item class. The instructors program will exercise all the required methods in both the Truck class and the Item class.
To receive full credit for this programming assignment, you must:
- Submit three separate files:
- One named XYProjectThree.java, where X and Y are your first and last initials, and
- a second file named Truck.java, which contains the class definition for the Truck class, and
- a third file named Item.java, which contains the class definition for the Item class.
- Submit a program that executes correctly.
Grading Guideline:
- Create a comment containing the students full name. (5 points)
- Correctly define all the private fields in the Truck class. (5 points)
- Correctly define the constructor method for the Truck class. (5 points)
- Correctly define all the methods in the Truck class. (5 points)
- Correctly define all the private fields in the Item class. (5 points)
- Correctly define the constructor method for the Item class. (5 points)
- Correctly define all the methods in the Item class. (5 points)
- Create Truck objects by correctly processing the fleet.txt file (10 points)
- Create Item objects and correctly add them to the appropriate trucks by processing the loading.txt file. (10 points)
- Correctly report the number of trucks created on the screen. (5 points)
- Correctly report the total number of records processed in the loading.txt file. (5 points)
- Correctly report the number of records that were skipped while processing the loading.txt file. (5 points)
- Create the Report.txt file with correct title and date and time. (10 points)
- Calculate and display the total weight and dollar value of all the items in all the trucks. (10 points)
- Calculate and include the total weight and dollar value of all the items in all the trucks in the report file. (10 points)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
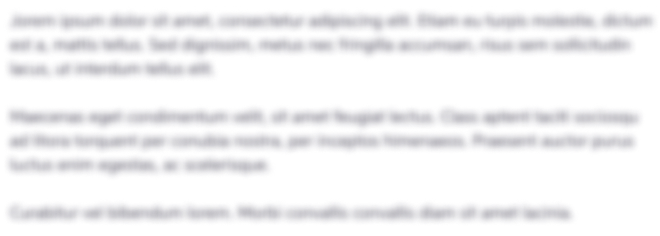
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started