Question
JAVA Purpose: This program will provide experience using decision statements, loops, and processing 2 dimensional arrays. The Angry Queens Game The goal of this game
JAVA
Purpose: This program will provide experience using decision statements, loops, and processing 2 dimensional arrays.
The Angry Queens Game
The goal of this game is to have the user place some number of angry queens onto a game board so that the angry queens cannot attack each other. The game board consists of N rows and N columns. An angry queen can attack horizontally (anything in her row), vertically (anything in her column), or diagonally from her position.
The game starts by asking how many angry queens the user wants place (N). This determines the size of the game board (NxN). Then the user is repeatedly asked where the next angry queen should be placed. The user specifies the queens position by entering a row and column number. Valid row and column numbers range from 0 up to N-1.
Based on the user specified position, there are 3 possible outcomes for placing that angry queen:
If there is an angry queen at the position specified by the user, that angry queen is removed from the game board.
If the position specified by the user is not safe, then the angry queen is not placed on the game board and the user is informed that the position specified is not safe.
If the position specified is safe, then the angry queen is placed on the game board at that position.
A safe position on the board cannot be attacked by any angry queen already placed on the game board. This means there are no angry queens in the row specified, in the column specified, or in any of the diagonals which contain the specified position.
After reaching a disposition regarding the users specified position, the current state of the game board is displayed to the user, and the process is repeated.
Once all N angry queens have been successfully placed on the game board, the game loop terminates, the user is congratulated, and asked if they wish to play again. If they wish to play on, they are once again prompted for the number of angry queens they wish to have in the new game.
To help you understand how the program should work, a sample program run is shown on the next several pages. The basic algorithm is described below.
1.Display the introduction to the game.
2.Get the number of angry queens from the user. It must be > 3. Do not proceed until a valid input has been received from the user.
3.Create a 2 dimensional array of characters which will represent the game board. Initialize this array with * characters.
Get a valid row and column number from the user as the position for the next angry queen. Do not proceed until the row and column numbers are both between 0 and N-1 inclusive.
If there is already an angry queen on the board at that position, remove it. Otherwise determine if it is safe to place an angry queen on the game board at the position specified. If it is safe, place the angry queen on the board at the position specified, otherwise let the user know that position is not safe.
Display the current game board to the user.
Repeat steps 4, 5, and 6 until all N angry queens have been successfully placed.
Congratulate the user. Determine if the user wishes to play again. If so, go to step 2.
Additional Requirements
You must use a 2 dimensional array of characters for the game board. You will also have a variable containing the total number of angry queens and a counter variable for the number of angry queens successfully placed. This is the only data you are allowed to have pertaining to the state of the game. Violation of this requirement will result in a grade of 0!
Do not use functions for this project! While they are usually desirable, the focus in this project is loops and array processing. Violation of this requirement will result in a grade of 0!
Make sure you document your program. A description of the program should be included in a Javadoc comment before the start of the class containing your program. You must also include inline comments for each significant part of your program.
The grading rubric follows on the last page of this document.
Sample program run:
Welcome to the Angry Queens Game!
The goal of this game is to place N Angry Queens on an
N x N game board so the Queens cannot attack each other.
Two Angry Queens cannot occupy the same row, column, or
diagonal on the game board.
Specify the number of Angry Queens, must be > 3
4
To remove an Angry Queen from the board,
just specify its row and column position.
Enter a row and column position for the next Angry Queen.
Must be >= 0 and < 4
0 2
* * Q *
* * * *
* * * *
* * * *
Enter a row and column position for the next Angry Queen.
Must be >= 0 and < 4
5 5
Your entry was invalid, try again.
Enter a row and column position for the next Angry Queen.
Must be >= 0 and < 4
1 0
* * Q *
Q * * *
* * * *
* * * *
Enter a row and column position for the next Angry Queen.
Must be >= 0 and < 4
1 3
It is not safe to place a queen there!
* * Q *
Q * * *
* * * *
* * * *
Enter a row and column position for the next Angry Queen.
Must be >= 0 and < 4
3 0
It is not safe to place a queen there!
* * Q *
Q * * *
* * * *
* * * *
Enter a row and column position for the next Angry Queen.
Must be >= 0 and < 4
3 2
It is not safe to place a queen there!
* * Q *
Q * * *
* * * *
* * * *
Enter a row and column position for the next Angry Queen.
Must be >= 0 and < 4
2 3
* * Q *
Q * * *
* * * Q
* * * *
Enter a row and column position for the next Angry Queen.
Must be >= 0 and < 4
3 1
* * Q *
Q * * *
* * * Q
* Q * *
Awesome! You won!
Do you want to play again? y or n:n
BUILD SUCCESSFUL (total time: 3 minutes 12 seconds)
Rubric (50 pts)
Displays an introduction to the game (3)
Gets valid input for the number of angry queens (3)
Get valid inputs for row, column position (5)
Removes angry queen from board if specified (4)
Detects when a specified row is not safe (4)
Detects when a specified column is not safe (4)
Detects when a specified position is contained in a diagonal that is not safe (7)
Only places an angry queen on board in safe position (5)
Displays the game board clearly showing any angry queens that have been placed (5)
Terminates game when all angry queens placed (3)
Only terminates program when user does not want to play again (3)
Proper coding style is followed. Comments provided for each significant step of the program. (4)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
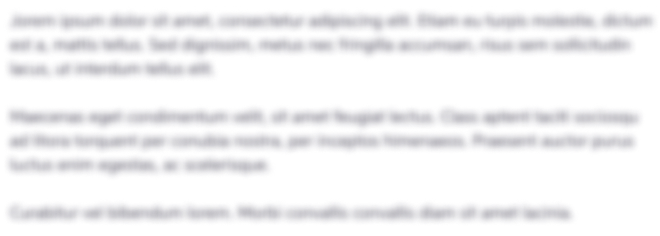
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started