Question
java question, i need to change my code but i'm having trouble so what i need is when im entering the test numbers for my
java question, i need to change my code but i'm having trouble so what i need is when im entering the test numbers for
my triangle
side 1: 3
side 2: 3
side 3: 3
it prints out this
but when i do the second test with
side1: 1
side 2: 1
side 3: 2
my problem is that if the triangle is invalid then i dont need it to print anything else after it i need to take the part where it says this is an isosceles out but i dont know how without corrupting the first test.
Here is my code:
import java.util.Scanner;
public class Triangle {
int a,b,c;
int val1,val2,val3;
private int setA;
private int setB;
private int setC;
public void Triangle(int a,int b,int c)
{
// method call isInvalid()
if (isInvalid(a,b,c)==true)
System.out.println("is not a right angle");
else
System.out.println("is not a valid triange");
// method call isInvalid()
if (isRight(a,b,c)==true)
System.out.println("is a right angle," +isRight(a,b,c));
// method call isIsosceles()
if (isIsosceles(a,b,c)==true)
System.out.println("is an isosceles triangle");
// method call isEquilateral()
if (isEquilateral(a, b, c)==true)
System.out.println("is an equilateral triangle ");
}
public int getA()
{
return setA;
}
public int getB()
{
return setB;
}
public int getC()
{
return setC;
}
public static boolean isInvalid(int a, int b, int c)
{
// triangle inequality theorem
if ((a+b >c)&&(b+c>a)&&(a+c>b))
return true;
else
return false;
}
public static boolean isRight(int a, int b, int c)
{
if(a>b&&a>c)
{
if((a*a)==(b*b)+(c*c))
return true;
else
return false;
}
else if(b>c&&b>a)
{
if((b*b)==(c*c)+(a*a))
return true;
else
return false;
}
else if(c>a&&c>b)
{
if((c*c)==(a*a)+(b*b))
return true;
else
return false;
}
else
{
return false;
}
}
public static boolean isEquilateral(int a, int b, int c)
{
if (a==b && b==c)
return true;
else
return false;
}
public static boolean isIsosceles(int a, int b, int c)
{
if(a ==b || a==c || b==c)
return true;
else
return false;
}
public static void main(String[] args) {
Triangle t=new Triangle();
// create Scanner object
Scanner input = new Scanner(System.in);
// enter value for side a
System.out.print("Enter side 1 (an integer):=> ");
t.setA=input.nextInt();
int a=t.getA();
// enter value for side b
System.out.print("Enter side 2 (an integer):=> ");
t.setB=input.nextInt();
int b=t.getB();
// enter value for side c
System.out.print("Enter side 3 (an integer):=> ");
t.setC=input.nextInt();
int c=t.getC();
System.out.printf("Triangle with sides: %d %d %d ",a,b,c);
t.Triangle(a,b,c);
}
}
----GRASP exec: java Triangle Enter side 1 (an integer) : => 3 Enter side 2 (an integer):=> 3 Enter side 3 (an integer) :=> 3 Triangle with sides: 3 3 3 is not a right angle is an isosceles triangle is an equilateral triangle ----GRASP exec: java Triangle Enter side 1 (an integer) :=> 1 Enter side 2 (an integer): => 1 Enter side 3 (an integer) :=> 2 Triangle with sides: 1 1 2 is not a valid triange is an isosceles triangleStep by Step Solution
There are 3 Steps involved in it
Step: 1
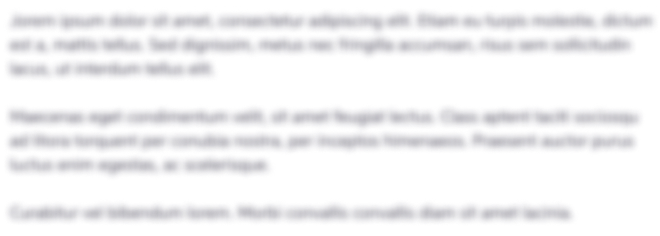
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started