Question
- Java Question - I only need help using toString and make StackArray implement StackInterface. My code is below. public interface StackInterface { public void
- Java Question - I only need help using toString and make StackArray implement StackInterface. My code is below.
public interface StackInterface
{
public void push(T newEntry);
public T peek();
public T pop();
public boolean isEmpty();
public void clear();
}
import java.util.*;
public class TheStack
{
private T stack[];
private int top;
private final static int DEFAULT_SIZE = 10;
public TheStack ()
{
this(DEFAULT_SIZE);
}
public TheStack (int initSize)
{
stack = (T[]) new Object [initSize];
top = -1;
}
public T peek()
{
if (top == -1)
return null;
return stack[top];
}
public boolean isEmpty()
{
return (top == -1);
}
public T pop()
{
if (top == -1)
return null;
T item = stack[top];
stack[top--] = null;
if(top > 0 && top == stack.length / 4)
resize (stack.length/2);
return item;
}
public void push(T item)
{
if (top == stack.length - 1)
resize(2 * stack.length);
stack[++top] = item;
}
public int size()
{
return (top + 1);
}
private void resize (int newSize)
{
T t[] = (T[]) new Object[newSize];
for (int i = 0; i
t[i] = stack[i];
stack = t;
}
@Override
public void clear() {
// TODO Auto-generated method stub
top = -1;
}
}
public class MyClass
{
public static void main(String args[]){
StackInterface
s.push(37);
s.push(42);
s.push(40);
s.push(56);
s.push(93);
s.push(70);
System.out.println("Top element is " + s.peek());
System.out.println("Popped element is " + s.pop());
System.out.println("Popped element is " + s.pop());
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
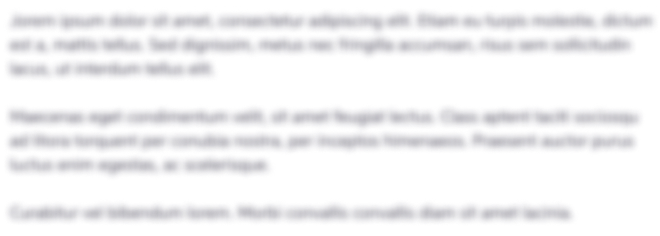
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started