Question
Java Seprate to 3 Files!!! 1. TileFloor.java (provided, but have to complete it) 2. JFrame.java (provided) 3.TileFloorViewer.java (provided) Complete the following file: 1.TileFloor.java import java.awt.Color;
Java
Seprate to 3 Files!!!
1. TileFloor.java (provided, but have to complete it)
2. JFrame.java (provided)
3.TileFloorViewer.java (provided)
Complete the following file:
1.TileFloor.java
import java.awt.Color; import java.awt.Graphics2D; import java.awt.Rectangle; import java.util.Random; /** * Describes a mosaic table top */ public class TileFloor { public static final int RED = 0; public static final int GREEN = 1; public static final int BLUE = 2; public static final int BLACK = 3; public static final int CYAN = 4; public static final int GAP = 3; public static final int SIDE = 10; public static final int DEFAULT_ROWS = 8; public static final int DEFAULT_COLUMNS = 6; private Random random; private int x; private int y; //must be initialized in the constructors private final int ROWS; private final int COLUMNS; /** * Models a tile floor with upper left hand corner at x, y and square tiles with side of 10 with the * default number of rows and columns * @param x the x coordinate of the upper left hand corner of the table top * @param y the x coordinate of the upper left hand corner of the table top */ public TileFloor(int x, int y) { random = new Random(12345); //complete this } /** * Models a tile floor with upper left hand corner at x, y and square tiles with side of 10. * @param x the x coordinate of the upper left hand corner of the table top * @param y the x coordinate of the upper left hand corner of the table top * @param rows the number of rows in this TileFloor * @param columns the number of squares in each row of this TileFloor */ public TileFloor( int x, int y, int rows, int columns) { random = new Random(12345); //complete this } /** * Sets a new x for the table top * @param x the new x coordinate of this object. */ public void setX(int x) { this.x = x; } /** * Sets a new y for the table top * @param theY the new y coordinate of this object. */ public void setY(int theY) { y = theY; } }
Use the following files:
JFrame.java
TileFloorComponent.java
import java.awt.Graphics; import java.awt.Graphics2D; import javax.swing.JComponent; public class TileFloorComponent extends JComponent { private static final long serialVersionUID = 1L; public void paintComponent(Graphics g) { // Recover Graphics2D Graphics2D g2 = (Graphics2D) g; TileFloor floor = new TileFloor(15, 20); floor.draw(g2); floor.setX(100); floor.setY(50); floor.draw(g2); floor = new TileFloor(50, 200, 10, 12); floor.draw(g2); } }
TileFloorViewer.java
import javax.swing.*; public class TileFloorViewer { public static void main(String[] args) { JFrame frame = new JFrame(); frame.setSize(300, 400); frame.setTitle("TileFloor"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); TileFloorComponent component = new TileFloorComponent(); frame.add(component); frame.setVisible(true); } }In this problem you will model a tile floor. The tile floor consists of some number of rows of (filled) colored squares. Each row contains some number of squares. Each square has a side of 10 pixels and is separated from its neighbors by 3 pixels. Each square is a random color: either red, green, blue, black, and cyan. Complete the class TileFloor. Use the constants defined for you in the starter code. You must use nested loops. Draw the rows and then the columns to match Codecheck output. For the colors, use the Color constants in the Color class. (Color.RED etc) The TileFloor will have two constructors * public TileFloor( int x, int y) where x and y are the coordinates of the upper left-hand corner of the floor. Create a Random object using the constructor that takes a seed. (Done for you) Use 8 as the default number of rows and 6 as the default the number of columns. (Use the constants defined for you in the starter) Do not draw in the constructor public TileFloor int x, int y, int rows, int columns) where xand yare the coordinates of the upper left-hand corner of the floor. rows is the number of rows and columns is the number of squares in each row. Create a Random object using the constructor that takes a seed. (Done for you). Do not draw in the constructor. The TileFloor will have these methods * public void draw (Graphics2D g2) draws the floor with the rows filled squares at the x, y coordinates. Use a nested loop * private Color randomColor ) randomly returns a Color object either red, green, blue, black, or cyan. Use the Random object you created in the constructor to determine which to return. You need to use if/ else if /else statement structure public void setX (int x) sets a new x coordinate. (Implemented for you) public void setY (int y) sets a new y coordinate. (Implemented for you) There is trick you can use to determine which color to return. Notice each color has been assigned an integer 0 to 4 in the constants. You can generate a random number between 0 (inclusive) and 5 (exclusive). If the random number is RED (O), return Color.RED. If the random number is GREEN (1), return Color.GREEN. Use the defined constants, not the numbers 0, 1 etc Provide Javadoc TileFloorComponent and TileFloorViewer are provided in this zip folder. Because the Random object is created with a seed, you will all get the same drawing every time. That is so Codecheck can check it. In this problem you will model a tile floor. The tile floor consists of some number of rows of (filled) colored squares. Each row contains some number of squares. Each square has a side of 10 pixels and is separated from its neighbors by 3 pixels. Each square is a random color: either red, green, blue, black, and cyan. Complete the class TileFloor. Use the constants defined for you in the starter code. You must use nested loops. Draw the rows and then the columns to match Codecheck output. For the colors, use the Color constants in the Color class. (Color.RED etc) The TileFloor will have two constructors * public TileFloor( int x, int y) where x and y are the coordinates of the upper left-hand corner of the floor. Create a Random object using the constructor that takes a seed. (Done for you) Use 8 as the default number of rows and 6 as the default the number of columns. (Use the constants defined for you in the starter) Do not draw in the constructor public TileFloor int x, int y, int rows, int columns) where xand yare the coordinates of the upper left-hand corner of the floor. rows is the number of rows and columns is the number of squares in each row. Create a Random object using the constructor that takes a seed. (Done for you). Do not draw in the constructor. The TileFloor will have these methods * public void draw (Graphics2D g2) draws the floor with the rows filled squares at the x, y coordinates. Use a nested loop * private Color randomColor ) randomly returns a Color object either red, green, blue, black, or cyan. Use the Random object you created in the constructor to determine which to return. You need to use if/ else if /else statement structure public void setX (int x) sets a new x coordinate. (Implemented for you) public void setY (int y) sets a new y coordinate. (Implemented for you) There is trick you can use to determine which color to return. Notice each color has been assigned an integer 0 to 4 in the constants. You can generate a random number between 0 (inclusive) and 5 (exclusive). If the random number is RED (O), return Color.RED. If the random number is GREEN (1), return Color.GREEN. Use the defined constants, not the numbers 0, 1 etc Provide Javadoc TileFloorComponent and TileFloorViewer are provided in this zip folder. Because the Random object is created with a seed, you will all get the same drawing every time. That is so Codecheck can check it
Step by Step Solution
There are 3 Steps involved in it
Step: 1
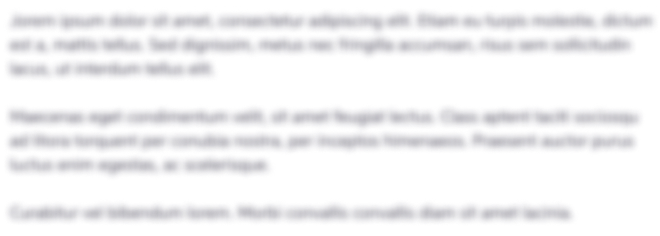
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started