Question
Java text allignment problem. Trying to change allignment via buttons for the jtaText. import java.awt.*; import javax.swing.*; import javax.swing.border.*; import java.awt.event.*; public class Unit08 extends
Java text allignment problem.
Trying to change allignment via buttons for the jtaText.
import java.awt.*; import javax.swing.*; import javax.swing.border.*; import java.awt.event.*;
public class Unit08 extends JFrame {
// Create radio buttons private JRadioButton jrbRed = new JRadioButton("Red"); private JRadioButton jrbYellow = new JRadioButton("Yellow"); private JRadioButton jrbWhite = new JRadioButton("White"); private JRadioButton jrbOrange = new JRadioButton("Orange"); private JRadioButton jrbGreen = new JRadioButton("Green");
// Create text area
// Create Buttons private JButton jbtLeft = new JButton("<="); private JButton jbtRight = new JButton("=>");
public static void main(String[] args) {
JFrame frame = new Unit08(); frame.setTitle("Unit08_Prog1"); frame.setSize(500,200); frame.setLocationRelativeTo(null); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); }
public Unit08() {
// Create a panel to hold radio buttons
JPanel jpRadioButtons = new JPanel(); jpRadioButtons.setLayout(new GridLayout(1, 5)); jpRadioButtons.add(jrbRed); jpRadioButtons.add(jrbYellow); jpRadioButtons.add(jrbWhite); jpRadioButtons.add(jrbOrange); jpRadioButtons.add(jrbGreen); add(jpRadioButtons, BorderLayout.NORTH);
// Create a radio-button group to group five buttons
ButtonGroup group = new ButtonGroup(); group.add(jrbRed); group.add(jrbYellow); group.add(jrbWhite); group.add(jrbOrange); group.add(jrbGreen);
jrbWhite.setSelected(true); //jtaText.setBackground(Color.white);
final JTextArea jtaText = new JTextArea("Programming is Fun", 5, 5); jtaText.setLineWrap(true); jtaText.setWrapStyleWord(true); jtaText.setEditable(true); JScrollPane scrollPane = new JScrollPane(jtaText); add(scrollPane, BorderLayout.CENTER);
// Create a panel to hold buttons
JPanel jpButtons = new JPanel(); jpButtons.setLayout(new GridLayout(1, 2)); jpButtons.add(jbtLeft); jpButtons.add(jbtRight); add(jpButtons, BorderLayout.SOUTH);
// Register listeners for radio buttons
jrbRed.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { jtaText.setBackground(Color.red); } });
jrbYellow.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { jtaText.setBackground(Color.yellow); } });
jrbWhite.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { jtaText.setBackground(Color.white); } });
jrbOrange.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { jtaText.setBackground(Color.orange); } });
jrbGreen.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { jtaText.setBackground(Color.green); } });
// Register listeners for buttons
jbtLeft.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { jtaText.setAlignment(Pos.BOTTOM_LEFT); } });
jbtRight.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { jtaText.setAlignment(Pos.BOTTOM_RIGHT); } });
}
}
I get this error when trying to compile:
Unit08.java:116: error: cannot find symbol jtaText.setAlignment(Pos.BOTTOM_LEFT); ^ symbol: variable Pos Unit08.java:123: error: cannot find symbol jtaText.setAlignment(Pos.BOTTOM_RIGHT); ^ symbol: variable Pos
Step by Step Solution
There are 3 Steps involved in it
Step: 1
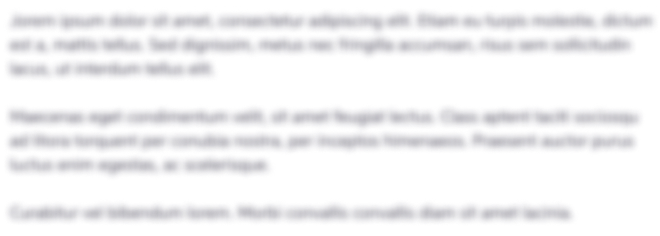
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started