Question
JAVA The goal of this assignment is to manage student data in an application using a fully encapsulated homogenous sorted singly linked list data structure.
JAVA The goal of this assignment is to manage student data in an application using a fully encapsulated homogenous sorted singly linked list data structure.
package test; import java.util.Random; import java.util.Scanner;
public class StudentApp { static SinglyLinkedList data = new SinglyLinkedList(); private static int menuChoice = 0; // need to declare static variable // private static SinglyLinkedList data;
private static void DrawMenu() { System.out.println("Enter: 1. Insert a new student's information,"); System.out.println(" 2. Fetch and output a student's information,"); System.out.println(" 3. Delete a student's information,"); System.out.println(" 4. Update a student's information,"); System.out.println(" 5. Output all the students information in sorted order"); System.out.println(" 6. Exit the program"); Scanner scan = new Scanner(System.in); try { menuChoice = Integer.parseInt(scan.nextLine()); System.out.flush(); } catch (Exception e) { System.out.flush(); System.out.println("ERROR: Choose a number between 1-6 "); menuChoice = 0; return; } if (menuChoice == 1) { Student student = new Student(); student.input(); data.insert(student); } else if (menuChoice == 2) { System.out.print("Enter a student name: "); String nameToSearch = scan.nextLine(); System.out.println(data.fetch(nameToSearch)); } else if (menuChoice == 3) { System.out.print("Enter a student name: "); String nameToSearch = scan.nextLine(); System.out.println(data.delete(nameToSearch)); } else if (menuChoice == 4) { System.out.print("Enter a student name: "); String nameToSearch = scan.nextLine(); Student studentToChange = data.fetch(nameToSearch); System.out.println("Current GPA: " + studentToChange.getGpa()); System.out.print("Update GPA: "); double newGpa = Double.parseDouble(scan.nextLine()); data.delete(nameToSearch); data.insert(new Student(studentToChange.getName(), studentToChange.getId(), newGpa)); } else if (menuChoice == 5) { data.showAll(); } else if (menuChoice == 6) { System.out.println("Thank you!"); } else { System.out.println("Error"); return; } }
public static void main(String[] args) { RandomStudents random = new RandomStudents(); Student newStudent = random.getStudent(); data.insert(newStudent);
while (menuChoice != 6) DrawMenu(); }
}
package test; import java.util.Scanner; import java.io.*;
class Student { private String name; private String id; private double gpa;
public Student() { this(" ", " ", 0.0); }
public Student(Student s) { name = s.name; id = s.id; gpa = s.gpa; }
public Student(String name, String id, double gpa) { set(name, id, gpa); }
public String toString() { return "Student: [Name: " + name + ", Id: " + id + " GPA: " + gpa + "]"; }
public int compareTo(String targetKey) { return (name.compareTo(targetKey)); }
public void input() { Scanner scanner = new Scanner(System.in); System.out.println("Enter Student name: "); name = scanner.nextLine(); System.out.println("Enter Student ID: "); id = scanner.nextLine(); System.out.println("Enter Student GPA: "); gpa = scanner.nextDouble(); scanner.nextLine(); }
public void set(String name, String id, double gpa) { this.name = name; this.id = id; this.gpa = gpa; }
public String getName() { return name; }
public String getId() { return id; }
public double getGpa() { return gpa; }
public boolean equals(Object otherObject) { if (otherObject == null) { return false; } else if (getClass() != otherObject.getClass()) { return false; } else { Student otherStudent = (Student) otherObject; return id.equals(otherStudent.id); } }
public void setName(String newName) { this.name = newName; }
public Student deepCopy() { Student clone = new Student(name, id, gpa); return clone; } }
package test;
class SinglyLinkedList {
private static Node h;
public SinglyLinkedList() {
h = null;
}
public boolean insert(Student student) {
if (student == null) {
return false;
}
Node newStudent = new Node(student);
newStudent.setRecord(student);
newStudent.setNext(null);
Node temp = null;
if (h == null) {
h = newStudent;
} else {
temp = h;
}
while (temp != null && temp.getNext() != null) {
temp = temp.getNext();
}
if (temp != null) {
temp.setNext(newStudent);
}
return true;
}
public Student fetch(String queryName) {
Node temp = h;
while (temp != null) {
if (temp.getRecord().compareTo(queryName) == 0)
return temp.getRecord();
temp = temp.getNext();
}
return null;
}
public boolean delete(String name)
{
if (h.getRecord().compareTo(name) == 0) {
h = h.getNext();
}
else {
Node curr = h, prev = null;
while (curr != null && curr.getRecord().compareTo(name) != 0)
{
prev = curr;
curr = curr.getNext();
}
if (curr == null)
return false;
prev.setNext(curr.getNext());
}
return true;
}
public boolean update(String queryName, Student updatedStudent)
{
Node temp = h;
while (temp != null && temp.getRecord().compareTo(queryName) != 0)
{
temp = temp.getNext();
}
if (temp == null)
return false;
temp.setRecord(updatedStudent);
return true;
}
public void showAll()
{
Node curr = h;
while (curr != null)
{
System.out.println(curr);
curr = curr.getNext();
}
}
}
////// RandomStudents.java
http://m.uploadedit.com/busd/1615864996367.txt
///// Node.java
http://m.uploadedit.com/busd/1615864834846.txt
The goal of this assignment is to manage student data in an application using a fully encapsulated homogenous sorted singly linked list data structure. No other types of data structure may be used for this assignment, not even another linked list variation. Each class that you write must be in its own Java source file with the exception of inner classes like the Node class presented in this chapter. Your SortedSinglyLinkedlist data structure class for this assignment will have operations that will again be in the key field mode, just as it was for Assignment 4B. You will again use the Student class that you have been using in previous assignments. Your data structure will use the Student name field as the key. No changes to the Student class should be necessary for this assignment. You may find it helpful to write a getKey method that returns the student name as a String. Your SortedSinglyLinkedlist data structure specification for this class is as follows: + SinglyLinkedlist Nodeh I/ List header SinglyLinkedlist() //Constructor boolean insert(Student) //Inserts Student object into structure Student fetch(String) 1/Fetch student object by name boolean delete(String) //Delete Student object given name boolean update(String, Student) // Updates student object void showAll) // Ordered console output of all students + The Node class mentioned above is like that in the text and here is its specification: Node Student record Node next //Points to next record in list Node) //Constructor NOTE: This class may be an inner class of SinglyLinkedList or can also be exernal. The specifications for this SinglyLinkedlist class are so very close to the textbook author's source code in Chapter 4 that you may want to download it and use that as a start. You will find it in the Chapter 4 folder on our Blackboard site. Some modifications are needed because the author uses a Listing class instead of a Student class. The big difference between this class and an unsorted version, is that you must maintain the nodes of the structure in sorted order. You cannot sort on output as many did for the Assignment 2B. The reason for this is the linked nature of the nodes requires that each node be kept in sorted order in relation to the other nodes in the structure. The search required for any of the operations (insert, fetch, delete, or update) must be a sequentional search. A binary search just will not work on this kind of structure. You may find the text discussion on pages 204 and 205 helpful in understanding how this structure works. After you have your student and Sorted SinglyLinkedList classes coded and tested, you will write a class named StudentApp that represents an application to keep track of student information. Unlike the similar task in Assignment 2B, there is no need to ask for a maximum size of the data set. You should program this though so that the user will be asked for the initial number of students. You may either prompt them to input the initial data set, using the input method of the Student class for each or use the RandomStudents class provided to you in Assignment 10 to generate the initial data set. Once this is complete, the user will be presented with the following menu options: Enter: 1 to insert a new student's information, 2 to fetch and output a student's information, 3 to delete a student's information, 4 to update a student's information, 5 to output all the student information in sorted order, and 6 to exit the program. This menu will be presented repeatedly until the user chooses option 6. Each of the options should call the methods that you wrote for your SortedSinglyLinkedList structure. You should include code that provides feedback for successful completion of the operation and an appropriate error message if an operation fails. Put your up front thinking in your Word document. Copy and paste your test runs into your Word document to include with your assignment submission. Your test program runs should show all options, not just that your code compiles. Copy and paste your test runs into your Word document to include with your assignment submission
Step by Step Solution
There are 3 Steps involved in it
Step: 1
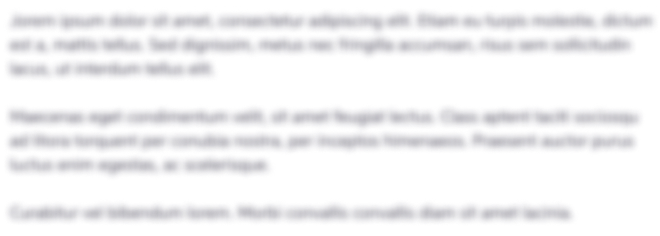
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started