Answered step by step
Verified Expert Solution
Question
1 Approved Answer
JAVA. The Main.java, linkedlist.java, NodeImpl.java, Node.java is posted. I have got pretty much all the codes for these instructions in linkedlist.java, but it is not
JAVA. The Main.java, linkedlist.java, NodeImpl.java, Node.java is posted. I have got pretty much all the codes for these instructions in linkedlist.java, but it is not letting me run for some reason.
public class Main { public static void main(String[] args) { LinkedList list = new LinkedList(); list.add(1); list.add(2); list.add(3); list.removeAtIndex(0); System.out.println(list.toString()); } }
--------------------------------------------------------------------------------------------------------------------
LinkedList.java public class LinkedList { private Node head = null; private Node tail = null; private int size = 0; /** * Remove the node at index i of the list. * Note that the first element is at index 0 * If i is larger than the size of the list, throw an IndexOutOfBounds Exception * * ex: list: 1 -> 2 -> 3 -> 4 * i: 1 * list after removeAtIndex: 1 -> 3 -> 4 * * @param i - index of node to remove */ public void removeAtIndex(int i) { if (i == 0) { Node q = head; head = q.getNext(); } else if ((i > numberOfElements - 1) || (i < 0)) System.out.println("Index out bounds."); else { Node currNode = head; for (int j = 0; j < i; j++) { currNode = currNode.getNext(); } Node temp = currNode; currNode = temp.getPrevNode(); currNode.setNext(temp.getNext()); temp = null; numberOfElements--; } } /** * Compute and return the average of all the numbers in the linked list rounded down to the nearest integer * @return an int that is the floor of the mean of the list. */ public int mean() { if (head == null) return -1; int count = 0; int sum = 0; int mean = 0; Node current = head; while (current != null) { count++; sum += current.data; current = current.next; } mean = Math.floor(sum/(double)count); return mean; } /** * Return true if this linked list is equal to the list argument, false otherwise. * Two lists are equal if they have the same size, and the same * elements in the same order. * ex: list: 1 -> 4 -> 2 * list2: 1 -> 4 -> 2 * return: true * * list: 1 -> 5 * list2: 2 -> 5 * return false; * * @return true if the lists have the same elements in the same order, false otherwise */ public boolean isEqual(LinkedList list2) { Node a = this.head, b = list2.head; while (a != null && b != null) { if (a.data != b.data) return false; a = a.next; b = b.next; } return true; } /** * Remove all the nodes at odd indices from the list. Remember that the first Node is at index 0 * * ex: list: 1 -> 3 -> 4 -> 2 -> 8 * list after removeOdds: 1 -> 4 -> 8 */ public void removeOdds() { Node current = head; Node previous = null; while ((current.getNext()) != null) { if (head == null) { System.out.println("The list is empty"); } else { while (current != null && (current.getElement()) % 2 != 1) { previous = current; current = current.getNext(); System.out.println("while condition loop entered"); System.out.println("current element " + current.getElement() + " previous element " + previous.getElement()); } if ((current.getElement()) % 2 == 1) { System.out.println("if condition met "); previous.setNext(current.getNext()); current = current.getNext(); System.out.println("current element " + current.getElement() + " previous element " + previous.getElement()); } } } } /** * Return true if the list is symmetrical, false otherwise * ex: list: 1 -> 2 -> 3 -> 2 -> 1 * return: true * * list: 1 -> 2 -> 3 -> 4 -> 5 * return: false * * @return true if the list is symmetrical, false otherwise */ public boolean isSymmetrical() { boolean isSymm = true; Stack stack = new Stack(); while (head != null) { stack.push(head.data); head = head.ptr; } while (head != null) { int i = stack.pop(); if (head.data == i) { isSymm = true; } else { isSymm = false; break; } head = head.ptr; } return isSymm; } /** * Stretch the list so that each element in the list is represented factor times * If the factor is 0 the list should be cleared (have 0 nodes) * ex: list: 1 -> 2 -> 3 * factor: 3 * list after multiply: 1 -> 1 -> 1 -> 2 -> 2 -> 2 -> 3 -> 3 -> 3 * * @param factor the amount to multiply the number of occurrences of each element by */ public void multiply(int factor) { // replace statement below with code you write Node current = head; Node nextNode = null; while(current != null){ nextNode = current.next; Node first = current; Node last = first; // creating copy for(int i=1; i 9 -> 8 -> 7 * list after reverse: 7 -> 8 -> 9 -> 10 * */ public void reverse() { Node pointer = head; Node previous = null, current = null; while (pointer != null) { current = pointer; pointer = pointer.next; } current.next = previous; previous = current; head = curret; } /** * Given a sorted linked list, remove the duplicate values from the list * ex: list: 5 -> 6 -> 7 -> 7 -> 7 -> 8 -> 8 -> 9 * list after removeRepeats: 5 -> 6 -> 7 -> 8 -> 9 * */ public void removeRepeats() { while (head != null) { Node temp = curr; while(temp!=null && temp.data==head.data) { temp = temp.next; } head.next = temp; head = head.next; } } /** * Return true if the list contains a cycle, false otherwise * ex: list: 1 -> 2 -> 3 - > 4 -- (4 points to 2) * ^ | * | | * --------------- * return: true * * list: 1 -> 2 -> 3 -> 4 * return: false * * @return true if the list contains a cycle, false otherwise */ public boolean containsCycle(Node head) { if (head == null) { return false; } Set visited = new HashSet(); visited.add(head); Node next = head.next; while( next != null) { if (visited.contains(next)) { return true; } visited.add(next); next = next.next; } return false; } /** * Merge the given linked list into the current list. The 2 lists will always be * either the same size, or the current list will be longer than list2. * The examples below show how to handle each case. * * Note: Do NOT create and return a new list, merge the second list into the first one. * * ex: list: 1 -> 2 -> 3 * list2: 4 -> 5 -> 6 * return: 1 -> 4 -> 2 -> 5 -> 3 -> 6 * * list: 1 -> 2 -> 3 -> 4 * list2: 5 -> 6 * return 1 -> 5 -> 2 -> 6 -> 3 -> 4 * * @param list2 */ public void merge(LinkedList list2) { Node list1_curr = head, list2_curr = list2.head; Node list1_next, list2_next; // While there are available positions in p; while (list1_curr != null && list2_curr != null) { // Save next pointers list1_next = list1_curr.next; list2_next = list2_curr.next; // make q_curr as next of p_curr list2_curr.next = list1_next; // change next pointer of q_curr list1_curr.next = list2_curr; // change next pointer of p_curr // update current pointers for next iteration list1_curr = list1_next; list2_curr = list2_next; } list2.head = list2_curr; } /* Implementation given to you. Do not modify below this. */ public int size() { return size; } public boolean isEmpty() { return size == 0; } public void clear() { head = null; tail = null; size = 0; } /* Returns true if the list contains a node whose value matches the element parameter, false otherwise */ public boolean contains(int element) { Node current = head; while(current != null) { if(current.getValue() == element) { return true; } current = current.getNext(); } return false; } /* converts the linked list into an array */ public int[] toArray() { int[] arr = new int[size()]; Node current = head; int i = 0; if(isEmpty()) { return arr; } while(current != null){ arr[i] = current.getValue(); current = current.getNext(); i++; } return arr; } /* adds a node to the end of the list */ public void add(int element) { Node newNode = new NodeImpl(element, null); if(isEmpty()) { head = newNode; tail = newNode; size++; } else { tail.setNext(newNode); tail = newNode; size++; } } /* removes the element from the list */ public boolean remove(int element) { Node current = head; if(isEmpty()) { return false; } if(current.getValue() == element){ head = head.getNext(); size--; return true; } while(current.getNext().getValue() != element) { current = current.getNext(); if(current == null) { return false; } } if(current.getNext().getNext() == null) { tail = current; } current.setNext(current.getNext().getNext()); size--; return true; } /* returns the value at the index parameter. */ public int get(int index) { validIndex(index); Node current = head; int i = 0; while (i < index) { current = current.getNext(); i++; } return current.getValue(); } /* sets the value of the node at index to the element */ public int set(int index, int element) { validIndex(index); Node current = head; int prevValue = 1; int i = 0; if(index == 0) { prevValue = head.getValue(); head.setValue(element); } else { while(current != null) { if(i == index) { prevValue = current.getValue(); current.setValue(element); return prevValue; } current = current.getNext(); i++; } } return prevValue; } /* adds a node at the given index with the given element as its value */ public void add(int index, int element) { if(index > size) { validIndex(index); } Node current = head; int i = 0; if(index == 0) { if(isEmpty()) { add(element); return; } else { Node newNode = new NodeImpl(element, head); head = newNode; size++; return; } } else if(index == size) { add(element); return; } while(current != null) { if(i == (index - 1)) { Node temp = current.getNext(); Node newNode = new NodeImpl(element, temp); current.setNext(newNode); size++; return; } else { current = current.getNext(); i++; } } } /* returns the index of the given element */ public int indexOf(int element) { Node current = head; int index = 0; while(current != null) { if(current.getValue() == element) { return index; } index++; current = current.getNext(); } return -1; } /* returns the last index of the element */ public int lastIndexOf(int element) { Node current = head; int index = -1; int i = 0; while(current != null) { if(current.getValue() == element) { index = i; } i++; current = current.getNext(); } return index; } public void validIndex(int i) { if(i < 0 || i >= size) { throw new IndexOutOfBoundsException("Invalid index"); } } public Node getHead() { return head; } /* prints out list */ public String toString() { String list = ""; Node current = head; while(current != null) { if(current.getNext() == null) list+= current.getValue(); else list += current.getValue() + " -> "; current = current.getNext(); } return list; } }
-----------------------------------------------------------------------------------------------------
NodeImpl.java
package a2; public class NodeImpl implements Node { private int _value; private Node _next; public Node prev = null; public Node next = null; public NodeImpl(int value, Node next) { _value = value; _next = next; } @Override public int getValue() { return _value; } @Override public void setValue(int value) { _value = value; } @Override public Node getNext() { return _next; } @Override public void setNext(Node next) { _next = next; } public Node getPrevNode() { return prev; } public void setPrevNode(Node n) { prev = n; } }
------------------------------------------------------------------------------------
package a2; public interface Node { int getValue(); void setValue(int value); Node getNext(); void setNext(Node next); default boolean hasNext() { return (getNext() != null); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
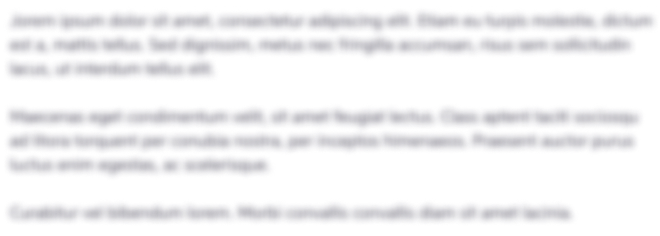
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started